Introduction
Ever had your Selenium test script abruptly halt because of an unexpected CAPTCHA? You’re not alone. CAPTCHAs those squiggly letters or image challenges exist to stop bots, but they can also break your automation flows. So, how do Selenium testers work around this problem?
Whether you’re preparing for a Selenium certification online, enrolled in an online Selenium course, or involved in test automation training, mastering CAPTCHA handling is crucial. This blog will walk you through practical methods, code examples, and industry best practices for dealing with CAPTCHAs in Selenium automation.
What Are CAPTCHAs?
CAPTCHA stands for “Completely Automated Public Turing test to tell Computers and Humans Apart.” It’s a security layer used on websites to prevent bots from abusing services like sign-ups, logins, and form submissions.
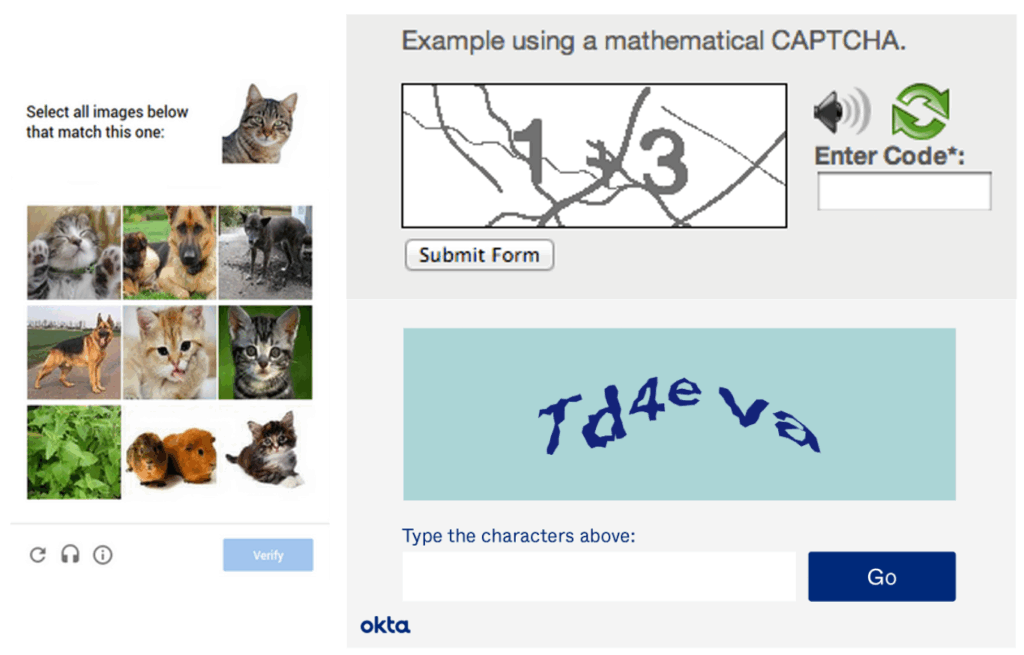
Types of CAPTCHAs You May Encounter:
- Text-based CAPTCHAs: Users enter distorted text from an image.
- Image-based CAPTCHAs: Users select images matching a prompt.
- reCAPTCHA v2: A checkbox or image challenge provided by Google.
- reCAPTCHA v3: Score-based, invisible CAPTCHA.
- hCaptcha: Similar to reCAPTCHA, increasingly popular for privacy reasons.
Why Are CAPTCHAs a Challenge in Selenium Automation?
CAPTCHAs are designed to block automated access. Selenium, being an automation tool, is often seen as a bot by these systems. This results in:
- Interrupted Tests: Scripts stop at CAPTCHA prompts.
- Reduced Automation Coverage: Test cases remain incomplete.
- Time Loss: Manual intervention delays test execution.
So, how do you keep your automation intact? Let’s explore the practical solutions.
Strategies to Handle CAPTCHAs in Selenium
Strategy 1: Disable CAPTCHA in Test Environments
If you control the application under test, the ideal approach is to disable CAPTCHAs in non-production environments.
Why This Works:
- You avoid modifying test scripts repeatedly.
- Test data is not affected.
- Full automation coverage is achievable.
Steps:
- Talk to your dev team.
- Add a feature toggle to disable CAPTCHA in dev/stage environments.
- Re-enable CAPTCHA for production only.
Strategy 2: Use Google reCAPTCHA Test Keys
For websites using reCAPTCHA, Google provides test keys that always return a positive verification. These can be added to the front-end and back-end during test configuration.
htmlBenefits:
- No CAPTCHA challenge is triggered.
- Allows continuous integration testing.
Note: Use these keys only in non-production environments.
Strategy 3: Integrate CAPTCHA Solving Services
When CAPTCHA cannot be disabled, third-party services like 2Captcha or AntiCaptcha can help. These services use real people or AI to solve CAPTCHA and return the result to your script.
Example Code (simplified for educational use):
python
import requests
# Upload the CAPTCHA image
image = open('captcha_image.png', 'rb')
response = requests.post(
'https://api.examplecaptcha.com/solve',
files={'file': image}
)
# Use the returned CAPTCHA solution
captcha_text = response.json()['solution']
input_box.send_keys(captcha_text)
Cons:
- Adds cost.
- May violate site terms of service.
Use responsibly and ethically.
Strategy 4: Use Browser Extensions
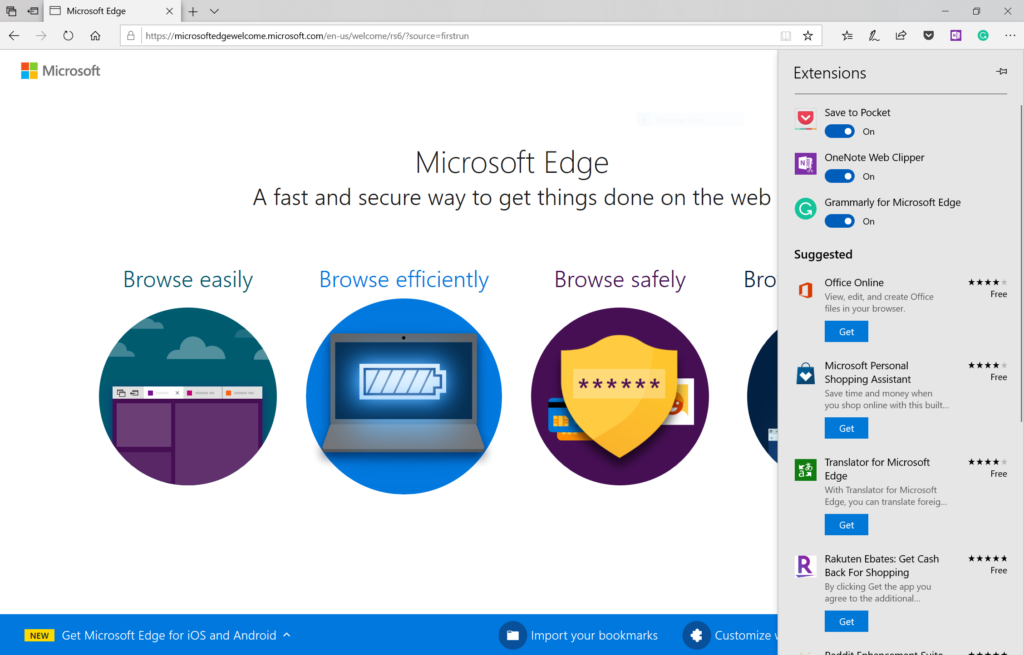
Extensions like Buster can be added to your test browser to auto-solve CAPTCHAs.
Steps:
- Download the extension
.crx
file. - Add it to your browser setup in Selenium:
python
from selenium.webdriver.chrome.options import Options
options = Options()
options.add_extension('buster.crx')
driver = webdriver.Chrome(options=options)
Note: May not work in headless mode and is not guaranteed to pass all challenges.
Strategy 5: Simulate Human Behavior
Some CAPTCHAs trigger when the system detects bot-like behavior. Making your script more human-like can reduce the number of CAPTCHA triggers.
Techniques:
- Use random waits.
- Scroll slowly through the page.
- Move the mouse before clicking.
Sample Code:
python
import random
import time
# Wait randomly between steps
time.sleep(random.uniform(1, 3))
This doesn’t bypass CAPTCHA but can reduce how often it appears.
Best Practices in CAPTCHA Handling
Handling multiple CAPTCHAs in Selenium can be a tricky affair, especially when your automation scripts run across diverse environments, user flows, or data sets. Each environment might present multiple CAPTCHAs in Selenium scenarios that behave differently, depending on traffic, browser patterns, or security thresholds. If not handled properly, these challenges can lead to broken test cases, flaky builds, and increased maintenance overhead.
To maintain test reliability, reduce manual intervention, and ensure your scripts can scale effectively, it’s essential to adopt a structured approach. By following industry-recommended best practices, you can confidently manage multiple CAPTCHAs in Selenium across development, QA, and staging pipelines. Whether you’re testing login forms, registration pages, or high-traffic workflows, having a robust strategy to address multiple CAPTCHAs in Selenium will help improve automation stability and overall test coverage.
Don’t Hardcode Solutions
Avoid embedding CAPTCHA-related logic directly into your Selenium scripts. Hardcoding solutions (like entering static CAPTCHA answers or bypassing logic using if
conditions) leads to fragile tests that break when the CAPTCHA changes. Instead, design a modular approach where CAPTCHA handling is isolated into separate reusable components. This is especially important when dealing with multiple CAPTCHAs in Selenium across different pages or environments.
Tip: Use external configuration files or environment variables to manage CAPTCHA logic dynamically.
Always Use Test Keys in QA Environments
If your application uses Google reCAPTCHA, make use of the official test keys provided by Google for staging and development. These test keys simulate a successful CAPTCHA verification without showing the actual challenge. This makes your Selenium automation stable and repeatable.
Benefit: Reduces manual effort and avoids unpredictable test failures caused by CAPTCHAs.
Avoid CAPTCHA on Login Pages in Staging
CAPTCHAs on login or authentication pages in staging can block continuous integration pipelines and automated testing. Always disable them in lower environments unless testing CAPTCHA itself is your goal. If your application displays multiple CAPTCHAs in Selenium flows, ensure they are conditionally loaded based on the environment.
Pro Tip: Use feature toggles or environment-based flags to control CAPTCHA behavior.
Log CAPTCHA Occurrences
Sometimes, CAPTCHAs appear unpredictably due to abnormal browsing behavior or traffic spikes. Make your scripts log each time a CAPTCHA is encountered. This data helps QA teams analyze the root cause and fine-tune automation flows when dealing with multiple CAPTCHAs in Selenium.
Outcome: Easier debugging, better reporting, and smarter test design.
Hands-On Code Example: CAPTCHA-Aware Automation
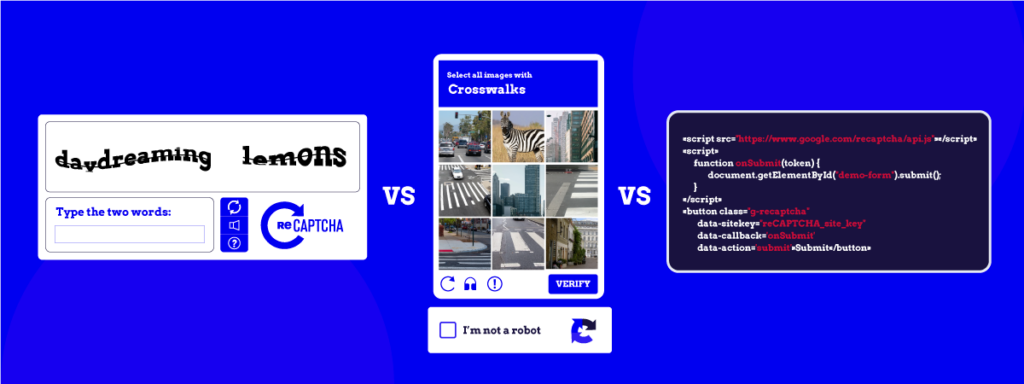
Here’s a simplified flow demonstrating how to integrate CAPTCHA-aware logic into your Selenium automation script, especially when dealing with multiple CAPTCHAs in Selenium across different workflows. In real-world scenarios, you might encounter multiple CAPTCHAs in Selenium during user registration, login, and payment flows all within a single application.
Automating these test cases requires flexible logic that can handle varying CAPTCHA challenges without breaking your test execution. This example introduces a dynamic and reusable method that prepares your script to intelligently detect and respond to multiple CAPTCHAs in Selenium, enhancing both stability and reliability in your test suite.
python
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
import random
driver = webdriver.Chrome()
driver.get("https://example.com")
# Human-like delays
time.sleep(random.uniform(1.5, 3.5))
# Enter username and password
driver.find_element(By.ID, "username").send_keys("testuser")
time.sleep(random.uniform(1, 2))
driver.find_element(By.ID, "password").send_keys("securepass")
time.sleep(random.uniform(1, 2))
# Handle CAPTCHA (mock input for demo)
try:
captcha_input = driver.find_element(By.ID, "captcha_input")
captcha_input.send_keys("manually_provided_value")
except:
print("CAPTCHA not found")
# Submit form
driver.find_element(By.ID, "submit").click()
In live environments, replace the manual CAPTCHA step with API or service logic.
Common Mistakes to Avoid
Trying to Automate CAPTCHA in Production Sites Without Permission
Attempting to bypass or automate CAPTCHAs in Selenium on live, production websites especially those you don’t own or control can result in serious consequences. This includes permanent account bans, blocked IP addresses, and potential legal issues. Always obtain proper authorization when testing in environments protected by CAPTCHA, and never use third-party CAPTCHA solvers on external production platforms without permission. Responsible use of CAPTCHAs in Selenium ensures compliance and avoids unintended security breaches.
Ignoring CAPTCHA in Test Coverage Reports
A frequent oversight in test automation is failing to report or account for CAPTCHAs in Selenium during test coverage analysis. Ignoring CAPTCHA-related scenarios leads to test gaps, missed validations, and incomplete automation. Especially when you deal with multiple CAPTCHAs in Selenium across workflows, it’s important to log when and where CAPTCHA interruptions occur. Including them in your test metrics helps you prioritize solutions and align automation goals with real-world behavior.
Running CAPTCHA Scripts in Headless Mode with Extensions
Another common mistake is attempting to handle CAPTCHAs in Selenium using browser extensions while running in headless mode. Many CAPTCHA-solving extensions, like Buster, require a visible browser window to function. Since headless mode lacks GUI support, the extension often fails silently, resulting in broken test flows. If your framework requires CAPTCHA handling via extensions, consider using a non-headless configuration or integrating alternative CAPTCHA-solving methods designed for automation.
Conclusion
CAPTCHAs are built to block automation but as a test engineer, your job is to build smart solutions. Whether you’re pursuing an online Selenium course or working toward Selenium certification online, understanding how to handle CAPTCHAs is essential for robust test automation.
Key Takeaways
- Disable CAPTCHAs in test environments when possible.
- Use test keys for reCAPTCHA challenges.
- Consider CAPTCHA-solving APIs or browser extensions if CAPTCHA can’t be bypassed.
- Simulate human behavior to avoid triggering CAPTCHA.
- Always test responsibly and ethically.
Ready to master Selenium automation and tackle real-world testing challenges like CAPTCHA handling? Enroll in H2K Infosys’ hands-on Selenium certification online course today and accelerate your test automation career!