Understanding Components
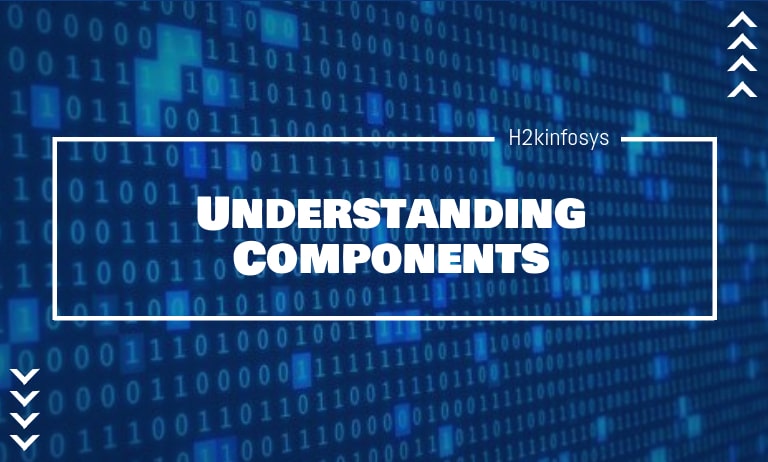
Components are one of the core building blocks of React. Every application developed in React is made up of pieces known as components. Components make the task of configuring UIs much easier. You can break a UI into multiple individual pieces called components, work on them independently, and then merge all of them in a parent component, which will become your final UI.
Components in React return a piece of JSX code that tells what should be displayed on the screen. There are two types of components in the React:
- Functional Components: Functional components are JavaScript functions and can be created by writing a JavaScript function. Those functions may or may not receive data as parameters. Given below is a valid functional component in React:
function Democomponent() { return <h1>Welcome Message!</h1>; }
- Class Components: The class components are complex than the functional components. The functional components do not know the other components in the program, whereas the class components work with each other. We can also pass data from one class component to other class components and can use JavaScript ES6 classes to create class-based components. Given below is a valid class-based component in React:
class Democomponent extends React.Component { render(){ return <h1>Welcome Message!</h1>; } }
We will use functional components only when we are sure that our component does not require interacting or working with any other component, i.e., these components do not require data from other components. But, we can compose multiple functional components under a single functional component. We can use class-based components for this purpose, but it’s not recommended, as using class-based components without need will make your application inefficient.
React is capable of rendering user-defined components. To render a React component, we can initialize an element with a user-defined component and then either pass this element as the first parameter to ReactDOM.render() or can directly pass the component as the first argument in the ReactDOM.render() method.
Syntax to initialize a component to an element:
const elementName = <ComponentName />;
Here, the ComponentName is the name of the user-defined component. The name of a component should always start with a capital letter. This is done to differentiate a component tag with HTML tags.
Below example renders a component named Welcome to the screen:
import React from 'react'; import ReactDOM from 'react-dom'; // This is a functional component function Welcome() { return <h1>Hello World!</h1> } ReactDOM.render( <Welcome />, document.getElementById("root") );
Output:
Here, we are calling the ReactDOM.render() with the first parameter.
React then calls the component Welcome, which returns <h1>Hello World!</h1>; as a result.
Then the ReactDOM efficiently updates the DOM to match with the returned element and renders that element to the DOM element with id as “root.”
Create a Class Component:
While creating a React component, the component’s name must start with an upper case letter. The component also have to include the extends React.Component statement, as it will create an inheritance to React.Component, and gives your component access to the functions of React.Component.
Step 1: Create a Class component named Car
class Car extends React.Component { render() { return <h2>I am a Car!</h2>; } }
Now, the React application has a component named Car, which returns an <h2> element.
Step 2: Display the Car component in the “root” element:
ReactDOM.render(<Car />, document.getElementById('root'));
Create a Function Component:
This is the same example as used above, but it is created using a Function component instead.
A Function component also returns HTML and it behaves pretty much the same way as a Class component.
Step 1: Create a Function component called Car
function Car() { return <h2>I am also a Car!</h2>; }
Once again, the React application has a Car component.
Step 2: Display the Car component in the “root” element:
ReactDOM.render(<Car />, document.getElementById('root'));
Component Constructor: If there is also a constructor() function in your component, this function will be called when it is initiated. The constructor function is the code where you initiate the component’s properties. In React, the component properties should be kept in an object known as state.
The constructor function is also a code where you honor the parent component’s inheritance by including the super() statement that executes the constructor function of parent component, and your component gets access to all those functions of the parent component.
Example: Create a constructor function, and add a property color:
class Car extends React.Component { constructor() { super(); this.state = {color: "red"}; } render() { return <h2>I am a Car!</h2>; } } Use the property color in the render() function: class Car extends React.Component { constructor() { super(); this.state = {color: "red"}; } render() { return <h2>I am a {this.state.color} Car!</h2>; } }
Props: Another way of handling properties of component is by using props. They are like function arguments, and you send them into the component as attributes.
Example: Use an attribute to pass color to the Car component, and use it in the render() function:
class Car extends React.Component { render() { return <h2>I am a {this.props.color} Car!</h2>; } } ReactDOM.render(<Car color="red"/>, document.getElementById('root'));
Components in Components: We can create components inside other components.
Example: Using the Car component inside the Garage component:
class Car extends React.Component { render() { return <h2>I am a Car!</h2>; } } class Garage extends React.Component { render() { return ( <div> <h1>Who lives in my Garage?</h1> <Car /> </div> ); } } ReactDOM.render(<Garage />, document.getElementById('root'));
Components in Files: React is actually re-using the code, and it can be smart to insert some of your components in separate files. For this, create a new file with a .js file extension and put the code inside it.
The file must start by importing React, and it has to end with the statement export default Car.
Step 1: This is the new file, we named it “App.js”:
import React from 'react'; import ReactDOM from 'react-dom'; class Car extends React.Component { render() { return <h2>I am a Car!</h2>; } } export default Car;
To use the component Car, you have to import the file in your application.
Step 2: Now, we will import the “App.js” file in the application, and use the Car component as if it is created here.
import React from 'react'; import ReactDOM from 'react-dom'; import Car from './App.js'; ReactDOM.render(<Car />, document.getElementById('root'));