Introduction
Understanding Stale Elements is crucial for anyone working with Selenium automation. Have you ever written a Selenium test case that suddenly breaks without changing the actual code? One of the most common reasons behind such unpredictable failures is the Stale Element Reference Exception in Selenium. This error might appear frustrating at first, but with the right understanding and approach, it becomes easy to manage.
Whether you’re just starting your online Selenium training or preparing for a Selenium certification online, understanding how and why this exception occurs is crucial for building reliable test automation frameworks. In this post, we’ll walk you through everything you need to know about Stale Element Reference Exception, how to resolve it, and how to prevent it.
What is Stale Element Reference Exception in Selenium?
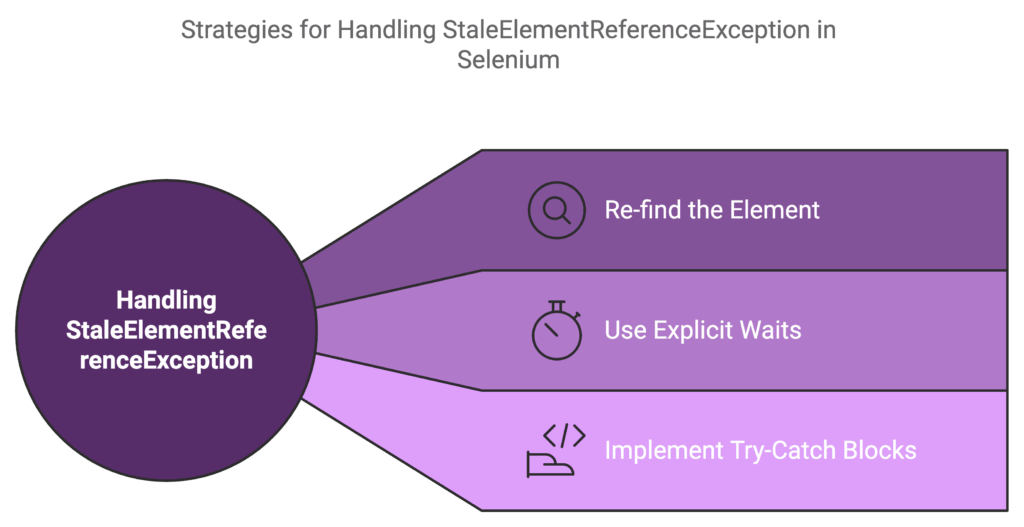
The StaleElementReferenceException in Selenium occurs when the element you’re trying to interact with is no longer attached to the DOM (Document Object Model). In simple terms, your test has a reference to an element that is outdated.
Common Error Message:
org.openqa.selenium.StaleElementReferenceException: stale element reference: element is not attached to the page document
When Does the Stale Element Reference Exception Occur?
This exception usually occurs in these scenarios:
- DOM Update: The page has been refreshed or reloaded after the element was located.
- Dynamic Content: Elements inside AJAX-driven pages or SPAs (Single Page Applications) often reload dynamically.
- Navigation Events: Clicking a link that redirects or loads another part of the page may lead to a stale reference.
Real-World Example:
Let’s say you locate a submit
button on a page and before clicking it, some dynamic content refreshes the page. Now, Selenium is still pointing to the old DOM reference.
Code Example: Stale Element Reference in Action
WebDriver driver = new ChromeDriver();
driver.get("http://example.com");
WebElement button = driver.findElement(By.id("submit-button"));
// Simulating a DOM change or refresh
Thread.sleep(5000);
driver.navigate().refresh();
// This line throws StaleElementReferenceException
button.click();
Output:
Exception in thread "main" org.openqa.selenium.StaleElementReferenceException
How to Handle Stale Element Reference Exception in Selenium
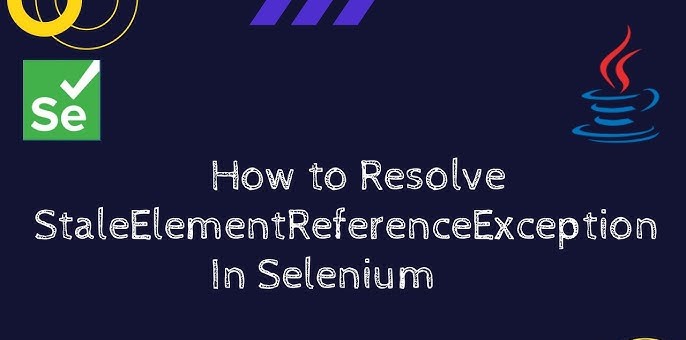
Re-Locate the WebElement
After a DOM update, re-locating the element ensures the reference is fresh.
button = driver.findElement(By.id("submit-button"));
button.click();
Use Try-Catch Block
Handle the exception with a retry logic:
int retries = 0;
while (retries < 3) {
try {
WebElement button = driver.findElement(By.id("submit-button"));
button.click();
break;
} catch (StaleElementReferenceException e) {
retries++;
}
}
Use WebDriverWait and ExpectedConditions
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement button = wait.until(ExpectedConditions.elementToBeClickable(By.id("submit-button")));
button.click();
Why Understanding This Exception in Selenium Matters
When automating web applications using Selenium, one of the most common challenges testers face is the Stale Element Reference Exception. Understanding Stale Elements Reference Exception in Selenium is essential for ensuring your automation scripts remain robust, especially when dealing with dynamic and frequently changing web pages.
The Impact of Dynamic Web Applications
Modern web applications are built to be responsive and dynamic. This means that elements on a page can be added, updated, or removed without reloading the entire page. While this is great for user experience, it presents a unique challenge for automation. Once the DOM (Document Object Model) changes, any previously located web elements become invalid. This is exactly where Understanding Stale Elements Reference Exception in Selenium becomes important.
What Is a Stale Element Reference?
A stale element is one that is no longer part of the DOM or has changed since it was located. If your script tries to interact with it (e.g., click, type, or read text), Selenium throws a StaleElementReferenceException. This exception tells you that the element you are trying to use is outdated.
Real-World Relevance of Handling This Exception
In real-world scenarios, users do not wait for elements to stabilize they interact with the application as it changes. Likewise, your test scripts should be prepared for such interactions. Understanding Stale Elements Reference Exception in Selenium empowers testers to write intelligent, failure-resistant scripts that can retry locating elements or implement waits when necessary.
By understanding Stale Elements Reference Exception in Selenium, you gain the ability to create more dependable and effective automated tests. This not only improves the success rate of your test executions but also contributes to higher software quality in agile development environments.
Industry Insight:
According to a study by Sauce Labs, over 30% of Selenium test failures occur due to synchronization issues many of which trace back to stale element exceptions.
Best Practices to Avoid Stale Element Reference
- Always locate elements after dynamic content loading.
- Use explicit waits instead of thread sleep.
- Avoid storing WebElement objects for long durations.
- Work with Page Object Model (POM) to ensure better element management.
- Regularly review and update locators for modern applications.
Practical Example with Page Object Model
public class LoginPage {
WebDriver driver;
By usernameField = By.id("username");
By passwordField = By.id("password");
By loginButton = By.id("login-btn");
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void login(String username, String password) {
driver.findElement(usernameField).sendKeys(username);
driver.findElement(passwordField).sendKeys(password);
driver.findElement(loginButton).click();
}
}
This method reduces stale references by locating elements only when needed.
Hands-On Tutorial: Fixing the Exception in a Real Test Case
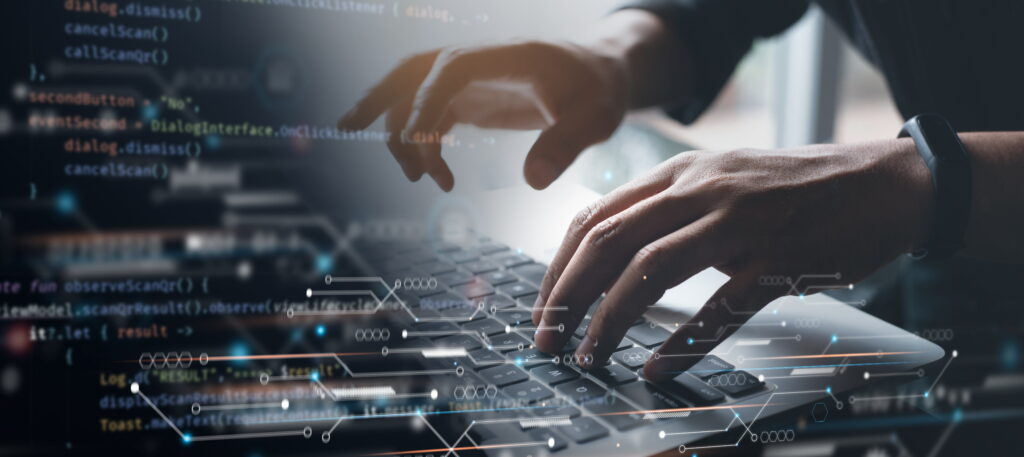
Scenario:
You have a test where you add an item to a cart and then click ‘Checkout’ but the page reloads after adding the item.
Before Fix (Unreliable):
WebElement checkout = driver.findElement(By.id("checkout"));
addItemToCart();
checkout.click(); // Might throw StaleElementReferenceException
After Fix (Reliable):
addItemToCart();
WebElement checkout = driver.findElement(By.id("checkout"));
checkout.click();
How H2K Infosys Helps You Master These Concepts
At H2K Infosys, we provide in-depth online Selenium training designed to prepare you for real-world automation testing challenges. Our Selenium course online includes hands-on exercises, live projects, and mock interview preparation to ensure you’re job-ready.
Whether you’re learning from scratch or upgrading your skills, our test automation training ensures you’re equipped to handle advanced topics like exception handling, waits, and framework development.
Course Highlights:
- Live classes with industry experts
- Hands-on labs and projects
- Selenium WebDriver, TestNG, Maven, Jenkins, and more
- Real-time scenario-based training
Key Takeaways
- The Stale Element Reference Exception in Selenium happens when the DOM changes and your test still references the old element.
- Handle it by re-locating elements or using explicit waits.
- Avoid storing element references for too long.
- Page Object Model can help manage elements better.
- Use real-time applications during training to solidify your understanding.
Conclusion
Stale Element Reference Exception in Selenium may be annoying, but it’s completely manageable once you understand the cause. With the right strategies and training, you can ensure your tests are robust and reliable.
Ready to master Selenium? Enroll in H2K Infosys’ Selenium certification online today and take your test automation skills to the next level!
Join our Selenium course online for real-time projects, expert guidance, and hands-on experience!