Introduction
Imagine running automated tests that take forever to complete or worse, fail intermittently without any clear cause. For QA engineers, speed and stability are more than just preferences they’re critical to success. As applications scale, automated test suites must keep up. That’s where Selenium performance testing comes in.
Selenium, the most widely adopted tool for browser-based automation testing, is powerful. But without optimization, even a robust Selenium script can slow down your pipeline or mislead with false negatives. This blog will dive into practical, evidence-backed Selenium performance testing tips to make your automation scripts more efficient, faster, and reliable.
Whether you’re a beginner or experienced tester, this guide will enhance your skills. If you’re pursuing a Selenium course online or considering one, these insights will give you an edge in real-world projects.
What Is Selenium Performance Testing?
Before we jump into optimization techniques, let’s clarify what we mean by Selenium performance testing.
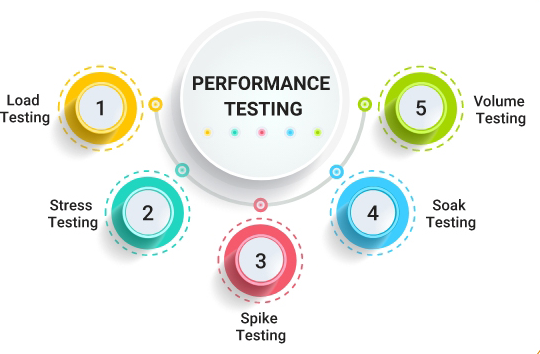
Selenium itself is not a performance testing tool like JMeter or LoadRunner. But performance testing in the Selenium context refers to improving:
- Execution speed of Selenium scripts
- Resource utilization
- Script stability
- Scalability of the test framework
Selenium Performance Testing is especially vital for continuous integration (CI) environments, where large test suites must run quickly and accurately.
Benefits of Performance Optimization in Selenium
- Reduced test execution time: Fast tests lead to quicker feedback loops.
- Improved test stability: Minimize flaky tests and random failures.
- Scalable testing: Easier parallel execution with optimized scripts.
- Resource efficiency: Lower load on CPU and memory, especially in CI/CD pipelines.
Stat Alert: According to a GitHub survey, developers spend 30% of their testing time fixing flaky or slow tests. Optimized Selenium scripts drastically reduce this overhead.
Use Explicit Waits Over Implicit Waits
What it is: Implicit waits apply a universal timeout. Explicit waits target specific elements with defined conditions.
Why it matters: Implicit waits cause unnecessary delays when elements are already available. Explicit waits are smarter and faster.
Code Example:
python from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, "username"))
)
Best Practice: Always prefer WebDriverWait
with proper expected conditions.
Reduce Unnecessary DOM Interactions
Every Selenium command triggers a call to the browser driver. More commands = more overhead.
Tips to reduce interactions:
- Cache frequently accessed elements
- Avoid redundant
.click()
or.send_keys()
calls - Minimize DOM queries inside loops
Bad Example:
python for item in items:
driver.find_element(By.ID, "submit").click() # repeated call
Optimized Version:
python submit_button = driver.find_element(By.ID, "submit")
for item in items:
submit_button.click()
Disable Animations and Transitions
Animations slow down interaction timing, causing Selenium to misfire.
Solution: Inject custom CSS or browser flags to disable them.
javascript driver.execute_script("document.body.style.transition = 'none';")
Or use Chrome flags:
python options.add_argument('--disable-gpu')
options.add_argument('--disable-extensions')
options.add_argument('--disable-animations')
Tip from H2K Infosys Selenium Course: Disable animations during test setup to ensure consistent behavior.
Run Tests in Headless Mode
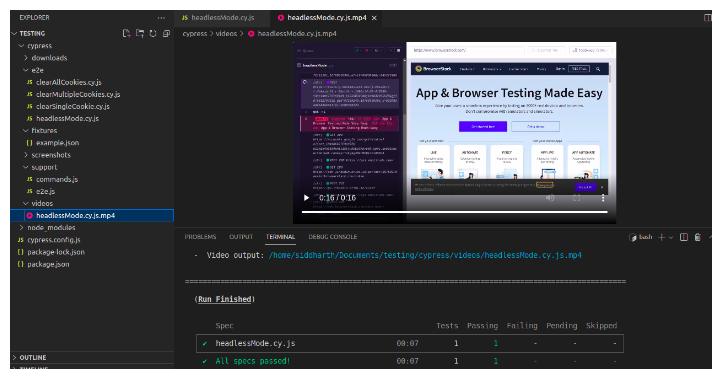
Headless mode improves test execution speed by skipping UI rendering.
python from selenium import webdriver
options = webdriver.ChromeOptions()
options.add_argument('headless')
driver = webdriver.Chrome(options=options)
Performance Boost: Headless tests can run up to 30% faster in CI/CD pipelines.
Use Parallel Execution with Selenium Grid or Tools Like TestNG
Why run one test at a time when you can run 10?
- Use Selenium Grid to distribute tests across nodes.
- In Java, use TestNG or JUnit for multithreaded execution.
- In Python, use pytest-xdist for parallelism.
TestNG Example:
xml <suite name="ParallelSuite" parallel="tests" thread-count="4">
<test name="Test1"> ... </test>
<test name="Test2"> ... </test>
</suite>
Optimize Locators
Poor locators increase test fragility and performance bottlenecks.
Best Practices:
- Prefer
ID
overXPath
- Avoid deep XPath expressions
- Use CSS Selectors where possible
Fast Locator:
python driver.find_element(By.ID, "login")
Slow Locator:
python driver.find_element(By.XPATH, "//div[2]/table[1]/tr[1]/td[3]/form/input")
Use Lightweight Browsers for CI/CD
In CI/CD, browser choice impacts speed. Consider:
- Headless Chrome or Firefox
- HtmlUnitDriver (Java-only, very lightweight)
- Using Docker containers with pre-installed drivers
Manage Browser Sessions Wisely
Avoid launching a new browser instance for every test if not needed.
Tips:
- Reuse sessions where feasible
- Use test fixtures or setup methods to handle drivers efficiently
Example:
python @pytest.fixture(scope="session")
def driver():
driver = webdriver.Chrome()
yield driver
driver.quit()
Profile and Analyze Test Runs
Use profiling tools to find bottlenecks.
- Selenium Grid Console: View execution metrics.
- Browser DevTools (Performance tab): Detect long-running operations.
- Third-Party Tools: Lighthouse, WebPageTest, or GTmetrix
Integrate with CI/CD for Continuous Feedback
Running optimized Selenium scripts in Jenkins, GitHub Actions, or GitLab CI ensures feedback is immediate and efficient.
Key CI Tips:
- Run in parallel
- Use headless mode
- Archive failure screenshots
- Send alerts for flaky tests
Real-World Example: Reducing Test Time by 60%
A US-based e-commerce firm enrolled their QA team in the Selenium training by H2K Infosys. Before optimization, their regression suite took 3 hours.
After applying best practices like parallelism, headless execution, and locator optimization, the suite ran in just over an hour.
“H2K Infosys’ hands-on Selenium course helped us transition from slow, fragile tests to a high-speed CI-ready pipeline.” QA Manager, Client Company
Bonus: Advanced Tips for Large-Scale Selenium Projects
- Use Page Object Model (POM): Clean architecture improves performance via reusability.
- Avoid Hard Waits (
time.sleep
) - Use BDD Tools like Cucumber or Behave for better test organization
- Log intelligently: Too many logs can slow down execution
- Archive test videos and screenshots smartly
Conclusion
Optimizing Selenium for performance isn’t just a good practice it’s an absolute necessity in modern software development. Fast, stable, and efficient scripts not only accelerate delivery speed but also enhance test reliability, reduce developer frustration, and ensure your automation suite scales effectively across CI/CD pipelines, large teams, and evolving project demands.
Mastering Selenium performance testing will set you apart in today’s automation-driven industry. The good news? You don’t have to learn it alone.
Ready to become a test automation expert?
Join H2K Infosys’ Selenium certification course online and gain real-world, hands-on skills to accelerate your career.
Key Takeaways
- Selenium performance testing focuses on speed, reliability, and script optimization.
- Use explicit waits, lightweight browsers, and parallel execution to improve performance.
- Apply best practices like headless mode, efficient locators, and browser session management.
- Real-world success is achievable especially with structured learning through a Selenium course.