Hibernate Configuration
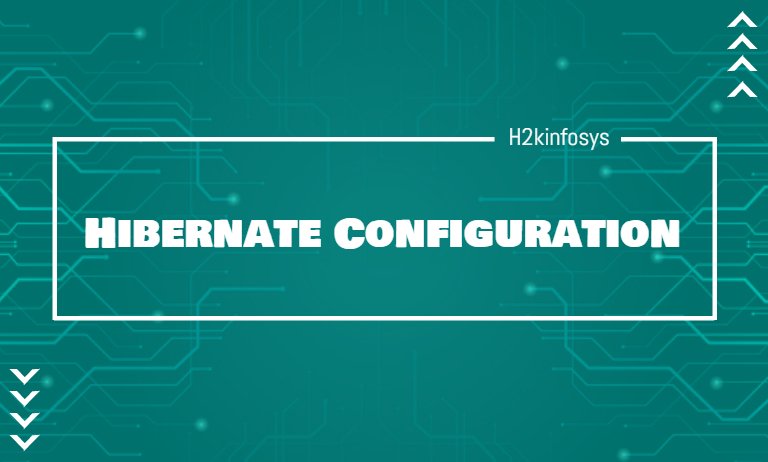
Let’s take a look at how to install and configure all environment needed for an application that works with Hibernate. Firstly, we will need to install Hibernate with other associated packages. After, we will work on the configuration. For our experiments with Hibernate examples, we will use the MySQL database. So, you need to make sure your MySQL database already have setup.
Before starting with Hibernate you need to have the installed latest version of Java on your machine. You can check if Java is installed correctly and its version by running “java -version” in cmd.
Hibernate installation
You can download the latest version of Hibernate from here: http://www.hibernate.org/downloads. Download .zip file in you use Windows OS or .tz file for Unix. We are going to use Hibernate 5.4.17.Final.
Installing Hibernate
Once your Hibernate archive is downloaded and unzipped, let’s perform the following two simple steps. Initially, you need to make sure you are your CLASSPATH variable is correct otherwise you will face problems during the compilation of your application. After, you will need to copy all the library files from /lib into your CLASSPATH.
The hibernate3.jar file has to be copied into your CLASSPATH. You can find this file in the root directory of the installation folder. It is the primary JAR that Hibernate needs to have for its work.
If you use maven in your application you need to include this dependency into your pom.xml:
<dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-agroal</artifactId> <version>5.4.17.Final</version> <type>pom</type> </dependency> In the case of Gradle: implementation 'org.hibernate:hibernate-agroal:5.4.17.Final' For Ivy: <dependency org='org.hibernate' name='hibernate-agroal' rev='5.4.17.Final'> <artifact name='hibernate-agroal' ext='pom'/> </dependency>
Hibernate Configuration
Hibernate needs to know where to find the mapping information. It defines the mapping between your Java classes and the database tables. To work with Hibernate you need a set of settings related to database and other related parameters to be configured. Developers that work on an application that includes the Hibernate technology place all such information as a standard Java properties file called hibernate.properties. Also, there is an option to supply this information as an XML file named hibernate.cfg.xml.
In our examples, we will use the way with XML formatted file hibernate.cfg.xml to specify required Hibernate properties. If some of the properties are not specified Hibernate takes the default value. Most of the properties are not required to specify them in the property file, but there are some mandatory properties. This file hibernate.cfg.xml location is in the root directory of your application’s classpath or in the resources folder.
Let’s look at the list of the important Hibernate properties. They are required to be configured for a database in a regular situation:hibernate.dialect – the property is responsible to pass information to Hibernate so it will generate the appropriate SQL for the chosen database.
hibernate.connection.driver_class – this property holds the JDBC driver class.
hibernate.connection.url – property contains the JDBC URL to the database instance.
hibernate.connection.username – property to place the database username.
hibernate.connection.password – property to place the database password.
hibernate.connection.pool_size – it is the maximum number of connections that can wait in the Hibernate database connection pool.
hibernate.connection.autocommit – property responsible for allowing the autocommit mode to be used for the JDBC connection.
In the case of use of a database along with an application server and JNDI, you will have to configure the more properties listened below:
hibernate.connection.datasource- property to define the JNDI name in the application server context, which you are using for the application.
hibernate.jndi.class – the property that holds the name of the InitialContext class for JNDI.
hibernate.jndi.<JNDIpropertyname> – property passes any JNDI property you need to the JNDI InitialContext.
hibernate.jndi.url – this property provides the URL for JNDI.
hibernate.connection.username – property to provide the database username.
hibernate.connection.password – property to provide the database password.
Hibernate with MySQL Database
Let’s create a Hibernate configuration for work with MySQL database as one of the most popular open-source database systems today. We are going to create the hibernate.cfg.xml configuration file that will be placed in the root of your application classpath.
Before starting with it, you need to create a database for our experiment. We have created the database with the name exampledb with the help of MySQL WorkBench.To create an XML configuration file you must use the Configuration DTD for Hibernate 3. You can find it here: http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd.
<?xml version = "1.0" encoding = "utf-8"?> <!DOCTYPE hibernate-configuration SYSTEM "http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name = "hibernate.dialect"> org.hibernate.dialect.MySQLDialect </property> <property name = "hibernate.connection.driver_class"> com.mysql.jdbc.Driver </property> <!-- Assume test is the database name --> <property name = "hibernate.connection.url"> jdbc:mysql://localhost/exampledb </property> <property name = "hibernate.connection.username"> root </property> <property name = "hibernate.connection.password"> root </property> <!-- List of XML mapping files --> <mapping resource = "Student.hbm.xml"/> </session-factory> </hibernate-configuration>
As you already understood, the above configuration file also includes a hibernate mapping file in the <mapping> tags.
Here is the list of various database dialect property types, in case you plan to use another database type:
- Microsoft SQL Server 2000 – org.hibernate.dialect.SQLServerDialect
- Microsoft SQL Server 2005 – org.hibernate.dialect.SQLServer2005Dialect
- SAP DB – org.hibernate.dialect.SAPDBDialect
- Sybase – org.hibernate.dialect.SybaseDialect
- Sybase Anywhere – org.hibernate.dialect.SybaseAnywhereDialect
- Microsoft SQL Server 2008 – org.hibernate.dialect.SQLServer2008Dialect
- Oracle (any version) – org.hibernate.dialect.OracleDialect
- Oracle 11g – org.hibernate.dialect.Oracle10gDialect
- Oracle 10g – org.hibernate.dialect.Oracle10gDialect
- Oracle 9i – org.hibernate.dialect.Oracle9iDialect
- PostgreSQL – org.hibernate.dialect.PostgreSQLDialect
- DB2 – org.hibernate.dialect.DB2Dialect
- HSQLDB – org.hibernate.dialect.HSQLDialect
Hibernate Mapping Files
Object-relational mappings in Hibernate are usually defined in an XML document. A lot of developers that use Hibernate technologies, choose to write the XML manually. But, there is a number of tools to generate the mapping XML document. For example, XDoclet, Middlegen,AndroMDA (for the advanced Hibernate users).
Let’s understand how to build the mapping file and look at its basic elements.
The root element of the mapping document is <hibernate-mapping>. It contains all the <class> elements.
We use the <class> elements to define the mappings of Java classes to the corresponding database tables. The Java class name is specified using the attribute name of the <class> element. The database table name is specified using the <class> element table attribute.
You can add an optional <meta> element. It is used to create a description of the class.
The <id> element in the mapping file is used to map the unique ID in class to the database table’s primary key. The id element attribute name refers to the class property. The id element attribute column refers to the column in the database table. The attribute type holds the hibernate mapping type, which will be converted from Java to SQL data type.
The element <generator> within the id element is used for auto-generation of the primary key. The class attribute of the generator element by default is set to one depending on the capabilities of the underlying database(identity, sequence, or Hilo algorithm).
The element <property> we use for mapping a Java class property to a corresponding column in the database table. The attribute name of the element <property> refers to the property in the corresponding class. The attribute column holds the column in the corresponding database table. The attribute type refers to the hibernate mapping type. These mapping types will be converted from Java to SQL data type. It’s not the complete list of the attributes and elements. There are other available.
Let’s look at the example. Here is the SQL query to create our table:
create table exampledb.STUDENT ( id INT NOT NULL auto_increment, first_name VARCHAR(20) default NULL, last_name VARCHAR(20) default NULL, email VARCHAR(20) default NULL, PRIMARY KEY (id) );
Here is our class that will be mapped to the table:
public class Student { private int id; private String firstName; private String lastName; private String email; public Student(int id, String firstName, String lastName, String email) { super(); this.id = id; this.firstName = firstName; this.lastName = lastName; this.email = email; } public Student() { } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } }
Based on the POJO class and the database table, we are going to define the mapping file Student.hbm.xml.
The mapping document has the file name with the format <classname>.hbm.xml.
<?xml version = "1.0" encoding = "utf-8"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD//EN" "http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name = "Student" table = "STUDENT"> <meta attribute = "class-description"> This class contains the student detail. </meta> <id name = "id" type = "int" column = "id"> <generator class="native"/> </id> <property name = "firstName" column = "first_name" type = "string"/> <property name = "lastName" column = "last_name" type = "string"/> <property name = "email" column = "email" type = "string"/> </class> </hibernate-mapping>
To prepare a Hibernate mapping document, you know how to map your Java data types into RDBMS data types. The types declared in the mapping files are called Hibernate mapping types. They are not Java data types and not SQL database types. For example, java.lang.Integer Java basic type is represented to ANSI SQL Type INTEGER, java.lang.Long to BIGINT, java.lang.Short to SMALLINT.
2 Comments