Login pages are one of the most common components of modern web applications. Whether you’re signing into a social media platform, a banking portal, or a cloud-based tool, you’re interacting with a login form. For testers, these pages are a critical point of failure and a prime target for automation. In this guide, we’ll break down everything you need to know about automating login pages with Selenium, from setup to execution.
Why Automate Login Pages?
Automating login pages is essential in any Selenium automation testing project. Here are some compelling reasons:
- Reusability: A login script can be reused across multiple test cases.
- Consistency: Automated tests reduce human error and ensure consistent behavior.
- Speed: Automation significantly reduces test cycle times.
- Regression Testing: Login is a common step in regression test suites.
According to a 2024 report by Capgemini, over 78% of QA teams automate their login tests as part of regression testing.
Prerequisites for Automating Login Pages
Before jumping into automating Login Pages with Selenium, it’s essential to ensure that certain prerequisites are met to create a stable and maintainable automation framework.
Understand the Application Flow
Start by gaining a thorough understanding of how the login functionality works within the application. Know the expected user behavior, page redirects, error messages, and session handling after login. This knowledge is crucial for accurately automating Login Pages with Selenium.
Identify Web Elements Clearly
Ensure that the username, password fields, login button, and error messages have unique and stable locators (IDs, names, XPaths, etc.). Unreliable locators can cause flaky test scripts and negatively affect the consistency of tests for Login Pages with Selenium.
Set Up the Testing Environment
Make sure Selenium WebDriver is configured correctly in your development environment. This includes the necessary browser drivers, framework setup (e.g., TestNG or JUnit), and any required plugins or extensions for testing Login Pages with Selenium.
Handle Security Measures
Some login pages use security measures like CAPTCHA, multi-factor authentication (MFA), or session timeouts. Understand how to bypass or simulate these conditions for successful automation of Login Pages with Selenium.
Meeting these prerequisites ensures a smoother and more effective automation process.
If you’re new to automation, we recommend enrolling in a Selenium course from H2K Infosys. Our hands-on curriculum equips you with skills to handle real-world scenarios.
Setting Up Your Selenium Environment
Here’s a quick setup guide to prepare for automating login pages with Selenium:
Install Java and Maven (or Python and pip)
# For Python users
pip install selenium
# For Java users, add Selenium as a Maven dependency
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.12.1</version>
</dependency>
Set Up WebDriver
Download the appropriate WebDriver (ChromeDriver, GeckoDriver) and set the path in your script.
from selenium import webdriver
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get('https://example.com/login')
Step-by-Step Guide: Automating Login Pages with Selenium
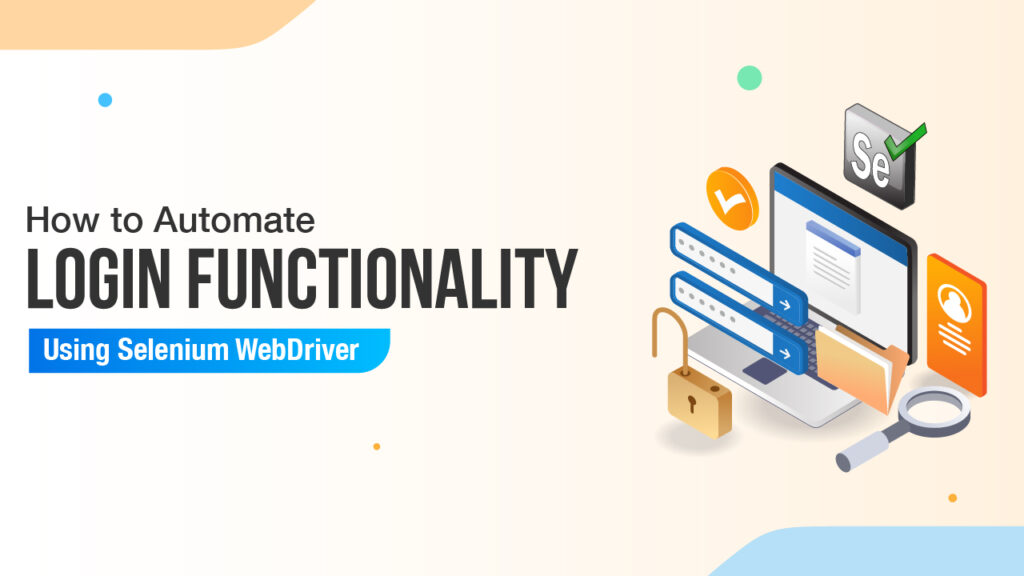
Step 1: Identify Elements on the Login Page
You need to locate the following elements:
- Username/email input field
- Password input field
- Login button
Use Selenium locators like ID, name, XPath, or CSS selector.
username = driver.find_element(By.ID, 'user')
password = driver.find_element(By.ID, 'pass')
login_button = driver.find_element(By.ID, 'login')
Step 2: Send Data to the Fields
username.send_keys('testuser')
password.send_keys('securepassword')
Step 3: Click the Login Button
login_button.click()
Step 4: Validate Successful Login
assert 'dashboard' in driver.current_url
Real-World Example: Automating Facebook Login (Demo)
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
# Set up WebDriver
driver = webdriver.Chrome()
driver.get("https://www.facebook.com")
# Locate login elements
driver.find_element(By.ID, "email").send_keys("[email protected]")
driver.find_element(By.ID, "pass").send_keys("yourpassword")
driver.find_element(By.NAME, "login").click()
Note: Facebook uses strict security, so this is only for educational purposes.
Handling Common Challenges
Dynamic Elements
Use WebDriverWait
and expected conditions.
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
WebDriverWait(driver, 10).until(EC.presence_of_element_located((By.ID, 'dashboard')))
CAPTCHA and OTP
Automating CAPTCHA or OTP is discouraged. Instead, request devs to disable them in the test environment.
Two-Step Authentication
Bypass with environment variables or mock APIs.
Using Page Object Model (POM) for Login
POM is a design pattern that improves code maintainability.
LoginPage.py
class LoginPage:
def __init__(self, driver):
self.driver = driver
self.username_field = (By.ID, 'user')
self.password_field = (By.ID, 'pass')
self.login_button = (By.ID, 'login')
def login(self, username, password):
self.driver.find_element(*self.username_field).send_keys(username)
self.driver.find_element(*self.password_field).send_keys(password)
self.driver.find_element(*self.login_button).click()
TestLogin.py
login_page = LoginPage(driver)
login_page.login('testuser', 'securepassword')
Best Practices for Automating Login Pages with Selenium
Automating Login Pages with Selenium is a crucial step in any test automation strategy, especially for web applications requiring user authentication. To ensure efficient and reliable automation, follow these best practices:
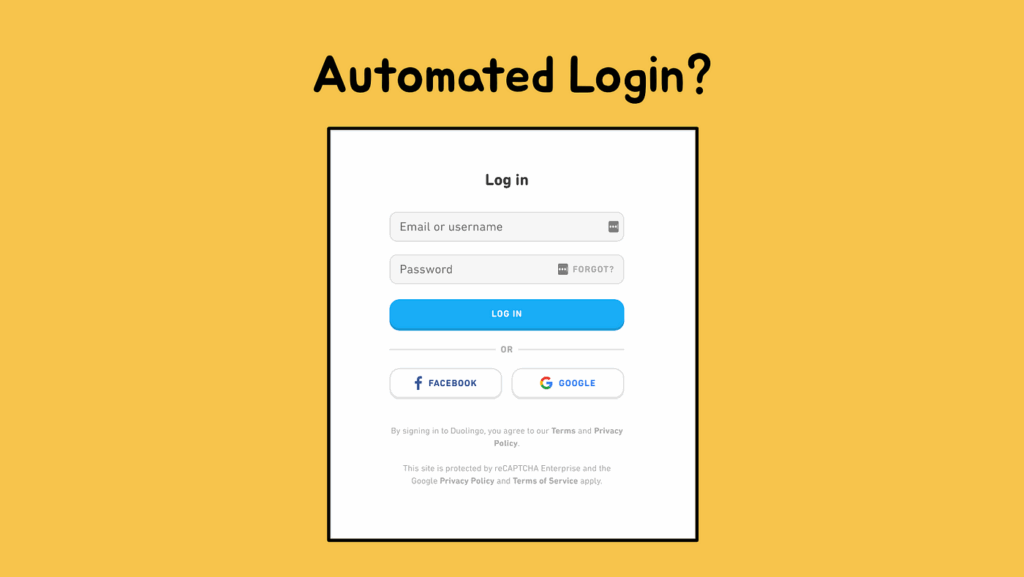
Use Data-Driven Testing
Implement data-driven testing to validate multiple login scenarios using different sets of credentials. This helps in verifying both valid and invalid login attempts on Login Pages with Selenium, improving test coverage.
Keep Login Logic Reusable
Abstract the login logic into reusable methods or utility classes. This modular approach avoids code duplication and ensures consistency across all test cases involving Login Pages with Selenium.
Incorporate Explicit Waits
Login pages often involve asynchronous behavior such as page loads or redirects. Use explicit waits to handle such dynamic elements, making your Login Pages with Selenium tests more stable and reliable.
Validate Login with Assertions
Always validate the login outcome using assertions. This confirms whether the user has successfully logged in or encountered an error, providing clear test results for Login Pages with Selenium.
Use Secure Storage for Credentials
Avoid hardcoding sensitive information. Use secure configuration files or environment variables to store login credentials safely while testing Login Pages with Selenium.
By following these practices, you can enhance the effectiveness of automating Login Pages with Selenium.
Tools to Enhance Login Page Automation
Tool | Purpose |
---|---|
TestNG/JUnit | Test execution and reporting |
Allure | Rich test reporting |
Jenkins | Continuous integration |
Docker | Cross-browser execution |
These tools are extensively covered in H2K Infosys’ Selenium certification course.
Case Study: Login Automation in a Real Project
A leading e-commerce company reduced manual testing time by 40% after automating login and checkout pages using Selenium WebDriver and Jenkins. This shift enabled faster releases and increased tester productivity.
Key Takeaways
- Login pages are a critical part of Selenium automation testing.
- Proper setup and locator strategy are essential for success.
- Real-world tools and best practices enhance test stability.
- Selenium training from H2K Infosys can help you master automation.
Conclusion
Automating login pages with Selenium is a foundational skill in automation testing. Whether you’re validating login credentials or running full regression suites, mastering this use case opens the door to broader testing success.
Ready to master Selenium automation testing? Enroll in the Selenium course at H2K Infosys and get hands-on training with real-world projects.