Selenium WebDriver enables automating drag and drop functionality, where web elements need to be moved from one location or area on the page and dropped onto a designated area or target element. Such functionality is often seen in interactive user interfaces, especially in applications involving task management, image editing, or file management.
In automation, replicating these user actions can be challenging, but with Selenium WebDriver, it becomes manageable. While simple commands work for many basic actions, drag and drop requires more advanced handling, as it involves two elements interacting with each other in a way that mimics real user actions, essential for achieving realistic test outcomes.
To perform drag-and-drop actions accurately in Selenium course or in practical test environments, testers utilize the Actions
class in Selenium. The Actions
class provides a method called dragAndDrop()
, which enables testers to chain commands, performing drag-and-drop actions smoothly.
This functionality goes beyond basic WebElement commands, allowing testers to create complex user interactions such as hovering, double-clicking, or clicking and holding, ensuring all aspects of the user experience are accurately simulated. By using the Actions class in Selenium WebDriver, testers can build reliable automated tests that closely mirror real-world scenarios, enhancing the comprehensiveness of test coverage and creating more interactive, responsive test scripts.
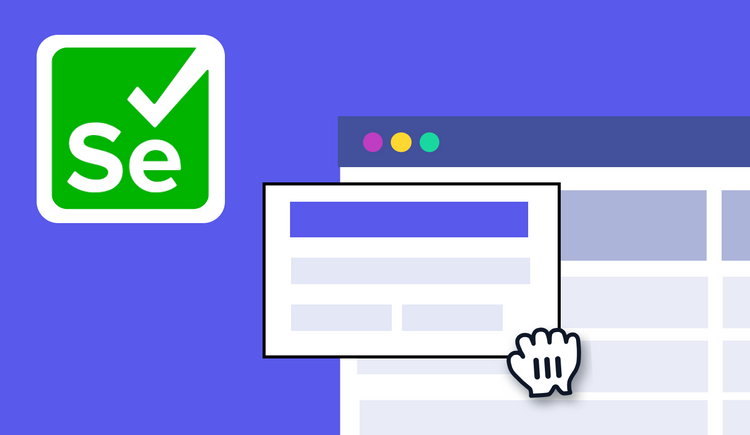
What is the Actions class in Selenium?
Actions class in Selenium WebDriver is a collection of individual actions that you want to perform. E.g., we might want to perform a mouse click on an element. In this case, we can look at two different actions:
- Moving the mouse pointer to the element
- Clicking on the element
There is a collection of methods available in the Actions class. The below screenshot shows the list of methods available.
Actions class & Action class reside in org.openqa.selenium.Interactions
package of Selenium WebDriver API. To consume these, import their packages.
import org.openqa.selenium.interactions.Actions; import org.openqa.selenium.interactions.Action; Action class is defined and called using the given syntax: Actions action = new Actions(driver); action.moveToElement(element).click().perform();
Methods of Action Class:
The Action class is mainly used for handling advanced mouse and keyboard actions, which are crucial for creating interactive and realistic tests in Selenium WebDriver. With this class, testers can perform various user interactions like single and double-clicking, right-clicking, dragging and dropping, and keyboard key presses. Selenium WebDriver offers specific methods within the Action class, such as clickAndHold()
, moveToElement()
, release()
, and sendKeys()
, to enable testers to build scripts that mimic real user actions.
This functionality allows for a more comprehensive and effective testing process by ensuring that automated tests closely reflect real-world usage scenarios. Selenium provides various methods.
- Mouse Actions:
- doubleClick(): it performs double click on the element
- clickAndHold(): it performs long click on the mouse without releasing it
- dragAndDrop(): it drags the element from one point and drops to another
- moveToElement(): it shifts the mouse pointer to the center of the element
- contextClick(): it performs right-click on the mouse
- Keyboard Actions:
- sendKeys(): it sends a series of keys to the element
- keyUp(): it performs key release
- keyDown(): it performs keypress without release
What is Drag and Drop in Selenium Web driver?
Drag and Drop action is performed using a mouse when a user moves/drags any web element from one location/area and then places/drops it to another point.
It is a common action used in Windows Explorer while moving a file from one folder to another. Here, the user selects a file from the folder, drags it to the desired folder, and drops it.
The different methods to perform Drag and Drop are:
- Drag and Drop using the Action Class dragAndDrop Method.
- Drag and Drop using the clickAndHold, moveToElement, and release Method.
- Drag and Drop using the dragAndDropBy and offset method.
Drag and Drop using the Action Class dragAndDrop Method:
In the Selenium dragAndDrop method, two parameters are passed:
- The first parameter is the Sourcelocator, which is being dragged.
- The second parameter is the Destinationlocator on which the Sourcelocator needs to be dropped.
Syntax:
Actions action = new Actions(driver); action.dragAndDrop(Sourcelocator, Destinationlocator).build().perform(); Example of Drag and Drop using Action Class dragAndDrop method: import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.interactions.Actions; public class DemoDragDrop { public static void main(String arg[]) throws Exception { // Initiate browser WebDriver driver=new FirefoxDriver(); // maximize browser driver.manage().window().maximize(); // Open webpage driver.get("http://jqueryui.com/resources/demos/droppable/default.html"); // Add 5 seconds wait Thread.sleep(5000); // Create object of actions class Actions act=new Actions(driver); // find element which we need to drag WebElement drag=driver.findElement(By.xpath(".//*[@id='draggable']")); // find element which we need to drop WebElement drop=driver.findElement(By.xpath(".//*[@id='droppable']")); // this will drag element to destination act.dragAndDrop(drag, drop).build().perform(); } }
Drag and Drop using the clickAndHold, moveToElement, and release Method:
Methods for performing drag and drop on a web element are as follows:
- clickAndHold(WebElement element) – It clicks a web element at the middle (without releasing).
- moveToElement(WebElement element) – It moves the mouse pointer to the middle of the web element without clicking.
- release(WebElement element) – It releases the left click (which is in the pressed state).
- build() – Generates a composite action.
Example of Drag and Drop using clickAndHold, moveToElement and release Method:
import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.interactions.Actions; public class DragAndDrop { public static void main(String arg[]) { System.setProperty("webdriver.gecko.driver","E:\\geckodriver.exe"); WebDriver driver = new FirefoxDriver(); driver.get("https://www.stqatools.com"); WebElement drag1 = driver.findElement(By.id("Drag_id")); WebElement drop1 = driver.findElement(By.id("Drop_id")); Actions act = new Actions(driver); act.clickAndHold(drag1).build().perform(); act.moveToElement(drop1).build().perform(); act.release(drop1).build().perform(); } }
Drag and Drop using the dragAndDropBy and offset method:
This method will click & hold the source element and moves by a given offset, then releases the mouse. Offsets are defined by x & y.
- xOffset is horizontal movement
- yOffset is a vertical movement
Syntax:
Actions action = new Actions(driver);
actions.dragAndDropBy(source, xOffset, yOffset).perform();
Example:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
public class HandleDropDrop {
public static void main(String arg[]) throws InterruptedException {
System.setProperty("webdriver.chrome.driver", "./src/test/resources/chromedriver.exe");
WebDriver driver=new ChromeDriver();
// Open webpage
driver.get("http://jqueryui.com/resources/demos/droppable/default.html");
driver.switchTo().frame(0);
// Add 5 seconds wait
Thread.sleep(5000);
// Create object of actions class
Actions act=new Actions(driver);
// find element which we need to drag
WebElement drag=driver.findElement(By.xpath(".//*[@id='draggable']"));
// calling the method and x,y cordinates are random
act.dragAndDropBy(drag, 250, 150).build().perform();
}
}
Conclusion
In conclusion, implementing drag-and-drop functionality with Selenium WebDriver provides a powerful way to automate complex user interactions, making it ideal for testing dynamic and interactive applications.
Using the Actions
class, testers can simulate real-world scenarios where elements are moved across the screen, allowing for a deeper level of testing on user interfaces that rely on drag-and-drop interactions. This capability ensures that applications respond correctly to user actions, enhancing the reliability of automated test cases for scenarios that require precision and seamless interactions.
By mastering drag-and-drop with Selenium WebDriver, testers gain the ability to automate detailed user flows that go beyond simple clicks and inputs. This functionality not only saves time and effort in testing but also increases test accuracy by replicating the nuanced actions of end users.
As a result, applications can be validated more comprehensively, leading to a smoother, error-free user experience. Leveraging drag-and-drop automation helps teams identify potential issues early, ensuring that the final product meets user expectations and performs optimally under various interaction scenarios.
Call to Action
Ready to elevate your skills in Drag and Drop with Selenium WebDriver? At H2K Infosys, we offer comprehensive, hands-on training designed to help you automate complex interactions, including the powerful drag and drop functionality.
This course will teach you how to effectively use the Actions
class to simulate real-world user actions, making your automated tests more accurate and responsive.By mastering these techniques in a Selenium certification course, you’ll be equipped to handle dynamic user interfaces and validate applications that require high levels of interactivity.
With expert-led guidance, our training at H2K Infosys goes beyond the basics, giving you in-depth experience in creating robust, interactive test cases that reflect real user behavior.
Our practical, project-based approach ensures you gain the confidence and skills needed to handle advanced automation challenges. Don’t miss out enroll today and learn to create seamless, efficient test scripts that stand out in the field of automation testing!