Garbage Collection in C# (.NET Framework)
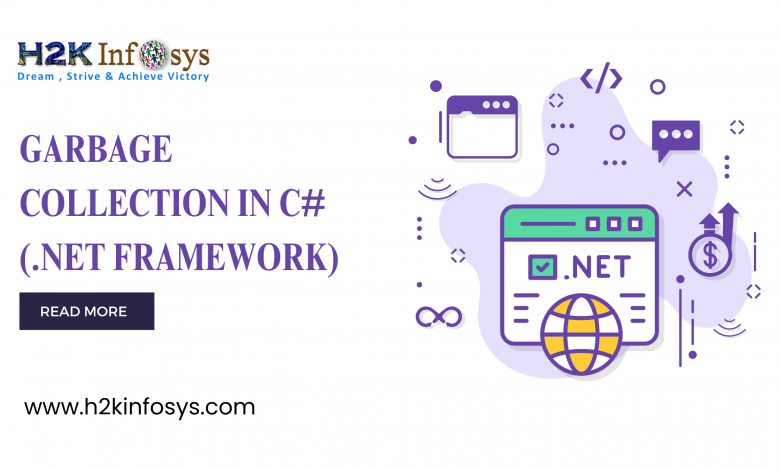
The .NET Framework and many other programming languages employ garbage collection as a memory management mechanism. Memory management and the automatic release of memory that the program is no longer using are the responsibilities of the garbage collector in C#.
In order to identify which objects are still in use and which are no longer required, the garbage collector periodically scans the memory of the program. The garbage collector automatically frees up memory for objects that are no longer in use after they are tagged for collection. To learn more, check out the online .NET course.
The following are some of the main attributes of the garbage collector in C#:
- Automatic memory management: Developers don’t have to bother about manually allocating or freeing up memory thanks to the garbage collector. By automatically managing memory, the garbage collector helps lower the possibility of memory leaks and other problems.
- Minimal effect on application performance: The trash collector normally has little effect on application performance because it operates in the background. Garbage collection, however, occasionally results in momentary application delays or slowdowns, especially when a significant quantity of memory needs to be released all at once.
- Generation-based collection: Memory management in C# is handled by the garbage collector using a generation-based methodology. Items are first assigned to a “young” generation; if they make it through several garbage collection cycles, they are then transferred to an “old” generation. Since the majority of objects are collected from the younger generation, this method helps shorten the time needed for garbage collection.
- Finalisation: The procedure that enables objects to carry out cleanup operations prior to their destruction is called finalisation, and the garbage collector also offers support for it. After all other objects have been collected, the garbage collector processes the finalisation queue that contains the objects with finalizers.
All things considered, the garbage collector is a strong and practical .NET Framework feature that may make memory management in C# programs are easier. Even while it could occasionally result in performance problems, they are usually avoidable with proper planning and memory consumption optimization of the application.
Garbage Collection in the .NET Framework allows for automatic memory management. A specific amount of heap memory is set aside for a class object when it is created at runtime. However, the memory space allotted to the object is wasted because it cannot be used once all of the program’s tasks pertaining to it have been finished. Garbage collection .NET Framework comes in quite handy in this situation since it automatically frees up memory when it is no longer needed.
Trash collection is always done on a managed heap, which is powered by an engine called the optimization engine. Garbage Collection.NET Framework takes place when at least one of several requirements is met. The following are the requirements listed:
- Garbage collection is required if the system has little physical memory.
- Garbage collection takes place when the amount of memory allocated to different items in the heap memory is above a predetermined threshold.
- Garbage collection takes place if the GC.Collect method is called. However, since garbage collectors typically operate automatically, this method is rarely invoked in exceptional circumstances.
Phases in Garbage Collection
The three primary stages of garbage collection are as follows. Here are some details about them:
- Marking Phase: During the marking phase, a list of every live object is made. To accomplish this, track down all of the root objects’ references. The heap memory may be cleared of any objects that are not included in the list of live objects.
- Relocating Phase: During this phase, all of the items that were listed on the list of active objects have their references modified to reflect the new location to which they would be moved during the compacting phase.
- Compacting Phase: During the compacting phase, the live objects are relocated and the space held by the dead objects is liberated, resulting in the compacting of the heap. Following garbage collection, every live item that is still there is shifted in the original order to the older end of the heap memory.
Heap Generations in Garbage Collection in .NET Framework
To ensure that various objects with varying lifetimes may be treated effectively during garbage collection .NET Framework, the heap memory is divided into three generations. Depending on the size of the project, the Common Language Runtime (CLR) will allocate RAM to each Generation. The Collection Means Method will be called internally by the Optimization Engine to determine which items belong in Generation 1 or Generation 2.
- Generation 0: The heap memory’s generation 0 contains all of the transient items, like temporary variables. Unless they are huge objects, all newly allocated objects are implicitly generation 0 objects. In general, generation 0 has the highest frequency of waste collection.
- Generation 1: Space occupied by generation 0 objects that are transferred to generation 1 if they are not released during a garbage collection cycle. This generation’s objects act as a kind of buffer between Generation 0’s short-lived items and Generation 2’s long-lived objects.
- Generation 2: Objects that are in Generation 1 and are not released in the subsequent garbage collection cycle are transferred to Generation 2. Since they are static objects and stay in heap memory for the length of the process, generation 2 objects are long-lived.
Note: A generation’s garbage collection entails the collecting of all subsequent generations’ garbage. This indicates that every item in that specific generation as well as any subsequent generations is freed. This is the reason why generation 2 garbage collection is referred to as a full garbage collection as every object in the heap memory has been released. Additionally, the memory allocated to Generation 2 will surpass that of Generation 1, and in a similar vein, Generation 1’s memory will surpass that of Generation 0’s (Generation 2 > Generation 1 > Generation 0).
Benefits of Garbage Collection
- Garbage Collection uses generations of garbage collection .NET Framework to successfully allocate things on the heap memory in an efficient manner.
- Garbage collection automatically releases memory space when it is no longer needed, thus manual memory freeing is not necessary.
- To ensure that no item inadvertently utilises the contents of another object, garbage collection manages memory allocation securely.
- Garbage collection clears the memory of objects that were previously released, so constructors of newly formed objects do not need to initialise every field.
Conclusion
Check out the online .NET training to learn more about Garbage Collection.