Introduction
In the competitive field of automation testing, mastering Selenium WebDriver is a critical skill for every software tester. One of the most sought-after capabilities of Selenium is its ability to interact seamlessly with Excel files. This integration enables testers to efficiently manage test data, perform data-driven testing, and log test results, making it a cornerstone of robust automation frameworks.
Excel files are widely used for storing test scenarios, inputs, and expected outputs in a structured format. By leveraging Selenium with Apache POI, testers can streamline their workflows and handle dynamic testing requirements with ease. This blog provides an in-depth guide to reading and writing Excel files in Selenium WebDriver, complete with code examples, practical applications, and troubleshooting tips to ensure success.
In the rapidly evolving field of Selenium testing, mastering Selenium WebDriver through a comprehensive Selenium course is a must for aspiring testers. Selenium not only allows you to automate web applications but also empowers you to handle real-world challenges like reading and writing data from Excel files. Whether you’re a beginner or a seasoned professional, understanding how to work with Excel in Selenium will significantly enhance your automation capabilities.
Why is Excel Integration Crucial in Automation Testing?
Excel files are widely used in the software testing lifecycle for storing test data, configurations, and results. Automation testers often need to:
- Retrieve test data stored in Excel files.
- Log execution results back into Excel.
- Utilize data-driven testing techniques for validating multiple scenarios efficiently.
By learning how to integrate Excel with Selenium WebDriver, you can boost the flexibility and robustness of your test scripts.
Prerequisites for Using Excel with Selenium
To read and write data from Excel files using Selenium WebDriver, you’ll need:
- Java Development Kit (JDK) installed.
- Apache POI Library for Excel operations.
- Eclipse IDE or any Java-compatible IDE.
- Basic knowledge of Selenium WebDriver and Java programming.
Let’s dive into a step-by-step tutorial that simplifies Excel file handling in Selenium.
Setting Up the Environment
Step 1: Add Apache POI Library to Your Project
Apache POI is a powerful library in Java designed for handling Microsoft Office files, including Excel. Follow these steps to integrate it into your project:
- Download Apache POI: Visit the Apache POI Official Website and download the library.
- Add JAR Files to Your Project:
- Extract the downloaded Apache POI package.
- Add the required JAR files (poi, poi-ooxml, poi-ooxml-schemas, and commons-collections4) to your project build path in Eclipse.
- Verify the Setup: Ensure your project recognizes the Apache POI library.
Step 2: Prepare an Excel File
Create a sample Excel file (e.g., TestData.xlsx
) with test data. For example:
TestCaseID | InputValue | ExpectedResult |
---|---|---|
TC001 | 10 | Pass |
TC002 | 20 | Pass |
Step 3: Configure Selenium WebDriver
Ensure your Selenium WebDriver setup is complete and working before proceeding.
Reading Data from Excel File
Reading data from an Excel file is a critical aspect of automation testing, particularly for data-driven frameworks. This process involves a series of steps to ensure that the required data is accessed efficiently and accurately. First, the file needs to be opened using appropriate libraries such as Apache POI for Java, OpenPyXL for Python, or similar tools depending on the programming language in use.
Once the file is open, the next step is navigating to the specific sheet that contains the data relevant to the test. This is typically done by identifying the sheet by its name or index. After locating the desired sheet, testers proceed to fetch the required data by specifying the cell, row, or column coordinates. Advanced implementations may include looping through multiple rows and columns to extract large datasets or applying filters to retrieve specific values.
Proper exception handling and validations are essential during this process to account for scenarios such as missing files, invalid sheet names, or empty cells, ensuring the robustness of the test script.
This systematic approach makes reading data from Excel files an indispensable part of creating reliable and reusable automation scripts.
Code Implementation
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.File;
import java.io.FileInputStream;
public class ReadExcelData {
public static void main(String[] args) {
try {
// Specify the path of the Excel file
File file = new File("TestData.xlsx");
// Load the file
FileInputStream fis = new FileInputStream(file);
// Load the workbook
Workbook workbook = new XSSFWorkbook(fis);
// Load the desired sheet
Sheet sheet = workbook.getSheet("Sheet1");
// Iterate through rows and columns
for (Row row : sheet) {
for (Cell cell : row) {
switch (cell.getCellType()) {
case STRING:
System.out.print(cell.getStringCellValue() + "\t");
break;
case NUMERIC:
System.out.print(cell.getNumericCellValue() + "\t");
break;
default:
System.out.print("\t");
}
}
System.out.println();
}
workbook.close();
fis.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Explanation
- File Input Stream: Loads the Excel file into the program.
- Workbook and Sheet: Represents the Excel file and its sheets.
- Iteration: Loops through rows and cells to fetch data.
Writing Data to Excel File
Writing data in automation testing is an essential task that involves either updating an existing file with new information or creating a completely new file to store the data. This process is particularly valuable in scenarios like logging test results, maintaining execution records, or saving dynamically generated test data. When updating an existing file, it typically involves appending new data to preserve previous records or overwriting specific cells with updated values.
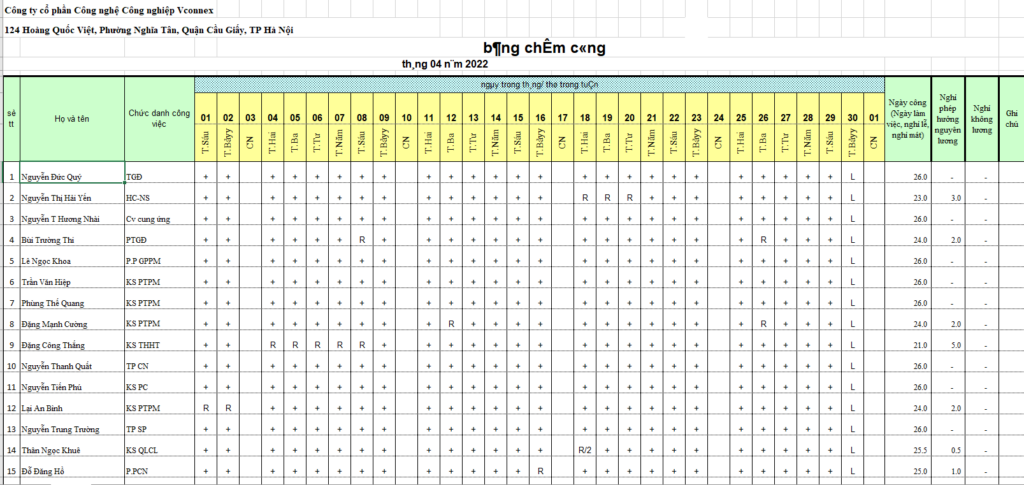
On the other hand, creating a new file is useful for generating fresh reports or storing data for isolated test runs. Modern testing frameworks, like Selenium, often integrate libraries such as Apache POI or OpenCSV to handle these tasks seamlessly. These libraries provide APIs to write data into various formats, including Excel and CSV, ensuring that the stored information is easily accessible and well-organized for future reference or reporting needs.
This functionality not only enhances the efficiency of automation testing but also facilitates better documentation and traceability of test outcomes.
Here’s an example of how to log execution results:
Code Implementation
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.File;
import java.io.FileOutputStream;
public class WriteExcelData {
public static void main(String[] args) {
try {
// Specify the path of the Excel file
File file = new File("TestResults.xlsx");
// Create a new workbook and sheet
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Results");
// Create a header row
Row headerRow = sheet.createRow(0);
headerRow.createCell(0).setCellValue("TestCaseID");
headerRow.createCell(1).setCellValue("Result");
// Add test results
Row row1 = sheet.createRow(1);
row1.createCell(0).setCellValue("TC001");
row1.createCell(1).setCellValue("Pass");
Row row2 = sheet.createRow(2);
row2.createCell(0).setCellValue("TC002");
row2.createCell(1).setCellValue("Fail");
// Write data to the file
FileOutputStream fos = new FileOutputStream(file);
workbook.write(fos);
fos.close();
workbook.close();
System.out.println("Data written successfully.");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Explanation
- Workbook Creation: Creates a new Excel file if one doesn’t exist.
- Sheet and Rows: Adds data row by row.
- File Output Stream: Writes updated data back to the file.
Real-World Applications
Excel is an incredibly versatile tool that finds its place in numerous real-world Selenium testing applications. Below are detailed use cases that highlight how Excel can be utilized effectively to enhance Selenium testing processes:
Data-Driven Testing
Data-driven testing is a widely used approach in Automation testing that allows testers to run multiple test scenarios by varying the input data. Excel plays a crucial role in this methodology:
- Test Input Management: Testers can store a wide range of input data and corresponding expected outcomes in Excel sheets. Selenium scripts can seamlessly fetch this data for executing test cases.
- Parameterization: Excel enables parameterizing test data, allowing dynamic fetching of inputs during Selenium script execution.
- Scalability: For extensive testing scenarios, Excel can handle large datasets efficiently, making it ideal for testing complex web applications.
- Integration with Selenium: Using libraries like Apache POI or OpenCSV, Selenium can interact with Excel to read and write data, streamlining the automation process.
Test Reporting
Efficient test reporting is critical for tracking the progress and outcomes of testing activities. Excel provides a user-friendly and customizable platform for managing Selenium test reports:
- Result Logging: Selenium test scripts can log the pass/fail status, errors, and execution time of each test case directly into Excel.
- Custom Reports: Testers can use Excel features like pivot tables and charts to present Selenium test results in a visually appealing format.
- Documentation: Excel serves as a repository for maintaining detailed documentation of Selenium test results, which is invaluable for audits and future references.
Configuration Management
Managing configuration details is another vital aspect of Selenium testing. Excel simplifies this process by serving as a centralized storage medium:
- Environment Settings: URLs, browser types, credentials, and other environment-specific settings can be stored in an Excel sheet for easy access.
- Dynamic Retrieval: Selenium scripts can dynamically read configuration details from Excel, reducing hardcoding and increasing script flexibility.
- Version Control: Testers can maintain multiple versions of configuration settings in Excel, enabling easy switching between environments like development, testing, and production.
Excel remains a cornerstone in Selenium testing due to its flexibility, simplicity, and powerful data manipulation capabilities. Whether it’s streamlining data-driven testing, generating insightful reports, or managing configuration details, Excel continues to be an indispensable tool for QA professionals aiming to optimize their Selenium testing workflows.
Common Challenges and Solutions
File Lock Issues
- Problem: Errors when accessing an already open Excel file.
- Solution: Ensure the file is closed before running the script.
Unsupported File Formats
- Problem: Errors while handling
.xls
files instead of.xlsx
. - Solution: Use the HSSF library for
.xls
files.
Performance with Large Files
- Problem: Slow processing of large Excel files.
- Solution: Optimize the code by limiting the data fetched or processed in one iteration.
Key Takeaways
- Excel file handling is essential for robust and flexible Selenium test scripts.
- Apache POI simplifies the process of reading and writing Excel files in Java.
- Real-world applications like data-driven testing and reporting make this skill invaluable for automation testers.
- Address common challenges proactively for seamless test execution.
Conclusion
Mastering Excel file operations with Selenium WebDriver is a game-changer for automation testers. This skill empowers you to implement data-driven testing, streamline reporting, and manage configurations with ease. By integrating Apache POI into your Selenium framework, you can handle diverse testing scenarios more effectively and elevate your automation capabilities.
Ready to elevate your Selenium skills? Enroll in H2K Infosys’ Selenium certification Training today and gain hands-on expertise in Selenium automation testing with real-world projects. Transform your career with industry-leading training programs!