Introduction
In the world of web automation, Selenium has become the go-to tool for developers and testers alike. As one of the most widely used frameworks for automating web browsers, Selenium enables testers to interact with web elements and perform a wide range of actions, from clicking buttons to validating text. Among the numerous features provided by Selenium, one of the most straightforward yet essential actions is the “Maximize Browser” command.
But why is maximizing the browser window so important in Selenium automation? How can you use it effectively to improve your testing workflows? In this post, we will delve into the details of the “Maximize Browser” functionality in Selenium, why it matters, and how to implement it in your automation scripts for optimal results.
What Is Selenium?
Before diving into maximizing the browser in Selenium, let’s quickly review what Selenium is and why it has gained such popularity in the realm of automation testing.
Selenium is a free, open-source automation testing framework that allows testers to automate web browsers. It is primarily used for automating web applications for testing purposes, but it can also be used to automate tasks such as data scraping and repetitive browser-based tasks. Selenium supports multiple browsers, including Chrome, Firefox, Safari, and Internet Explorer, making it a versatile tool for cross-browser testing.
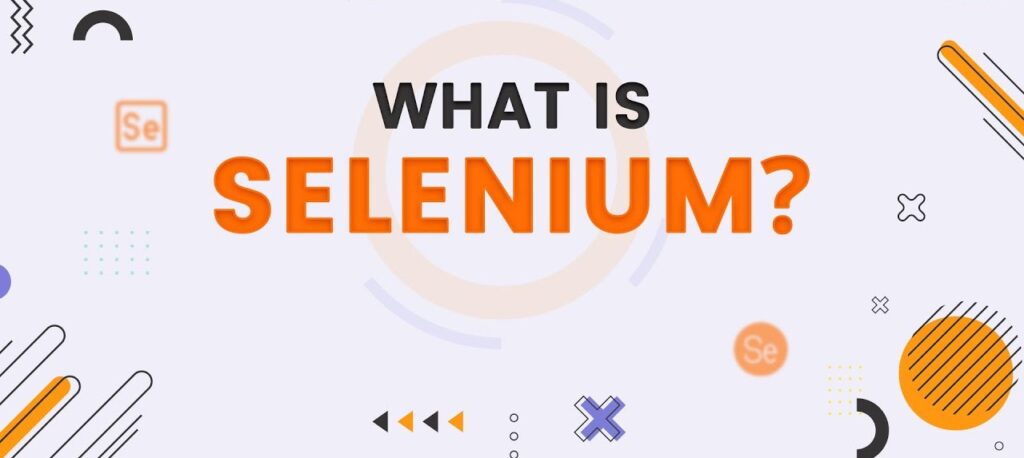
The Selenium WebDriver API provides a way to interact with a web page through a variety of programming languages, including Java, Python, C#, Ruby, and JavaScript. The powerful commands and functions in Selenium allow testers to simulate user actions, validate page elements, and automate complex workflows.
The Importance of Maximizing the Browser in Selenium
In automation testing, one of the first things you may notice when running your tests is that the browser window may not open in full-screen mode. This can affect the behavior of your web application and even result in missed test cases or inaccurate test results. Therefore, maximizing the browser window before executing any tests becomes crucial.
Here are some key reasons why maximizing the browser window in Selenium is essential:
- Improved Element Visibility: Some web elements might be hidden or off-screen when the browser window is not maximized. Maximizing the window ensures that all elements are visible and available for interaction during the test.
- Consistent Test Environment: By maximizing the browser window, you ensure that the application behaves the same way during tests, regardless of the screen resolution or window size. This helps avoid discrepancies in test results caused by window size variations.
- Replicating Real-World Scenarios: In most real-world applications, users interact with web pages in full-screen mode, so maximizing the browser window ensures that tests accurately reflect how users would experience the application.
- Better Interaction with UI Components: Some UI components (such as modal windows, dropdowns, and popups) might only appear or behave differently when the browser is maximized. Maximizing the window ensures that these elements are rendered correctly during tests.
How to Maximize Browser in Selenium
Now that we understand why maximizing the browser in Selenium is important, let’s explore how to implement this functionality in your Selenium automation scripts.
In Selenium, the “maximize” feature is available through the WebDriver interface. Different programming languages have their own syntax for maximizing the browser, but the core concept remains the same.
Maximize Browser in Selenium with Java
To maximize the browser in Selenium WebDriver in Java, you can use the manage().window().maximize()
method. This command is executed after launching the browser and will maximize the window to fill the screen.
Example Code:
java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class MaximizeBrowserExample {
public static void main(String[] args) {
// Set path to ChromeDriver executable
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// Initialize WebDriver
WebDriver driver = new ChromeDriver();
// Open a website
driver.get("https://www.example.com");
// Maximize the browser window
driver.manage().window().maximize();
// Perform additional test actions here
// Close the browser
driver.quit();
}
}
In this example, the manage().window().maximize()
command maximizes the Chrome browser window once the website is loaded.
Maximize Browser in Selenium with Python
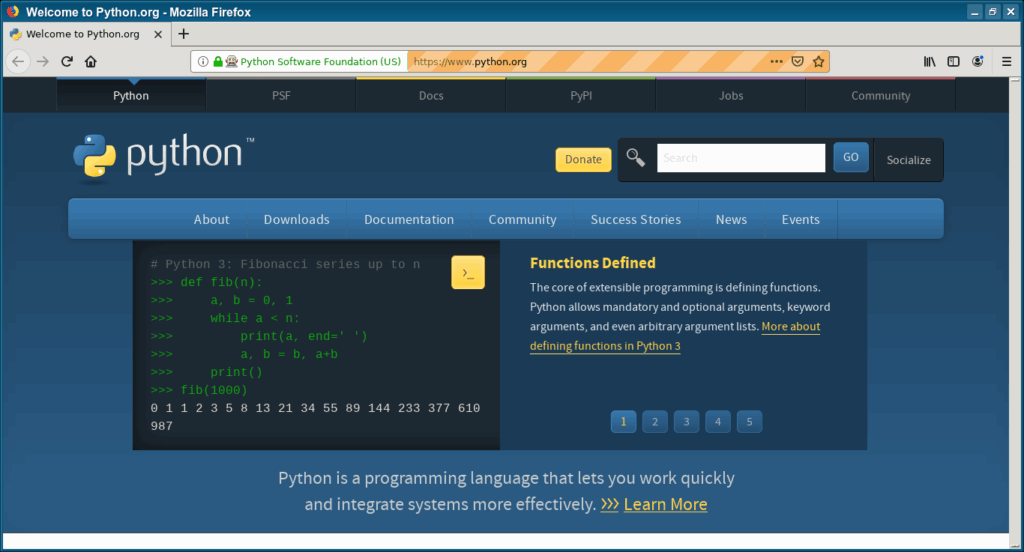
For Python, the process is very similar. We will use the maximize_window()
method from the Selenium WebDriver.
Example Code:
python
from selenium import webdriver
# Initialize WebDriver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open a website
driver.get("https://www.example.com")
# Maximize the browser window
driver.maximize_window()
# Perform additional test actions here
# Close the browser
driver.quit()
Again, the maximize_window()
method is used to ensure the browser window opens in full-screen mode for a consistent testing experience.
Maximize Browser in Selenium with C#
In C#, Selenium provides the Manage().Window.Maximize()
method to maximize the browser in Selenium. Here’s how to use it:
Example Code:
csharp
using OpenQA.Selenium;
using OpenQA.Selenium.Chrome;
class Program
{
static void Main(string[] args)
{
// Initialize WebDriver
IWebDriver driver = new ChromeDriver();
// Open a website
driver.Navigate().GoToUrl("https://www.example.com");
// Maximize the browser window
driver.Manage().Window.Maximize();
// Perform additional test actions here
// Close the browser
driver.Quit();
}
}
This C# example uses Manage().Window.Maximize()
to open the browser in full-screen mode before running any tests.
Common Pitfalls and Troubleshooting
While maximizing the browser in Selenium is generally straightforward, there are a few potential pitfalls to be aware of:
- Browser-Specific Behavior: Some browsers, such as Internet Explorer, might not respond to the maximize command in the same way as Chrome or Firefox. If you face issues with maximizing the window on certain browsers, try using browser-specific drivers or adjusting browser settings.
- Screen Resolution Variations: Maximizing the browser window might not behave consistently on all systems due to varying screen resolutions. To address this, you can use additional commands to set the window size explicitly before running the test.
- Minimized Browser on Remote Machines: When running Selenium tests on remote machines (such as Selenium Grid or cloud-based platforms), ensure that the display is not minimized, as this might prevent the maximize command from executing correctly.
Advanced Techniques for Window Management in Selenium
While maximizing the browser in Selenium window is a simple yet effective command, Selenium also provides additional window management functions that allow you to set custom window sizes or handle multiple browser windows. These techniques can further enhance your test automation.
Custom Window Sizes
In some cases, you might want to set the window to a specific size rather than maximizing it. Selenium WebDriver allows you to adjust the window dimensions to suit your testing needs using the manage().window().setSize()
method.
Example Code (Java):
java
import org.openqa.selenium.Dimension;
driver.manage().window().setSize(new Dimension(1024, 768));
This command sets the window size to 1024×768 pixels, which can be useful for testing applications under different screen resolutions.
Handling Multiple Windows or Tabs
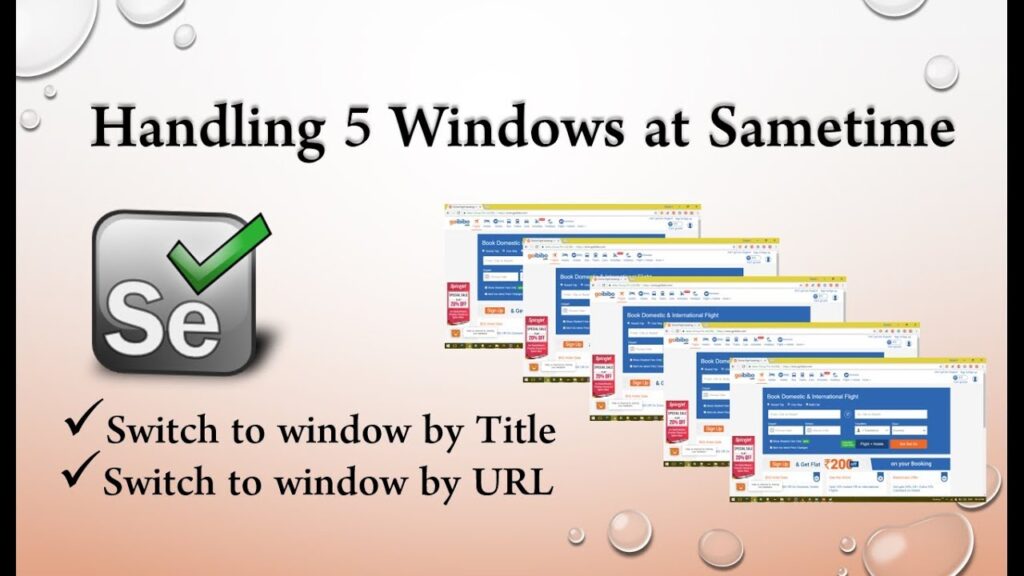
Selenium also supports working with multiple windows or browser tabs. You can switch between them using the windowHandles
property and the switchTo()
method.
Example Code (Python):
python
# Get the window handle of the current window
main_window = driver.current_window_handle
# Open a new tab and switch to it
driver.execute_script("window.open('https://www.example.com', '_blank');")
new_window = driver.window_handles[1]
# Switch to the new tab
driver.switch_to.window(new_window)
# Perform actions in the new tab
# Switch back to the main window
driver.switch_to.window(main_window)
This approach is essential for testing applications that involve multiple windows or popups.
Key Takeaways:
- Maximizing the browser in Selenium is crucial for ensuring consistency in your tests and improving the visibility of UI components.
- Selenium provides simple commands to maximize the browser in selenium across multiple programming languages, including Java, Python, and C#.
- Handling different screen resolutions, browser-specific behavior, and multiple windows are key aspects of managing the browser window effectively in Selenium.
- For learners and professionals interested in expanding their knowledge of Selenium, courses like Selenium certification online or Selenium course online offer comprehensive training to master Selenium and browser management.
Conclusion
Maximizing the Browser in Selenium window is a simple yet powerful command that can significantly improve the reliability and consistency of your Selenium-based automation tests. Whether you’re a beginner or an experienced tester, mastering this concept is fundamental to building robust test automation frameworks. For a deeper dive into Selenium automation testing and to enhance your skills, consider enrolling in a Selenium course online or pursuing Selenium certification online.
By gaining hands-on experience and learning best practices, you can elevate your testing career and contribute to more efficient and accurate automation processes. Enroll today at H2K Infosys for comprehensive training and certification to master Selenium and take your skills to the next level!
Want to become an expert in Selenium automation testing? Join H2K Infosys today and gain hands-on experience with real-world projects!
One Response