Introduction to Maximize Browser in Selenium
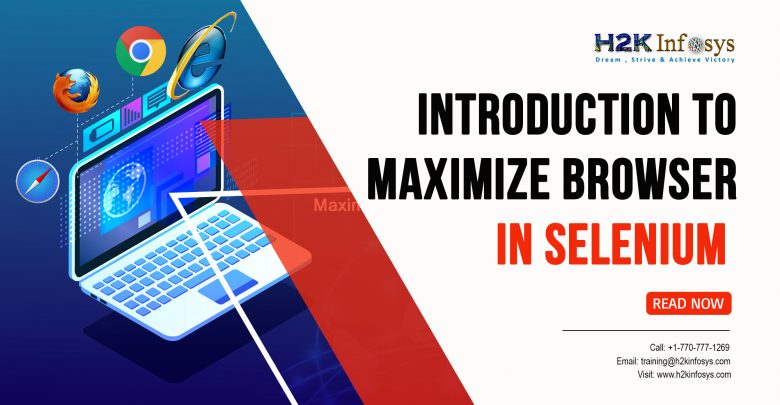
Maximizing the browser is very important in the selenium framework while automating any web application. While executing the selenium framework or any script, the browser may not be in the full-screen state. Thus we need to maximize the browser so that all the elements of the web application are visible.
You should maximize the browser at the start of the script so that the script gets executed successfully without any error. If the browser is not maximized, all the elements on the web application may not be visible by the selenium, and the framework will fail.
There are several ways to maximize or minimize the browser. Selenium Webdriver does not provide any direct method for minimizing the browser. For minimizing the browser in Selenium Webdriver, we need to use the resize method.
Some of the methods to resize the current browser window in Selenium Webdriver are:
How to Resize a Browser in Selenium Webdriver:
Below, the Selenium script depicts how to resize a browser. This script will follow the following steps:
- Opens the Google Chrome browser.
- Navigate to the given site URL.
- Resize the browser window.
- Close the browser window.
public class ResizeBrowser { public static void main(String args[]) { System.setProperty("webdriver.chrome.driver","Path of the Chrome Driver (E.g. D://scripts//chromedriver.exe) "); WebDriver driver = new ChromeDriver(); driver.get("https://www.google.com/"); Dimension d = new Dimension(200,1070); //Resize current window to set dimension driver.manage().window().setSize(d); //Close the browser driver.quit(); } }
How to Maximize a Browser in Selenium Webdriver:
The below script depicts how to maximize a browser. This script will follow the following steps:
-
- Opens the chrome browser.
- Navigate to the given site URL.
- Maximize the browser window.
- Close the browser window.
import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class Maximize { public static void main(String args[]) throws InterruptedException { System.setProperty("webdriver.chrome.driver","Path of the Chrome Driver (E.g. D://scripts//chromedriver.exe) "); WebDriver driver = new ChromeDriver(); driver.get("https://www.google.com/"); //Maximize current window driver.manage().window().maximize(); //Close the browser driver.quit(); } }
How to Minimize a Browser in Selenium Webdriver:
The below script depicts how to minimize a browser. This script will follow the following steps:
-
- Opens the chrome browser.
- Navigate to the given site URL.
- Minimize the browser window.
- Close the browser window.
import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class Maximize { public static void main(String args[]) throws InterruptedException { System.setProperty("webdriver.chrome.driver","Path of the Chrome Driver (E.g. D://scripts//chromedriver.exe) "); WebDriver driver = new ChromeDriver(); driver.get("https://www.google.com/"); Point p = new Point(0,2000); //Minimize the current window to set position driver.manage().window().setPosition(p); //Close the browser driver.quit(); } }
Note: If the user wants to use the Firefox browser instead of the Chrome browser, then set the property of FirefoxDriver and create FirefoxDriver object instead of ChromeDriver.
System.setProperty(“webdriver.gecko.driver”,”Path of the Firefox Driver (E.g. D://scripts//geckodriver.exe) “);
WebDriver driver = new FirefoxDriver();