Unit Testing: A Comprehensive Guide to Ensuring Software Quality
Unit Testing is a fundamental practice in software development, ensuring that individual components of an application work as intended. This extensive guide will explore the intricacies of unit testing, its importance, how it differs from integration testing, best practices, tools, and practical examples to help you implement unit testing effectively. We will also delve into challenges and strategies for overcoming them, providing a thorough understanding of this crucial aspect of software development.
What is Unit Testing?
Unit testing is a type of software testing where individual units or components of a software application are tested in isolation. A unit is the smallest testable part of an application, such as a function, method, or object. The main objective of unit testing is to ensure that each unit functions correctly on its own.
Key Characteristics of Unit Testing
- Isolation: Units are tested independently from other parts of the application to ensure their functionality is correct.
- Automation: Unit tests are typically automated, which allows for continuous testing and integration.
- Early Detection: By testing units early in the development process, defects can be identified and fixed before they escalate into more significant issues.
Unit Testing vs QA testing
Unit testing and Quality Assurance (QA) testing are both crucial components of the software development lifecycle, serving distinct but complementary purposes.
Unit testing is a type of software testing where individual components or pieces of code are tested to ensure they work as expected. Typically written and performed by developers, unit tests are used to validate that each unit of the software performs correctly. These tests are usually automated and run every time changes are made to the codebase to ensure new changes don’t break existing functionality. Unit testing is highly specific, focusing on very small parts of the application, such as functions or methods, and it is the first line of defense in identifying bugs and issues early in the development process.
On the other hand, QA testing encompasses a broader view of the software to ensure its overall quality. This type of testing is typically conducted by professional testers or QA engineers who evaluate the complete application in various environments to simulate real-world usage. QA testing includes various methodologies like integration testing, system testing, acceptance testing, and more. It focuses on aspects such as functionality, performance, security, and user experience, aiming to identify any discrepancies from the initial requirements or potential improvements.
While unit testing is focused on correctness and the technical aspects of individual components, QA testing ensures that the software as a whole meets the business and user requirements and delivers a seamless user experience. Together, they help in delivering a robust and reliable software product.
Importance of Unit Testing
Unit testing is vital for maintaining and improving software quality. Here are some key reasons why unit testing is essential:
Early Bug Detection
Unit tests enable developers to catch bugs early in the development process. Early detection reduces the cost and effort required to fix defects compared to finding them later in the development lifecycle or after the software has been deployed.
Simplifies Integration
Since unit tests verify the functionality of individual units, integrating these units into a complete system becomes more straightforward. Developers can be more confident that if individual units work correctly, their integration will likely be smooth.
Facilitates Refactoring
Refactoring is the process of restructuring existing code without changing its external behavior. Unit tests provide a safety net during refactoring, ensuring that changes do not introduce new bugs. This encourages developers to improve code quality without fear of breaking existing functionality.
Documentation
Unit tests serve as a form of documentation. They provide examples of how units of code are intended to be used and what their expected outputs are for given inputs. This can be incredibly helpful for new developers or when revisiting code after some time.
Improves Design
Writing unit tests often leads to better software design. It encourages developers to write modular, cohesive, and loosely coupled code that is easier to test.
Unit Testing vs. Integration Testing
While unit testing focuses on individual units, integration testing examines how different units work together. Understanding the distinction between these two types of testing is crucial for comprehensive software testing.
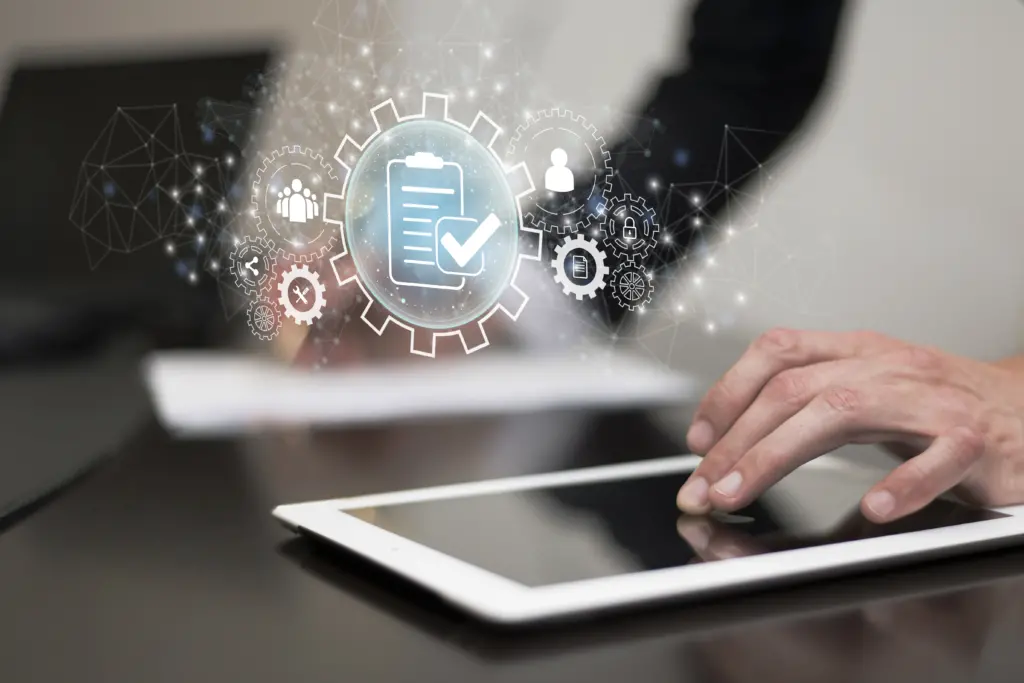
Unit Testing
- Scope: Tests individual units or components in isolation.
- Objective: Ensure each unit functions correctly on its own.
- Dependency: No dependencies on other units or external systems.
- Tools: JUnit, NUnit, pytest, etc.
Integration Testing
- Scope: Tests the interactions between integrated units or components.
- Objective: Ensure that combined units work together as expected.
- Dependency: Requires multiple units or components to be integrated and sometimes involves external systems.
- Tools: Selenium, JUnit (for integration tests), TestNG, etc.
Key Differences
- Focus: Unit testing targets individual units, while integration testing focuses on the interaction between units.
- Complexity: Unit tests are generally simpler and faster to execute than integration tests, which can be more complex and time-consuming due to the involvement of multiple components.
- Execution Frequency: Unit tests are executed frequently during development, whereas integration tests are typically run less often, such as during build processes or before release.
How do developers use unit tests?
Developers use unit tests to ensure that individual components or units of source code are functioning correctly. These tests are typically written and executed by software developers to confirm that each part of the application behaves as expected under various conditions.
Unit tests are a foundational element of Test-Driven Development (TDD), a methodology where tests are written before the actual code. This approach helps in defining desired behaviors and validating that the code meets its specifications. By isolating each part of the program and testing it separately, developers can catch and fix errors early in the development cycle, which improves the quality of the software and reduces debugging time later on.
Moreover, unit tests contribute to the documentation of the code. They provide insights into what the code is supposed to do, making it easier for new team members to understand the existing codebase. This aspect is crucial when teams grow or when turnover occurs.
Unit tests also facilitate refactoring, which is the process of restructuring existing code without changing its external behavior. Since the tests cover the functionality of individual units, developers can modify the code with confidence, knowing that any regression or deviation from the original behavior will be caught by the tests.
Automating these tests and integrating them into a continuous integration/continuous deployment (CI/CD) pipeline ensures that every change made to the codebase is automatically tested, thereby maintaining code quality throughout the development process. This practice is essential in agile development environments, where frequent changes and iterative updates are common.
Unit Testing Best Practices
Unit testing is a foundational practice in software development that helps ensure individual components of an application work as intended. To maximize its effectiveness, there are several best practices developers can follow:
- Write Clear, Simple Tests: Each unit test should be concise and focus on a single functionality. This makes tests easier to write, understand, and maintain. Avoid complex dependencies in unit tests to ensure that they are not fragile and do not break with minor changes in the codebase.
- Practice Test-Driven Development (TDD): This involves writing tests before writing the code they are meant to test. TDD encourages thoughtful design and cleaner, more bug-resistant code by focusing development around fulfilling test requirements.
- Isolate Tests: Tests should be isolated, meaning they do not depend on external databases, files, or other components. Use mocking and stubbing techniques to simulate these dependencies, allowing tests to run quickly and reliably.
- Cover Edge Cases: It’s crucial to test not only the typical paths through the code but also the edge cases. This includes testing for null inputs, unexpected values, and boundary conditions.
- Ensure Tests are Repeatable and Independent: Each test should be able to run independently of others and produce the same results every time, regardless of external factors like the environment or the order in which tests are run.
- Automate and Integrate Continuously: Automate the execution of unit tests to run with every build or integration cycle. This helps catch issues early and improves the quality of the software continuously.
- Maintain Documentation: Well-documented tests can serve as an excellent reference for the expected behavior of the system. This is especially useful for new team members or when revisiting a module after some time.
By adhering to these best practices, developers can create a robust suite of unit tests that enhance the stability and maintainability of their applications, leading to higher quality software and more efficient development cycles.
To maximize the effectiveness of unit testing, developers should follow best practices. Here are some guidelines to consider:
Write Independent Tests
Each unit test should be independent of others. Tests should not rely on the outcome of other tests and should not share state. This ensures that tests are reliable and can be run in any order.
Use Descriptive Names
Test names should clearly describe what they are testing. This makes it easier to understand the purpose of each test and what aspect of the unit’s functionality is being verified.
Follow the Arrange-Act-Assert (AAA) Pattern
The AAA pattern is a common structure for writing unit tests:
- Arrange: Set up the test environment, including creating objects and setting initial states.
- Act: Execute the unit of code being tested.
- Assert: Verify that the outcome matches the expected result.
Mock Dependencies
When a unit depends on external systems or other units, use mock objects to simulate these dependencies. Mocking allows for isolated testing and can help simulate different scenarios, such as errors from external services.
Keep Tests Small and Focused
Each test should focus on a single aspect of the unit’s functionality. Small, focused tests are easier to understand, maintain, and debug.
Run Tests Frequently
Integrate unit tests into the development workflow, running them frequently to catch issues early. Automated testing tools and continuous integration systems can facilitate this practice.
Maintain Tests
Unit tests should be maintained alongside the code they test. If the code changes, the tests should be updated to reflect those changes. Neglecting test maintenance can lead to false positives or negatives, reducing the reliability of the tests.
Tools and Frameworks for Unit Testing
Several tools and frameworks can help automate and streamline unit testing. Here are some popular ones:
JUnit
JUnit is a widely used testing framework for Java applications. It provides annotations to identify test methods and supports various assertions to verify test outcomes.
NUnit
NUnit is a unit-testing framework for .NET languages. It is similar to JUnit but designed for use with .NET applications.
pytest
pytest is a powerful testing framework for Python. It supports fixtures, parameterized testing, and has a rich plugin architecture.
TestNG
TestNG is another testing framework for Java that is inspired by JUnit but includes additional features like data-driven testing and parallel test execution.
xUnit.net
xUnit.net is a free, open-source, community-focused unit testing tool for the .NET Framework. It is the latest technology in the xUnit family and is designed to address some of the issues with NUnit.
Prerequisite of Unit Testing
Implementing unit testing effectively requires adherence to several prerequisites that ensure the process is both robust and efficient. Firstly, the codebase needs to be modular; this architecture facilitates the isolation of individual units, which are the smallest testable parts of an application. Each unit should have a single responsibility, making it straightforward to test without dependencies on other units.
Developers must have a comprehensive understanding of the software’s architecture and behavior to write effective tests. This knowledge aids in identifying critical areas where tests should focus, ensuring that each unit performs its intended function correctly. Additionally, selecting the right testing framework is crucial. For example, JUnit is popular for Java, PyTest for Python, and NUnit for .NET applications. These frameworks provide tools and conventions for writing, organizing, and running tests efficiently.
Another important aspect is the integration of the testing process into the development workflow. This integration often involves setting up a continuous integration/continuous deployment (CI/CD) pipeline that automatically runs tests with each code commit, helping catch issues early and frequently.
Finally, fostering a development culture that values testing and quality assurance is essential. This cultural aspect ensures that all team members prioritize testing and maintain high standards in the codebase. Encouraging collaboration and regular code reviews also helps improve test quality and coverage, making the unit testing process more effective and valuable.
Workflow of Unit Testing
The workflow of unit testing is a structured process that ensures each component of an application works as intended independently before integration. This workflow begins with the planning phase, where developers define what to test and identify the criteria for successful tests. This involves understanding the functionality of the units and determining the input and output expectations.
Following planning, developers write the unit tests. In a test-driven development (TDD) approach, this step actually precedes writing the functional code. Tests are written based on the specifications and are designed to fail initially. Developers then write or modify the application code until the tests pass, ensuring the code meets the desired specifications.
Once the tests are written and the code is implemented, the execution phase involves running these tests. This is typically automated through a testing framework which provides tools to easily execute all tests and report results. Common frameworks include JUnit for Java, PyTest for Python, and NUnit for .NET.
After execution, the results are analyzed. Successful tests confirm correct behavior, while failed tests require debugging and code revision. Developers fix the code and retest until all tests pass reliably.
Finally, maintaining and updating tests is crucial as the application evolves. As new features are added or existing features are modified, corresponding unit tests must also be updated or new tests added to ensure ongoing reliability and performance. This ongoing maintenance is vital to sustain the integrity of the application over time.
Unit Testing Techniques
Unit testing techniques are varied and designed to ensure that every aspect of a unit is tested thoroughly to produce reliable and robust software. Among the most common techniques are:
- Black-box Testing: This technique is used when the tester has no knowledge of the internal workings of the item being tested. Tests are based on requirements and functionality, not on how the function is implemented. This focuses on input and output and is useful in detecting unexpected behavior changes.
- White-box Testing: In contrast to black-box testing, white-box testing involves a thorough knowledge of the internal workings of the code. Testers use this knowledge to write test cases that cover all paths through the code, including loops and conditional branches, ensuring comprehensive coverage.
- Boundary Value Analysis: This is particularly useful in testing edge cases. It involves testing at the boundaries between partitions. For instance, if a function is supposed to handle inputs from 1 to 10, testing would be conducted at values just around and at the boundaries, such as 0, 1, 10, and 11.
- Equivalence Partitioning: This technique divides input data into valid and invalid partitions and tests from each partition. The aim is to uncover errors in handling different classes of input.
- State-based Testing: This approach is useful when the software’s behavior is dependent on its state. Test cases are designed to test the same functionality under various states.
- Error Guessing: This relies on the tester’s experience to guess the most probable areas of error in a unit. Test cases are developed based on common errors or past experiences.
By integrating these techniques, developers can create a comprehensive suite of unit tests that not only verifies the correctness of the code but also enhances its reliability and maintainability.
Implementing Unit Testing: A Practical Example
To illustrate how unit testing works in practice, let’s walk through an example using Python and pytest.
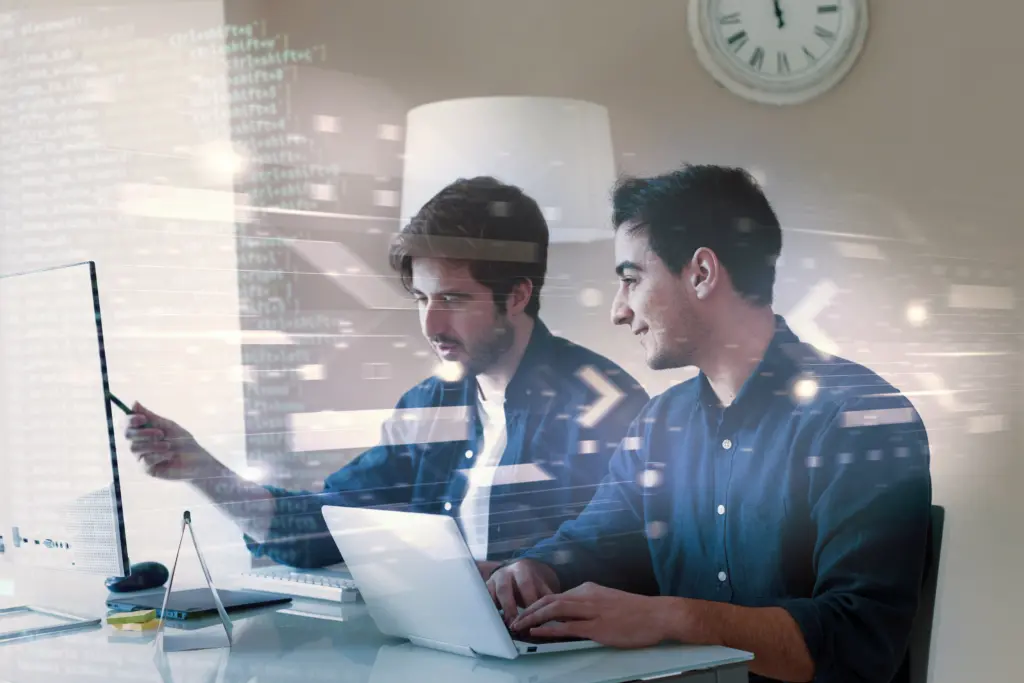
Example: Testing a Calculator Class
Consider a simple Calculator
class with methods for addition, subtraction, multiplication, and division. We want to write unit tests to verify that each method works correctly.
Calculator Class
pythonCopy codeclass Calculator:
def add(self, a, b):
return a + b
def subtract(self, a, b):
return a - b
def multiply(self, a, b):
return a * b
def divide(self, a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
Unit Tests with pytest
pythonCopy codeimport pytest
from calculator import Calculator
@pytest.fixture
def calculator():
return Calculator()
def test_add(calculator):
assert calculator.add(2, 3) == 5
assert calculator.add(-1, 1) == 0
assert calculator.add(-1, -1) == -2
def test_subtract(calculator):
assert calculator.subtract(3, 2) == 1
assert calculator.subtract(-1, 1) == -2
assert calculator.subtract(-1, -1) == 0
def test_multiply(calculator):
assert calculator.multiply(2, 3) == 6
assert calculator.multiply(-1, 1) == -1
assert calculator.multiply(-1, -1) == 1
def test_divide(calculator):
assert calculator.divide(6, 3) == 2
assert calculator.divide(-1, 1) == -1
assert calculator.divide(-1, -1) == 1
with pytest.raises(ValueError):
calculator.divide(1, 0)
Running the Tests
To run the tests, navigate to the directory containing the test file and run the following command:
bashCopy codepytest
pytest will discover and execute the tests, providing a report of the results.
Challenges in Unit Testing
While unit testing offers numerous benefits, it also comes with challenges that developers must address:
Writing Tests for Legacy Code
Legacy code often lacks unit tests, and adding tests can be difficult, especially if the code is not well-designed or modular. Refactoring may be necessary to make the code more testable, but this can introduce risks.
Ensuring Test Coverage
Achieving high test coverage, where a large percentage of the codebase is covered by unit tests, can be challenging. It’s essential to prioritize critical areas and ensure that tests are meaningful rather than aiming for arbitrary coverage metrics.
Mocking Complex Dependencies
Mocking can become complex when units have many dependencies or interact with external systems. Properly managing mocks and ensuring they accurately simulate real-world scenarios is crucial for reliable tests.
Maintaining Tests
As the codebase evolves, maintaining unit tests can become a significant task. Tests must be updated to reflect changes in the code, and neglecting this can lead to a brittle test suite that fails frequently or provides false positives.
Strategies for Effective Unit Testing
Given the challenges, here are some strategies to help ensure your unit testing efforts are effective and sustainable:
Test-Driven Development (TDD)
Test-Driven Development is a software development approach where tests are written before the code. The cycle involves writing a failing test, writing the minimum code to pass the test, and then refactoring the code while keeping the tests passing. TDD helps ensure that tests are integral to the development process and that code is written with testing in mind.
Continuous Integration (CI)
Integrating unit testing into a continuous integration (CI) system ensures that tests are run automatically with each code change. CI systems like Jenkins, Travis CI, and GitHub Actions can automate the execution of tests, providing immediate feedback on code changes.
Code Reviews
Incorporate unit tests into code reviews. Reviewing both the code and its associated tests ensures that tests are well-written and cover the intended functionality. It also helps spread knowledge about testing practices across the development team.
Use Coverage Tools
Code coverage tools measure the percentage of code that is tested by unit tests. Tools like JaCoCo for Java, Coverage.py for Python, and Istanbul for JavaScript can help identify untested parts of the codebase, guiding efforts to improve test coverage.
Mocking and Stubbing
Use mocking and stubbing to isolate units from their dependencies. Libraries like Mockito for Java, unittest.mock for Python, and Sinon.js for JavaScript provide powerful features for creating mocks and stubs.
Parameterized Tests
Parameterized tests allow the same test to run with different sets of data. This helps ensure that units work correctly across a range of inputs. Most testing frameworks support parameterized tests, such as JUnit’s @ParameterizedTest
and pytest’s parameterized fixtures.
Advanced Topics in Unit Testing
For those looking to delve deeper into unit testing, here are some advanced topics and techniques:
Behavior-Driven Development (BDD)
Behavior-Driven Development extends TDD by writing tests in a natural language that describes the behavior of the application. Tools like Cucumber for Java and SpecFlow for .NET support BDD by allowing tests to be written in a readable, domain-specific language.
Mutation Testing
Mutation testing is a technique to evaluate the quality of your unit tests. It involves making small changes (mutations) to your code and checking if your tests detect these changes. If tests pass with the mutated code, it indicates that the tests may not be thorough enough. Tools like PIT for Java and MutPy for Python support mutation testing.
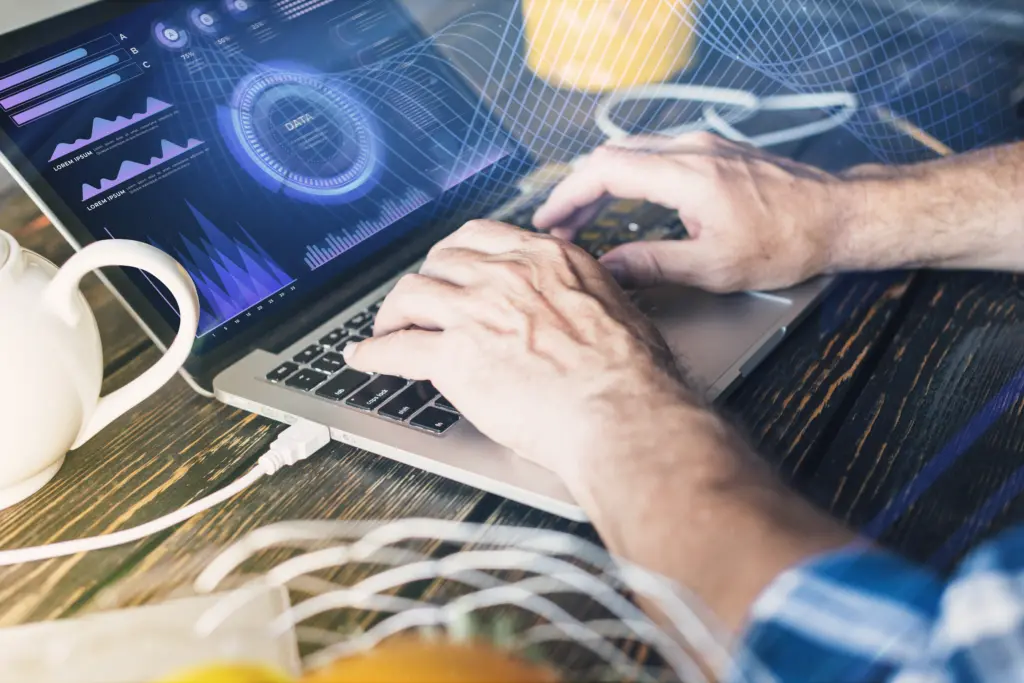
Property-Based Testing
Property-based testing involves defining properties that your code should satisfy and then generating a wide range of inputs to test these properties. Tools like QuickCheck for Haskell and ScalaCheck for Scala support property-based testing.
Testing Asynchronous Code
Testing asynchronous code, such as code involving callbacks, promises, or async/await, can be challenging. Ensure your testing framework supports asynchronous tests, and use features like mock timers and stubs to control asynchronous behavior during tests.
Future of Unit Testing
The field of unit testing continues to evolve with advancements in technology and practices. Here are some trends and future directions:
AI and Machine Learning
Artificial Intelligence (AI) and Machine Learning (ML) are starting to play a role in unit testing. Tools are emerging that can automatically generate unit tests by analyzing code and identifying edge cases. These tools can help improve test coverage and reduce the manual effort required to write tests.
Improved Tooling and Integration
The integration of unit testing tools with development environments is becoming more seamless. IDEs like Visual Studio Code, IntelliJ IDEA, and PyCharm provide built-in support for running and debugging unit tests, making it easier for developers to write and maintain tests.
Focus on Performance Testing
While traditional unit testing focuses on functionality, there is a growing emphasis on performance testing at the unit level. Ensuring that individual units perform efficiently can lead to overall performance improvements in the application.
Enhanced Collaboration
As development teams become more distributed, tools that facilitate collaboration on unit tests are becoming more important. Features like shared test cases, collaborative debugging, and real-time feedback are becoming standard in modern development environments.
Advantages of Unit Testing
Unit testing offers several significant benefits that contribute to the overall quality and reliability of software. Here are the key advantages:
- Early Bug Detection: Unit tests catch bugs early in the development process, making them easier and less costly to fix.
- Simplifies Integration: By ensuring each unit works correctly in isolation, unit testing facilitates smoother integration, reducing the likelihood of issues when combining different parts of the system.
- Facilitates Refactoring: Unit tests provide a safety net during refactoring, allowing developers to improve and clean up code without fear of introducing new bugs.
- Improves Code Quality: Writing unit tests encourages developers to write more modular, cohesive, and loosely coupled code, leading to better overall software design.
- Documentation: Unit tests serve as documentation that describes how the code is supposed to work, making it easier for new developers to understand the functionality and expected behavior of the code.
- Supports Continuous Integration: Automated unit tests are a crucial part of continuous integration (CI) pipelines, ensuring that new changes do not break existing functionality and helping maintain a stable codebase.
- Enhances Confidence: Knowing that the code has been thoroughly tested boosts developer confidence in making changes, adding new features, and maintaining the software.
Disadvantages of Unit Testing
While unit testing offers numerous benefits, it also comes with certain drawbacks that developers need to be aware of:
- Initial Time Investment: Writing unit tests requires an upfront time commitment, especially when setting up the testing framework and writing tests for existing codebases. This can slow down the initial development pace.
- Maintenance Overhead: As the codebase evolves, unit tests need to be updated to reflect changes. This ongoing maintenance can be time-consuming and requires diligence to ensure tests remain relevant and accurate.
- Limited Scope: Unit tests only verify the functionality of individual units in isolation. They do not catch issues that arise from the interaction between different components or the overall system behavior.
- False Sense of Security: Relying solely on unit tests can create a false sense of security. Comprehensive testing should include other types of tests, such as integration, system, and acceptance testing, to cover different aspects of the application.
- Complexity with Dependencies: Testing units that have complex dependencies or interact with external systems can be challenging. Properly mocking or stubbing these dependencies requires additional effort and can complicate the test setup.
- Difficulty in Testing UI Components: Unit testing is generally not suitable for testing user interface components or user experience aspects, which require different testing approaches, such as UI testing or usability testing.
Overall, while unit testing is a powerful tool for ensuring code quality, it is important to recognize its limitations and use it in conjunction with other testing methods for comprehensive software validation.
When is Unit Testing Less Beneficial?
Unit testing, while invaluable in many scenarios, may be less beneficial under certain conditions:
- Highly Interdependent Code: In systems with highly interdependent code, isolating units for testing can be challenging and may not provide significant insights. The effort required to mock dependencies might outweigh the benefits.
- Rapid Prototyping: During the initial stages of rapid prototyping or proof-of-concept projects, the focus is on quickly validating ideas rather than ensuring robust, production-quality code. In such cases, unit testing might slow down the iterative development process.
- Legacy Code with Poor Design: Introducing unit tests to legacy code that lacks modularity and has poor design can be difficult and time-consuming. Extensive refactoring might be required to make the code testable, which may not always be feasible.
- Simple and Stable Code: For simple, stable, and rarely changing code, the cost of writing and maintaining unit tests might not justify the benefits. Examples include utility functions that perform straightforward tasks.
- Tight Deadlines: In projects with extremely tight deadlines, the time required to write comprehensive unit tests might be better spent on delivering core functionality. However, this should be a carefully considered trade-off, as skipping tests can lead to more significant issues later.
- UI and Integration Focused: When the primary concern is the overall integration and user interface, unit tests might not capture the necessary aspects of user interactions and system behaviors. In such cases, integration testing and UI testing are more appropriate.
While unit testing is a critical part of quality assurance, understanding when it is less beneficial helps in making informed decisions about testing strategies.
Conclusion
Unit testing is a critical practice in software development that ensures the reliability, maintainability, and quality of code. By focusing on individual units of code, developers can catch bugs early, simplify integration, facilitate refactoring, and improve overall code quality.
Understanding the differences between unit testing and integration testing, following best practices, leveraging the right tools and frameworks, and addressing common challenges are essential for effective unit testing. As technology and practices continue to evolve, staying informed about advanced topics and future trends will help you keep your unit testing efforts effective and relevant.
Implementing unit testing may require an initial investment of time and effort, but the long-term benefits far outweigh the costs. By making unit testing an integral part of your development process, you can create robust, high-quality software that meets the needs of users and stands the test of time. check out qa tester course online to get certified in qa.
19 Responses
It tests individual units or components of software.
It verifies that each unit of the software code performs as expected.
It is done during the development of an application by the developers.
The main reasons to perform unit testing are:
*Unit testing helps us to fix the bugs early in the development phase and save costs.
*It helps developers to understand the testing code base and also activates them to make changes quickly.
*Good unit tests give project documentation.
*Unit test help to re-use the code.
*Migrate your code and test to our new project.
Unit testing can be done in two ways:
1.Manual
2.Automated
Unit testing:
Unit testing is a type of software testing where the individual units or components of software are tested. The goal is to verify that each unit of the software code performs as expected. Unit testing is done during the development of an application by the developers.
The main reasons to perform unit testing are:
1. Unit testing helps us to fix the bugs early in the development phase and save costs.
2. It helps developers to understand the testing code base and also activates them to make changes quickly.
3. Good unit tests give project documentation.
4. Unit test help to re-use the code.
5. Migrate your code and test to our new project.
Unit testing can be done in two ways:
1. Manual
2. Automated
Unit testing is basically automated but may be still performed manually. Software engineering doesn’t favour over the other but automation is preferred.
Is to test each unit or component of the software. It is also to make sure that the code of each unit performs correctly. It also helps fix bugs, makes changes, give project documentation, test help to re-use the code and to migrate to test new codes. There are two ways to do unit testing, manual and automatic. Most unit testing are done automatic. The developer can write a code to continuously test function and later remove it.
Unit testing is a type of software testing where the individual units or components of software are tested.done by the developer.unit testing is done in manual or automation testing.unit testing helps to identify the defects in the early stage and saves cost.Unit testing help in reusing the code by migrating your code and test new project.
Unit Testing is done on smaller chunks of code by isolating the code and testing a small section.
Unit testing is very useful as it helps in early detection of defects and proves to be cost effective. It helps the developers understand the code base better, it makes to easy documentation of testing, it makes the code reusable thereby, easy to migrate and run the code for new project.
Unit testing is a type of software testing where the individual units or components of software are tested. The goal is to verify that each unit of the software code performs as expected. Unit testing is done during the development of an application by the developers. Unit tests isolate a section of code and verify its accuracy.
Unit testing is important because software developers try to save time doing minimal unit testing. Inappropriate unit testing may lead to higher defect fixing cost.
The main reasons to perform unit testing are:
1. Unit testing helps us to fix the bugs early in the development phase and save costs.
2. It helps developers to understand the testing code base and also activates them to make changes quickly.
3. Good unit tests give project documentation.
4. Unit test help to re-use the code.
5. Migrate your code and test to our new project.
Unit testing can be done in two ways:
1. Manual
2. Automated
Unit testing is a type of testing where it’s test individual unit or component of the software.It helps developers to understand the testing code base. There are 2 ways to do unit testing 1. Manual and 2.Automated but automated is a preferred way.The goal of unit testing is to verify each unit of the software code perform as expected.Unit testing is important because developers will try to save time by doing minimal unit testing.
Unit testing tests individual units or components of software.
It verifies that each unit of the software code performs as expected.
It is done during the development of an application by the developers.
The main reasons to perform unit testing are:
1)Unit testing helps us to fix the bugs early in the development phase and save costs.
2) it helps developers to understand the testing code base and also activates them to make changes quickly.
3)Good unit tests give project documentation.
4) unit test help to re-use the code.
5)Migrate your code and test to our new project.
Unit testing can be done in two ways:
1.Manual
2.Automated
Unit testing is a type of software testing where the individual units or components of software are tested. The goal is to verify that each unit of the software code performs as expected. Unit testing is done during the development of an application by the developers. Inappropriate unit testing may lead to higher defect fixing cost. If the defects are identified at later test levels like integration testing, system testing, and even beta testing after the application is built the defect fixing cost will be higher.
The main reasons to perform unit testing are:
1. Unit testing helps us to fix the bugs early in the development phase and save costs.
2. It helps developers to understand the testing code base and also activates them to make changes quickly.
3. Good unit tests give project documentation.
4. Unit test help to re-use the code.
5. Migrate your code and test to our new project
Unit testing can be done in two ways: 1.Manual 2.Automated . Unit testing is basically automated but may be still performed manually.
Unit testing is a type of software testing where the individual units or components of software are tested. The goal is to verify that each unit of the software code performs as expected. This type of testing is done by developers during the developmental stage. The developers verify accuracy of a isolated section of code. There are two types of unit testing. These are manual testing and automated testing. Reasons for unit testing are as follows:
-Unit testing helps us to fix the bugs early in the development phase and save costs.
-It helps developers to understand the testing code base and also activates them to make changes quickly.
-Good unit tests give project documentation.
-Unit test help to re-use the code.
-Migrate your code and test to our new project
Unit testing is type of software testing where units or components of software are tested to verify that each unit is performing as expected. Main reasons to perform unit testing are:
1. 1.Unit testing helps us to fix the bugs early in the development phase and save costs.
2. It helps developers to understand the testing code base and also activates them to make changes quickly.
3. Good unit tests give project documentation.
4. Unit test help to re-use the code.
5. Migrate your code and test to our new project.
There are two ways to do unit testing. They are: manual and automated.
A coder generally uses the unit testing framework to develop automated tests. Using this automation framework, the developer codes criteria into test to validate the accuracy of the code. The workflow of unit test includes to create test cases, review/rework, baseline and execution of test cases.
Unit Testing is a type of testing where the individual units of software are tested during its development phase. Each unit of software needs to be tested and verified.
Testing at the initial level may decrease the defect fixing cost. unit testing helps to fix the bugs, understand the testing code base, helps to make changes quickly, give project documentation, helps to reuse the code, Migrate code and test to our new project.
Unit Testing can be done in two ways:
1. Manual
2. Automated
Unit testing is basically automated but also can be done Manually.
Unit testing:
Unit testing is a type of software testing where the individual units or components of software are tested. The goal is to verify that each unit of the software code performs as expected. Unit testing is done during the development of an application by the developers.
The main reasons to perform unit testing are:
1. Unit testing helps us to fix the bugs early in the development phase and save costs.
2. It helps developers to understand the testing code base and also activates them to make changes quickly.
3. Good unit tests give project documentation.
4. Unit test help to re-use the code.
5. Migrate your code and test to our new project.
Unit testing can be done in two ways:
1. Manual
2. Automated
Unit testing is basically automated but may be still performed manually. Software engineering doesn’t favour over the other but automation is preferred.
Unit Testing:
If we perform a test on an individual unit or component of a software, it is called unit testing.
It verifies that each unit of the software code performs as expected.
Unit testing can help finding and fixing bugs in early stage of software development (if there are any), so it definitely saves cost of the software building process.
It is done during the development of an application by the developers.
Unit testing can be done manually or by automated process.
Unit testing is usually done by developers to verify the software code. Unit testing helps fix bugs, save coast make changes, give product documentation, and migrate to test new codes. A unit test can be done in two ways Manual or Automatic.