Introduction to ParseInt in Java
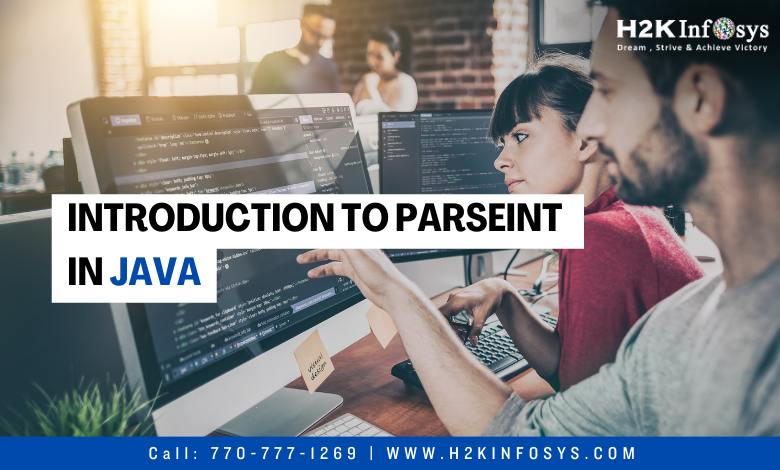
You’ve probably heard about “parseInt” if you’re a programmer in Java or even simply a beginner trying to learn more about the language. However, what does this mean and how can one apply it?
You will discover the definition of parseInt and its applications in Java programming in this post. Additionally, you’ll discover important pointers to remember when utilizing parseInt in your code. To learn more, check out our Java online training.
In Java, what does parseInt do?
A Java package called ParseInt offers a straightforward method for parsing and validating information from outside sources. It doesn’t rely on any other libraries and is made to be simple to use and light. Any Java application, including standalone and web apps, can use ParseInt.
ParseInt provides two main features: parsing and validation.
The process of translating an input’s outward format (such as a string) into its internal representation (such as an integer) is known as parsing.
Making sure that the input complies with a certain format or set of guidelines is known as validation.
Numerous input formats, including dates, integers, URLs, email addresses, and more, can be parsed using ParseInt.
Additionally, it can be used to check input against a predetermined format or rules. ParseInt, for instance, can be used to verify the validity of an email address before sending an email to it.
A straightforward API that is simple to understand is used for parsing and validation. The two primary functions in the API are validate() and parse(). Since any Java code can invoke these methods, parseInt is simple to incorporate into already-existing applications.
The java.lang.Integer class has the parseInt() method. Because it’s static, you can call it without first constructing an Integer object.
Using parseInt in Java
Calling parseInt only requires passing an integer’s string representation as an argument:
static int parseInt(String s)
It might occasionally be necessary to parse an integer’s string representation in a different number base, such as binary, octal, or hexadecimal.
In order to accomplish this, you can use the following syntax to supply an extra argument to parseInt that specifies the number’s radix (base):
static int parseInt(String s, int radix)
The parseInt() method requires a string that only contains numeric characters. An exception will be raised and the conversion will not happen if the string contains any non-numeric characters.
Advantages of ParseInt
Thus, what would make using parseInt() desirable? Check out a few of the advantages listed below.
Simplicity
The simple method SimpleParseInt accepts a string as an input and returns the corresponding integer value. It makes the conversion process simpler and more efficient by doing away with the requirement for manual string parsing.
Robustness
The underlying complexity of converting strings to integers, such as managing several number bases, monitoring for overflow, and identifying erroneous characters, is automatically taken care of by ParseInt. As a result, there is less chance of errors, and your code is more reliable.
Consistency
ParseInt converts text to integers using a standard method, ensuring consistent output across many Java platforms and programs. Your code becomes easier to maintain and more portable as a result.
Exception Handling
A NumberFormatException will be raised if the string that is supplied to parseInt is not a valid representation of an integer. This allows you to use a try-catch block or examine the input before executing parseInt, which makes it easier to find and fix issues in your code.
Java ParseInt in Action
Assume you run a website where visitors can enter their age. The user’s input could be transformed into an integer using the parseInt function so that it can be kept in a database.
The user’s age may then be obtained from the database by using the parseInt function to transform it back into a text string.
Take an age verification form seen on an alcohol website, for instance. This function can be used to store and retrieve data from your back end.
When using parseInt in Java, there are a few considerations to make.
Firstly, parseInt will return an error if the text string cannot be transformed into a valid integer.
Second, when the string is converted to an integer, leading and trailing whitespace characters are ignored.
Lastly, the string will be processed as a hexadecimal number if it begins with 0x or -0x.
The parseInt method of the Integer class can be used, for example, if you need to parse an int from a string in Java. This function returns an int and accepts a String as an input. Here’s an easy illustration:
String str = “123”; int i = Integer.parseInt(str); System.out.println(i); // Prints 123
You’ll receive an exception if the String you’re attempting to parse isn’t a valid int. As an illustration:
String str = “abc”; int i = Integer.parseInt(str); // Throws NumberFormatException
How to Use ParseInt in Java
Examining a Java parseInt example is the best method to comprehend the idea and implement it correctly.
Here’s an illustration of how to convert a string to an integer in Java using parseInt:
String str = “123”; int number = Integer.parseInt(str); System.out.println(“The integer value is: ” + number);
The parseInt function of the Integer class receives the string “123” as an input in this example, and it transforms the string into the integer value 123 and stores it in the number variable. “The integer value is: 123” will be the ultimate result.
Take a look at the code that publishes the square of a given integer after asking the user to enter it:
import java.util.Scanner; public class Main { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print(“Enter a number: “); String input = scanner.nextLine(); int number = Integer.parseInt(input); int square = number * number; System.out.println(“The square of ” + number + ” is ” + square); } }
The user’s input is read as a string in this example using the Scanner class. After the input string has been converted to an integer and placed in the number variable, the parseInt method is called on it. Subsequently, the square is computed using the number variable and output to the console.
Instructions to Use ParseInt
Here are some detailed instructions for assembling the parseInt Java library.
1.Import the java.lang package
You must import the java.lang package, which contains the Integer class, in order to use the parseInt method. To accomplish this, append the subsequent line to the beginning of your Java file:
import java.lang.*;
2.Convert a string to an integer.
You can use the parseInt method of the Integer class and supply the string you wish to convert as an argument to convert a string to an integer. The equivalent integer value will be returned by the parseInt method. As an illustration:
String str = “123”; int number = Integer.parseInt(str); System.out.println(“The integer value is: ” + number);
3.Get output.
The parseInt function of the Integer class receives the string “123” as an input in this example, and it transforms the string into the integer value 123 and stores it in the number variable. “The integer value is: 123” will be the ultimate result.
It’s crucial to keep in mind that a NumberFormatException will be raised if the text supplied to parseInt is not a valid integer representation. You can examine the input before calling parseInt, or you can use a try-catch block to handle this exception.
Conclusion When transforming integer string representations into real integer numbers, Java’s parseInt function offers a number of advantages. This Java technique for converting texts to integers is straightforward, reliable, and consistent, making it an essential tool for developers who need to handle numerical data in their programs. Check out the Java free online course to learn more.