Life Cycle of React Component
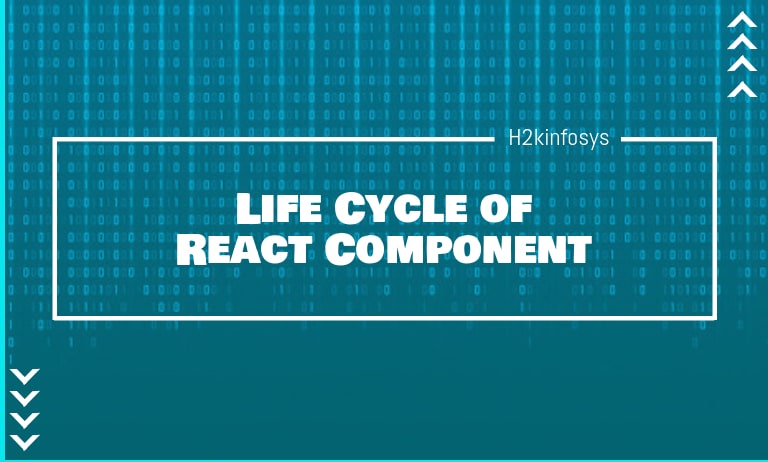
In ReactJS, the creation of every component involves various lifecycle methods. These lifecycle methods are known as the component’s lifecycle. These methods are not very complicated and are called at various points during a component’s life. The lifecycle of a component is divided into four phases:
- Initialization: This is the first stage where the component is constructed with the provided Props and default state and is done in the constructor of a Component Class.
- Mounting: This is the stage of rendering the JSX returned by the render method itself.
- Updating: This is the stage when the state of a component is updated, and the application is repainted.
- Unmounting: As the name suggests, this is the final step of the component lifecycle where the component is removed from the page.
1. Initialization Phase:
It is the birth phase of the lifecycle of a ReactJS component. Here, the component starts its journey on the way to the DOM. In this stage, a component contains the default Props and initial State, and these default properties are defined in the constructor of a component.
class Initialize extends React.Component { constructor(props) { // Calling the constructor of // Parent Class React.Component super(props); // initialization process this.state = { date : new Date(), clickedStatus: false }; }
The initial phase occurs only once and consists of the following methods:
- getDefaultProps(): This is used to specify the default value of this.props. It is invoked before creating the component, or any props from the parent is passed into it.
- getInitialState(): This is used to specify the default value of this.state and is invoked before the creation of the component.
2. Mounting Phase:
In this stage, the instance of a component is created and inserted into the DOM. It contains the following methods:
- componentWillMount(): It is called immediately before a component gets rendered into the DOM. Here, when you call setState() inside this method, the component will not re-render.
- componentDidMount(): This method is called immediately after a component gets rendered and placed on the DOM, and you can do any DOM querying operations.
- render(): This method is defined in each component and is responsible for returning a single root HTML node element. If you don’t want to display anything, you can return a null or false value.
class LifeCycle extends React.Component { componentWillMount() { console.log('Component will mount!') } componentDidMount() { console.log('Component did mount!') this.getList(); } getList=()=>{ /*** method to make api call*** } render() { return ( <div> <h3>Hello mounting methods!</h3> </div> ); } }
3. Updating Phase:
It is the next stage of the lifecycle of a react component. In this stage, we get new Props and change State. This phase also allows us to handle user interaction and provide communication with the components hierarchy. This phase aims to ensure that the component is displaying the latest version of itself. This phase repeats again and again. This phase contains the following methods:
- componentWillRecieveProps(): This is invoked when a component receives new props. If you want to update the state in response to the prop changes, you should compare this.props and nextProps to perform state transition by using this.setState() method.
- shouldComponentUpdate(): This is invoked when a component decides any changes/updates to the DOM and allows you to control the behavior of component’s updating itself. If the method returns true, the component will update. Otherwise, it will skip updating.
- componentWillUpdate(): This is invoked just before the component updating occurs, and you can not change the component state by invoking this.setState() method. It will not be invoked if the method shouldComponentUpdate() returns false.
- render(): This is invoked to examine this.props and this.state, and it returns one of these types: React component elements, Arrays and fragments, Booleans or null, String and Number. If the method shouldComponentUpdate() returns false, the code inside render() will be called again to ensure that the component displays itself properly.
- componentDidUpdate(): This is invoked immediately after the component updating occurs. Here, you can put any code inside this you want to execute once the updating occurs, and it is not called for the initial render.
class LifeCycle extends React.Component { constructor(props) { super(props); this.state = { date : new Date(), clickedStatus: false, list:[] }; } componentWillMount() { console.log('Component will mount!') } componentDidMount() { console.log('Component did mount!') this.getList(); } getList=()=>{ /*** method to make api call*** fetch('https://api.mydomain.com') .then(response => response.json()) .then(data => this.setState({ list:data })); } shouldComponentUpdate(nextProps, nextState){ return this.state.list!==nextState.list } componentWillUpdate(nextProps, nextState) { console.log('Component will update!'); } componentDidUpdate(prevProps, prevState) { console.log('Component did update!') } render() { return ( <div> <h3>Hello Mounting Lifecycle Methods!</h3> </div> ); } }
4. Unmounting Phase:
It is the last stage of the react component lifecycle. It is invoked when a component instance is destroyed and unmounted from the DOM. This phase contains only one method:
- componentWillUnmount(): This method is called immediately before a component is destroyed and unmounted permanently. It performs any necessary cleanup related tasks such as invalidating timers, event listener, canceling network requests, or cleaning up DOM elements. If a component instance is unmounted, you cannot mount it again.
import React, { Component } from 'react'; class App extends React.Component { constructor(props) { super(props); this.state = {hello: "hi"}; this.changeState = this.changeState.bind(this) } render() { return ( <div> <h1>ReactJS component's Lifecycle</h1> <h3>Hello {this.state.hello}</h3> <button onClick = {this.changeState}>Click Here!</button> </div> ); } componentWillMount() { console.log('Component Will MOUNT!') } componentDidMount() { console.log('Component Did MOUNT!') } changeState(){ this.setState({hello:"All!!- Its a great reactjs tutorial."}); } componentWillReceiveProps(newProps) { console.log('Component Will Recieve Props!') } shouldComponentUpdate(newProps, newState) { return true; } componentWillUpdate(nextProps, nextState) { console.log('Component Will UPDATE!'); } componentDidUpdate(prevProps, prevState) { console.log('Component Did UPDATE!') } componentWillUnmount() { console.log('Component Will UNMOUNT!') } } export default App;
Output:
When you click on the button Click Here, you get the updated result shown in the below screen.
One Comment