Introduction
In the world of web automation, Selenium WebDriver is one of the most popular tools for automating browsers. It’s a powerful solution for testing web applications and simulating user interactions with web elements. Among the various challenges that automation testers face, working with elements like the DatePicker in Selenium is particularly tricky.
A DatePicker is a graphical interface element that allows users to select dates, often found in forms and online booking systems. Automating this process in Selenium WebDriver can be challenging, but with the right approach, it becomes a seamless task. In this blog post, we’ll delve into how to select dates from a DatePicker in Selenium, provide a step-by-step guide, and cover common pitfalls you should avoid. Additionally, we’ll discuss the importance of learning automation testing techniques like these in real-world projects.
Why Learn Selenium for Automation Testing?
Before diving into the technicalities of working with the DatePicker in Selenium, let’s take a moment to understand why learning Selenium is an essential skill for today’s QA professionals. Selenium allows testers to automate repetitive tasks, execute complex scenarios, and increase test coverage all while reducing the human effort required for testing.
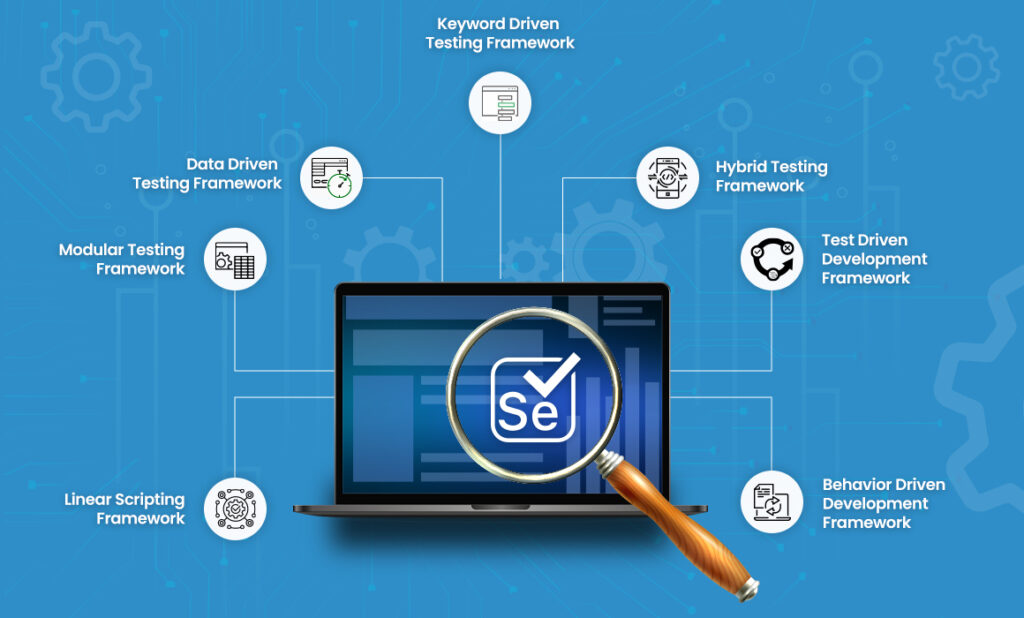
With Selenium, testers can:
- Automate web applications across different browsers.
- Improve testing efficiency with minimal code.
- Perform cross-browser testing with ease.
- Integrate with other tools like TestNG and JUnit for better reporting and test management.
If you’re looking to enhance your career in automation testing, enrolling in Selenium course is a great first step. Training programs like those offered by H2K Infosys can help you gain the hands-on skills needed to navigate real-world testing challenges, including working with elements like the DatePicker.
Understanding the DatePicker in Selenium
A DatePicker in Selenium is a UI component that allows users to select a specific date from a calendar pop-up. These DatePicker in Selenium is commonly used in forms for booking flights, events, or even setting reminders. They might appear as simple text fields or in the form of an interactive calendar with previous and next arrows.
Automating the selection of a date in Selenium requires a deep understanding of how the DatePicker works and how to interact with it. The challenge arises because the DatePicker is often a combination of dynamic HTML elements, making it more complicated than simple form fields.
Types of DatePickers
Before we dive into the code, let’s explore the different types of DatePickers you may encounter in Selenium:
- Basic DatePicker: This is a simple calendar interface where the user can select a date. The DatePicker can either be a text box where the user enters the date manually, or it may pop up when the user clicks on a calendar icon.
- Date Picker with Dropdowns: This type of DatePicker uses dropdown lists for day, month, and year selection, rather than a graphical calendar interface.
- Date Picker with Date Range: In some cases, you may need to select a start and end date from the same DatePicker, such as when booking a hotel or event. This requires selecting a date range instead of just a single date.
In the following sections, we will walk through the steps to automate DatePicker interactions using Selenium WebDriver.
Selecting Date from DatePicker in Selenium WebDriver: Step-by-Step Guide
Step 1: Launch the Browser and Navigate to the Page Containing the DatePicker
First, you’ll need to initialize your WebDriver and navigate to the page where the DatePicker is located. Let’s assume you are testing an application where users need to select a travel date from a DatePicker on the booking page.
java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class DatePickerAutomation {
public static void main(String[] args) {
// Set the path for the WebDriver executable
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// Initialize the WebDriver and open the browser
WebDriver driver = new ChromeDriver();
// Navigate to the page with the DatePicker
driver.get("https://example.com/booking");
}
}
Step 2: Locate the DatePicker Element
You will need to locate the DatePicker element on the web page using one of the various Selenium locator strategies like id
, name
, xpath
, or cssSelector
. Here’s an example of how to locate a DatePicker using XPath:
java
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
WebElement datePicker = driver.findElement(By.xpath("//input[@id='date-picker']"));
Step 3: Interact with the DatePicker
After identifying the DatePicker, the next task is to interact with it. In many cases, clicking the input field will trigger a calendar to pop up. You can automate this by clicking on the DatePicker element:
java
datePicker.click();
Step 4: Select a Date
Once the calendar is open, the next step is selecting a date. To achieve this, you need to identify the day of the month and interact with it. You can use XPath to locate the date in the calendar and click on it.
java
// Example for selecting a specific date (e.g., 15th of the month)
WebElement day = driver.findElement(By.xpath("//a[text()='15']"));
day.click();
Step 5: Verify Date Selection
After selecting the date, it’s important to verify that the correct date has been selected. You can retrieve the value from the input field and compare it with the expected date.
java
String selectedDate = datePicker.getAttribute("value");
System.out.println("Selected Date: " + selectedDate);
Step 6: Handle Dynamic Elements and Date Ranges
Many modern DatePicker in Selenium are dynamic, meaning the calendar will adjust the displayed month when you click on the “Next” or “Previous” buttons. In such cases, you will need to handle these dynamic elements by checking if the target date exists and, if not, navigating through the calendar to the correct month.
java
WebElement nextButton = driver.findElement(By.xpath("//span[@class='next']"));
nextButton.click(); // Click to go to the next month
Best Practices for Working with DatePickers in Selenium
While selecting a date from a DatePicker in Selenium may seem straightforward, there are several best practices to keep in mind to ensure your test scripts are reliable and efficient. Implementing these best practices helps ensure your scripts are robust and adaptable to changes in the web application. Below are some key strategies to optimize your Selenium automation when working with DatePicker in Selenium
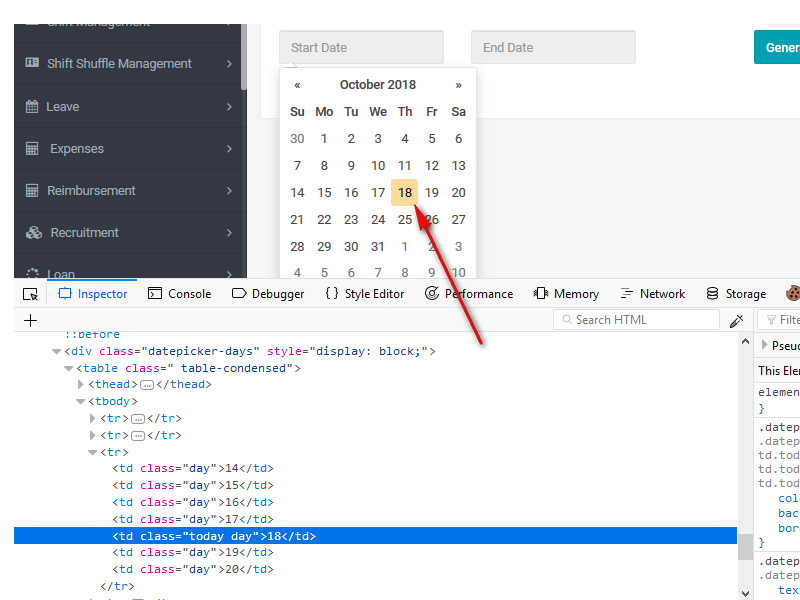
Use Explicit Waits: In Selenium, it’s essential to use explicit waits to wait for elements to load or become clickable before interacting with them. This is particularly important for DatePicker in Selenium, which can take time to load or appear dynamically after an action. Instead of using implicit waits or Thread.sleep(), which can be unreliable and inefficient, use WebDriverWait
to wait until the DatePicker element or its components (such as the calendar or next/previous buttons) are clickable.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement datePickerInput = wait.until(ExpectedConditions.elementToBeClickable(By.id("date-picker")));
datePickerInput.click();
Handle Dynamic Elements with Precision: Many modern web applications use dynamic DatePickers that adjust based on user input. For example, the calendar may change based on the selected month or year, requiring interaction with the navigation buttons (such as “next” or “previous”). Make sure your test accounts for these changes by dynamically locating the next/previous month buttons and performing actions like clicking on them if the desired date is not visible
WebElement nextMonthButton = driver.findElement(By.xpath("//span[@class='next']"));
nextMonthButton.click(); // Navigate to the next month if the desired date is not visible
Use Robust Locators: When selecting a date from a DatePicker, make sure you’re using strong and reliable locators. While XPath and CSS selectors are common, it’s important to choose locators that won’t break easily due to changes in the web application’s structure. For example, using locators based on stable attributes (such as id
, class
, or name
) ensures your script is less prone to breaking when the DOM structure changes.
Verify Date Format Consistency: Date formats can vary across applications, such as using dd/MM/yyyy
or MM/dd/yyyy
. Ensure that your Automation script selects the correct date format according to the application’s requirements. Also, when working with DatePickers, it’s essential to validate that the selected date is correctly displayed in the input field after it is chosen.
String selectedDate = datePickerInput.getAttribute("value");
assert selectedDate.equals("15/01/2024"); // Verify that the date format matches the expected format
Test Multiple Date Scenarios: A DatePicker may have additional functionality, such as the ability to select a range of dates (start and end dates) or pick a date from a disabled calendar. It’s important to test these edge cases. Test scenarios where the selected date is part of a range, ensure that the calendar doesn’t allow selection of past dates (if that’s the desired behavior), and handle months and years that may not be immediately visible.
Conclusion
Automating the selection of dates from DatePicker in Selenium WebDriver requires a combination of patience, attention to detail, and an understanding of how dynamic web elements work. By following the steps and best practices outlined in this guide, you’ll be well on your way to becoming proficient at handling DatePickers in your Selenium tests.
As you continue to explore the world of Selenium automation testing, remember that the real-world applications of these skills are vast. Whether you’re automating a simple form submission or testing complex online booking systems, knowing how to work with DatePickers in Selenium will be a valuable asset in your testing toolkit.
If you’re eager to learn more and apply these skills in real-world projects, enrolling in online Selenium training can provide you with the hands-on experience you need to succeed in automation testing. H2K Infosys offers comprehensive Selenium certification courses that will equip you with the skills necessary to excel in the field.
Key Takeaways
- Selenium WebDriver provides powerful tools to interact with DatePickers in automated tests.
- Handling dynamic and complex DatePickers requires understanding of WebDriverWait and navigation techniques.
- Learning Selenium through online training and earning a Selenium certification can significantly enhance your testing career.
Ready to level up your Selenium skills? Enroll in H2K Infosys’ Selenium Automation Testing Course today and start mastering the tools that will set you apart in the industry!
2 Responses
Are you planning to attend digital marketing interviews? Worrying about how to prepare for the interview, here is the list of top Digital Marketing Interview Questions and Answers.
Thanks for posting this info.