Introduction
Imagine releasing a web application without testing. Bugs slip through. Customers get frustrated. Your brand suffers. That’s where automated testing steps in faster, repeatable, and reliable.
Selenium with TestNG is one of the most powerful combinations in automation testing today. While Selenium handles browser interactions, TestNG brings structure, data handling, and reporting power. For professionals pursuing a Selenium certification or enrolling in a Selenium course, understanding this integration is a foundational skill.
In this guide, we’ll walk you through everything a beginner needs to know from setup to execution. Whether you’re just starting or preparing for job-ready automation testing, this blog is your step-by-step learning path.
What is Selenium?
Selenium is an open-source test automation tool used for automating web applications. It simulates user actions like clicking, typing, and navigating between pages just like a real user would.
Key Benefits of Selenium
- Supports Multiple Languages: Java, Python, C#, Ruby, and more.
- Cross-Browser Testing: Compatible with Chrome, Firefox, Edge, and Safari.
- Platform Independent: Runs on Windows, macOS, and Linux.
- Highly Extensible: Integrates with tools like TestNG, Maven, Jenkins, etc.
- Large Community: Widely used with active contributions and support.
What is TestNG?
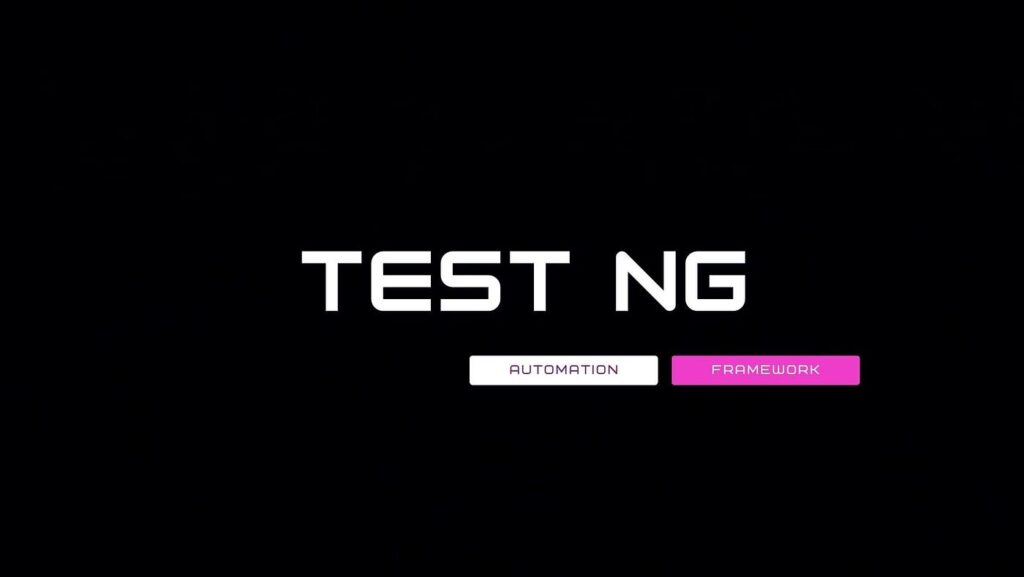
TestNG stands for “Test Next Generation.” It’s a testing framework inspired by JUnit but with added features to make automation testing more flexible and powerful.
Why Use TestNG with Selenium?
Selenium alone doesn’t manage test cases, dependencies, or reporting. TestNG fills these gaps by allowing:
- Test execution in a structured format.
- Grouping and prioritization of tests.
- Parallel test execution.
- Generating readable test reports.
- Data-driven testing using
@DataProvider
.
Real-World Use Case: E-Commerce Website
Let’s say you’re automating an e-commerce site. You want to test:
- Login functionality.
- Product search.
- Adding items to the cart.
- Checkout process.
Using Selenium with TestNG, you can:
- Create individual test methods for each action.
- Group related tests (like “Smoke Tests” or “Regression Tests”).
- Run them sequentially or in parallel across browsers.
- Generate a test report with success/failure results.
Setting Up Selenium with TestNG
Let’s break it down into practical steps.
Step 1: Install Java JDK
Before everything, make sure Java is installed on your system. Selenium and TestNG are Java-based, so this is a must.
Step 2: Install Eclipse IDE
Eclipse is a popular IDE for Selenium and Java. After installation:
- Launch Eclipse.
- Set up a new workspace.
- Create a Java project.
Step 3: Add Selenium JARs
Download Selenium WebDriver JAR files and add them to your project’s build path:
- selenium-java.jar
- selenium-server.jar
- client-combined-x.x.x.jar
Step 4: Install TestNG in Eclipse
Go to Help > Eclipse Marketplace
, search for “TestNG”, and install it. After restarting Eclipse, right-click your project and select “Convert to TestNG.”
Step 5: Basic Selenium with TestNG Script
java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class DemoTest {
@Test
public void openGoogle() {
WebDriver driver = new ChromeDriver();
driver.get("https://www.google.com");
driver.quit();
}
}
This script opens Google using Chrome browser and then closes it.
Understanding TestNG Annotations
TestNG annotations control how and when methods are executed.
Annotation | Purpose |
---|---|
@BeforeSuite | Runs once before all tests in the suite |
@BeforeTest | Runs before any test case |
@BeforeClass | Runs before the first test method |
@BeforeMethod | Runs before every @Test method |
@Test | Marks a method as a test case |
@AfterMethod | Runs after every @Test method |
@AfterClass | Runs after all test methods |
@AfterTest | Runs after all test cases |
@AfterSuite | Runs once after all tests are done |
TestNG XML Configuration
TestNG uses an XML file to define test suites and execution parameters.
Sample testng.xml
xml
<!DOCTYPE suite SYSTEM "https://testng.org/testng-1.0.dtd" >
<suite name="Demo Suite">
<test name="Demo Test">
<classes>
<class name="DemoTest"/>
</classes>
</test>
</suite>
This file:
- Defines a suite.
- Includes one test class.
- Can be expanded to include more tests, parallel executions, and groups.
Running Tests in Parallel
Parallel execution reduces total testing time. Update the XML:
xml
<suite name="Parallel Suite" parallel="tests" thread-count="2">
<test name="Test1">
<classes>
<class name="TestClass1"/>
</classes>
</test>
<test name="Test2">
<classes>
<class name="TestClass2"/>
</classes>
</test>
</suite>
TestNG will now run TestClass1
and TestClass2
simultaneously.
Data-Driven Testing Using @DataProvider
When you want to test the same functionality with different data sets, use @DataProvider
.
Example:
java
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
public class LoginTest {
@DataProvider(name = "loginData")
public Object[][] getData() {
return new Object[][] {
{"user1", "pass1"},
{"user2", "pass2"},
{"user3", "pass3"}
};
}
@Test(dataProvider = "loginData")
public void loginTest(String username, String password) {
System.out.println("Login with: " + username + " / " + password);
// Add Selenium code here
}
}
Each set of credentials will run the login test separately.
Benefits of Learning Selenium with TestNG
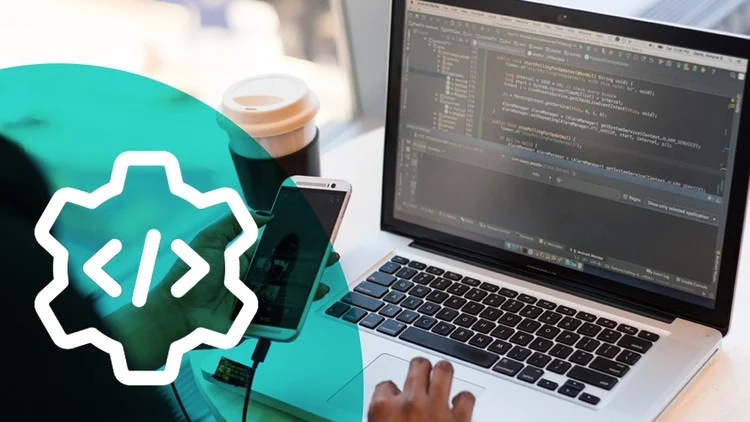
Job-Readiness
Most automation testing roles require knowledge of Selenium with TestNG. Learning this combo can significantly boost your job opportunities.
Better Test Management
Using TestNG makes it easier to manage hundreds of test cases. You can group tests, prioritize them, and control their execution flow.
Clean and Maintainable Code
TestNG encourages structured and clean code, which is easier to read, maintain, and debug.
Selenium Certification Preparation
If you’re pursuing a Selenium certification, learning TestNG will help you handle questions on frameworks, annotations, and test design.
Industry Trends & Stats
- 90% of companies using Selenium adopt TestNG as their preferred framework.
- 80% of Selenium job descriptions require hands-on experience with TestNG.
- Organizations like Amazon, Capgemini, and Cognizant integrate TestNG into their Selenium pipelines.
Common Challenges (and How to Solve Them)
Challenge | Solution |
---|---|
Test flakiness | Use @BeforeMethod and @AfterMethod for setup/cleanup |
Long test execution times | Enable parallel execution |
Handling multiple test data | Use @DataProvider |
Poor test reports | Use built-in TestNG HTML reports |
Real-World Test Case: Automating Login Functionality
Here’s how you might test a login screen:
java
@Test
public void loginToApp() {
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/login");
driver.findElement(By.id("username")).sendKeys("testuser");
driver.findElement(By.id("password")).sendKeys("testpass");
driver.findElement(By.id("loginButton")).click();
String expectedUrl = "https://example.com/dashboard";
Assert.assertEquals(driver.getCurrentUrl(), expectedUrl);
driver.quit();
}
This test:
- Navigates to login page.
- Enters credentials.
- Clicks the login button.
- Verifies redirection to dashboard.
Best Practices for Selenium with TestNG
- Always use
@BeforeMethod
for driver setup and@AfterMethod
for teardown. - Avoid hard-coded waits; use explicit waits instead.
- Name your tests clearly and consistently.
- Group similar tests together in XML.
- Store test data separately (e.g., Excel, CSV, database).
Key Takeaways
- Selenium with TestNG offers a robust solution for browser automation and test management.
- It supports structured execution, parallel testing, and detailed reporting.
- Learning this combination is essential for automation testers and those pursuing a Selenium certification.
- Through hands-on projects, you’ll gain real-world skills that can boost your career.
Conclusion
If you’re serious about becoming an expert in automation testing, mastering Selenium with TestNG is a non-negotiable step. It’s the bridge between manual testing and CI/CD-enabled automation pipelines.
Ready to take the next step?
Join H2K Infosys’ hands-on Selenium course today and become a job-ready automation testing professional.