What is Public/Private Key Security?
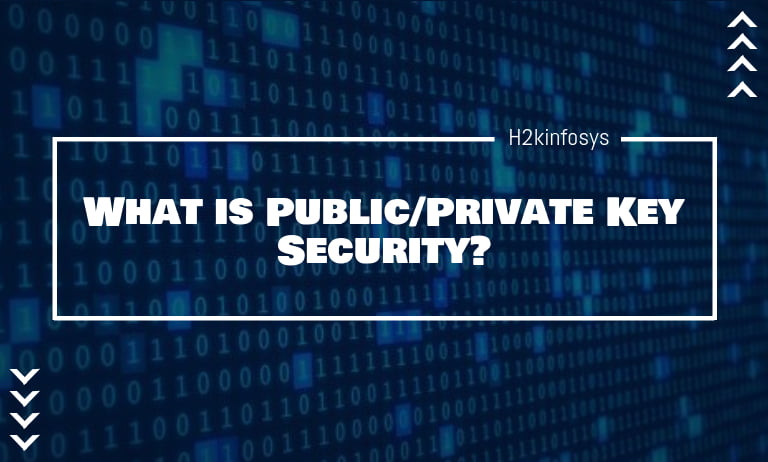
Encryption technologies are used to secure data. Passing the confidential data over the network may create Public/Private Key security issues. Thus, encrypting the data before passing through the network and decrypting it afterward can overcome these security issues.
The process of converting plain text into the cipher text is called Encryption, whereas the process of restoring cipher text into the plain text is called Decryption.
There are two types of Encryption:
- Symmetric Key Encryption: It means using the same key to both encrypt and decrypt the message. The key used is called a secret key, as it is known to only two entities (sender and receiver).
- Asymmetric Key Encryption: It uses two different keys, one to encrypt the message and other to decrypt the message. A public key encrypts the message that is distributed freely. A private key is used to decrypt the message, which is only known to its owner.
Encryption is important to Web Service security because SOAP messages are plain text by default. There are various ways to implement asymmetric encryption are AES (Advanced Encryption Standard), RSA (Rivest, Shamir, Adleman), IDEA (International Data Encryption Algorithm), etc.
Here, we are going to implement asymmetric encryption using RSA.
- For this, you first need to generate Public/Private Key security using the SecureRandom class, which is used to generate a random number.
SecureRandom random = new SecureRandom();
- After this, the KeyGenerator class will provide a getInstance() method that can be used to pass a string variable that denotes the Key Generation Algorithm. It returns the KeyGenerator Object.
KeyPairGenerator KPGenerator = KeyPairGenerator.getInstance(KeyGeneration_Algorithm_string_variable);
- Last, we will initialize the keyGenerator object with 2048 bits key size and pass the random number.
keyPairGenerator.initialize(2048, secureRandom);
Now, the secret key is generated, and to see the generated key, which is an object, we can convert it into a hex binary format using DatatypeConverter.
Below is the code implementation of above approach:
package javacryptography; import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.SecureRandom; import javax.xml.bind.DatatypeConverter; public class Asymmetric { private static final String RSA = "RSA"; public static KeyPair generateRSAKkeyPair() throws Exception { SecureRandom secureRandom = new SecureRandom(); KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance(RSA); keyPairGenerator.initialize(2048, secureRandom); return keyPairGenerator.generateKeyPair(); } public static void main(String arg[]) throws Exception { KeyPair keypair = generateRSAKkeyPair(); System.out.println("Public Key is: " + DatatypeConverter.printHexBinary( keypair.getPublic().getEncoded())); System.out.println("Private Key is: " + DatatypeConverter.printHexBinary( keypair.getPrivate().getEncoded())); } }
Output:
In the above code, we have generated the private key and public key. Now, we will implement the encryption and decryption methods.
- The cipher class is used for two modes, that is the encryption and decryption. As Asymmetric encryption uses two different keys, we will use the private key for encryption and the public key for decryption.
cipher.init(Cipher.ENCRYPT_MODE, privateKey);
cipher.init(Cipher.DECRYPT_MODE, publicKey);
- The doFinal() method is invoked on the cipher, which then encrypts/decrypts data in a single-part operation, or can finish a multiple-part operation and returns it in a byte array.
- Finally, we will get the Cipher text after Encryption with ENCRYPT_MODE.
Below is the code implementation of above approach:
package javacryptography; import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.PrivateKey; import java.security.PublicKey; import java.security.SecureRandom; import java.util.Scanner; import javax.crypto.Cipher; import javax.xml.bind.DatatypeConverter; public class Asymmetric { private static final String RSA = "RSA"; private static Scanner sc; public static KeyPair generateRSAKkeyPair() throws Exception { SecureRandom secureRandom = new SecureRandom(); KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance(RSA); keyPairGenerator.initialize(2048, secureRandom); return keyPairGenerator.generateKeyPair(); } public static byte[] do_RSAEncryption(String plainText, PrivateKey private Key) throws Exception { Cipher cipher = Cipher.getInstance(RSA); cipher.init(Cipher.ENCRYPT_MODE, private Key); return cipher.doFinal(plainText.getBytes()); } public static String do_RSADecryption(byte[] cipherText, PublicKey public Key) throws Exception { Cipher cipher = Cipher.getInstance(RSA); cipher.init(Cipher.DECRYPT_MODE, public Key); byte[] result = cipher.doFinal(cipherText); return new String(result); } public static void main(String arg[]) throws Exception { KeyPair keypair = generateRSAKkeyPair(); String plainText = "This is the PlainText " + "I want to Encrypt using RSA."; byte[] cipherText = do_RSAEncryption(plainText, keypair.getPrivate()); System.out.println("The Public Key is: " + DatatypeConverter.printHexBinary( keypair.getPublic().getEncoded())); System.out.println("The Private Key is: " + DatatypeConverter.printHexBinary( keypair.getPrivate().getEncoded())); System.out.print("The Encrypted Text is: "); System.out.println(DatatypeConverter.printHexBinary(cipherText)); String decryptedText = do_RSADecryption(cipherText, keypair.getPublic()); System.out.println("The decrypted text is: " + decryptedText); } }
Output: