Exception handling
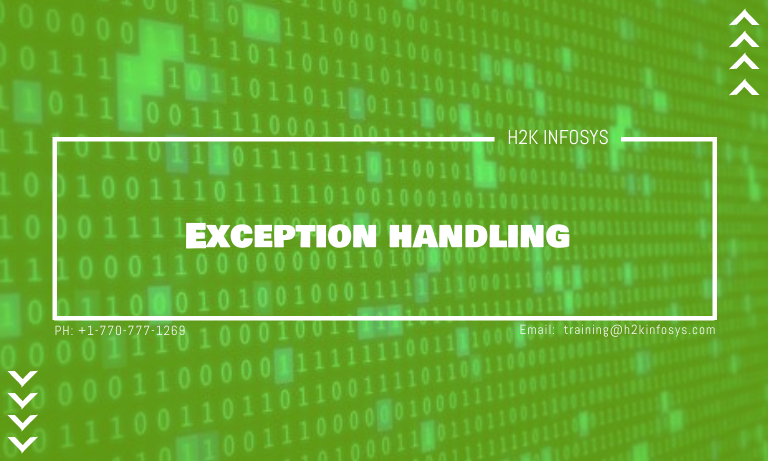
Basically exception handling in the Java can be defined as an object which will describe an exceptional condition which has been occurred in a piece of code. When an exception condition arises object representing that exception will be created and thrown in the method which will cause the error.
Java exceptional handling will be managed by the five keywords which includes: try, catch, throw, throws and finally.
Program statements which we want to monitor for the exception will be contained within the try block, if an exception occurs within try block it is thrown.
Our code can catch exception and handle some it in some of the rational manner, syntax of exception handling is:
Try { // block of code which is to monitor errors } Catch(exceptiontype1 exOb) { // exception handler for exceptiontype1 } Catch (exceptiontype2 exOb) { //exception handler for exceptiontype2 } //…… Finally } //block of code which is to be executed } In this exception type will be the type of exception which has occurred.
Exception types
In this exception types are subclass of the built-in class throwable, this is at the top of the exception class. Throwable is been classified as exception and error.
throwable
exception error
Using try and catch:
Default exception handler is provided by the run time system. It is useful for the debugging the program in which exception is handled. This will provide two benefits: first- it will fix the errors and second- it will prevent the program which is stopped running and print a stack trace whenever an error will be occurred
Let us see an example for this which process arithmetic exception generated by division by zero
Class Exc2 { Public static void main(string args[]) { Int d,a; Try { d = 0; A = 42 / d; System.out.println(“this will not be printed”); } catch (arithmeticexceptoin e) { System.out.println(“division by zero”); } System.out.println(“after catch statement”); } }
Output
Division by zero
After catch statement
Once the exception is been thrown, program will control the transfers out of the try block into the catch block. Catch is also not called so the execution never returns to the try block from catch thus this line will not be printed. Once the catch statement is executed program control continues with next line in the program which follows entire try and catch mechanism.
Try and catch statement will form a unit. Scope of the catch clause will be restricted to those statements which is specified by the preceding try statement. Catch statement will not catch an exception thrown by another try statement.
Let us see another program where each iteration of the for loop will obtain two random integers these will be divided by each other and result is used to divide the value 12345
Import java. util. Random; Public static void main(string args []) { Int a=0, b=0, c=0; Random r = new Random (); For ( int i=0; i<32000; i++ ) { Try { B=r.nextint(); C=r.nextint(); A=12345/ (b/c); System.out.println(“division by zero”); A=0; } System.out.println(“a “+ a); } } }
Questions:
- What is exception handling?
- With program explain catch and try?
1.Exception handling in the Java can be defined as an object which will describe an exceptional condition which has been occurred in a piece of code. When an exception condition arises object representing that exception will be created and thrown in the method which will cause the error.
Java exceptional handling will be managed by the five keywords which includes: try, catch, throw, throws and finally.
Program statements which we want to monitor for the exception will be contained within the try block, if an exception occurs within try block it is thrown.
2.Once the exception is been thrown, program will control the transfers out of the try block into the catch block.
Try and catch statement will form a unit. Scope of the catch clause will be restricted to those statements which is specified by the preceding try statement. Catch statement will not catch an exception thrown by another try statement.
Default exception handler is provided by the run time system. It is useful for the debugging the program in which exception is handled. This will provide two benefits: first- it will fix the errors and second- it will prevent the program which is stopped running and print a stack trace whenever an error will be occurred
Output
Division by zero
After catch statement
Once the exception is been thrown, program will control the transfers out of the try block into the catch block. Catch is also not called so the execution never returns to the try block from catch thus this line will not be printed. Once the catch statement is executed program control continues with next line in the program which follows entire try and catch mechanism.
Let us see an example for this which process arithmetic exception generated by division by zero
Exception handling in the Java can be defined as an object which will describe an exceptional condition which has been occurred in a piece of code. When an exception condition arises object representing that exception will be created and thrown in the method which will cause the error.
Java exceptional handling will be managed by the five keywords which includes: try, catch, throw, throws and finally.
Program statements which we want to monitor for the exception will be contained within the try block, if an exception occurs within try block it is thrown.
2.Once the exception is been thrown, program will control the transfers out of the try block into the catch block.
Try and catch statement will form a unit. Scope of the catch clause will be restricted to those statements which is specified by the preceding try statement. Catch statement will not catch an exception thrown by another try statement.
Default exception handler is provided by the run time system. It is useful for the debugging the program in which exception is handled. This will provide two benefits: first- it will fix the errors and second- it will prevent the program which is stopped running and print a stack trace whenever an error will be occurred
Output
Division by zero
After catch statement
Once the exception is been thrown, program will control the transfers out of the try block into the catch block. Catch is also not called so the execution never returns to the try block from catch thus this line will not be printed. Once the catch statement is executed program control continues with next line in the program which follows entire try and catch mechanism.
Let us see an example for this which process arithmetic exception generated by division by zero
1. What is exception handling?
Exception handling in the Java can be defined as an object which will describe an exceptional condition which has been occurred in a piece of code. When an exception condition arises object representing that exception will be created and thrown in the method which will cause the error.
Java exceptional handling will be managed by the five keywords which includes: try, catch, throw, throws and finally.
2. With program explain catch and try?
Default exception handler is provided by the run time system. It is useful for the debugging the program in which exception is handled. This will provide two benefits: first- it will fix the errors and second- it will prevent the program which is stopped running and print a stack trace whenever an error will be occurred
Exception handling can be defined in java as an object which will describe an exceptional condition which has been occurred in a piece of code. When an exception condition arises object representing that exception will be created and thrown in the method which will cause the error.
There are 5 keywords to manage exception handling: try ,catch, throw,throws,finally
Exception handling using try and catch
1. Default exception handler is provided by the run time system. It is useful for the debugging the program in which exception is handled. This will provide two benefits:
– it will fix the errors and second-
-it will prevent the program which is stopped running and print a stack trace whenever an error will be occurred.
2. Try and catch statement will form a unit. Scope of the catch clause will be restricted to those statements which is specified by the preceding try statement. Catch statement will not catch an exception thrown by another try statement.
Basically exception handling in the Java can be defined as an object which will describe an exceptional condition which has been occurred in a piece of code. When an exception condition arises object representing that exception will be created and thrown in the method which will cause the error.
Java exceptional handling will be managed by the five keywords which includes: try, catch, throw, throws and finally.
Exception types-:
In this exception types are subclass of the built-in class throwable, this is at the top of the exception class. Throwable is been classified as exception and error.
Using try and catch:
Once the exception is been thrown, program will control the transfers out of the try block into the catch block. Catch is also not called so the execution never returns to the try block from catch thus this line will not be printed. Once the catch statement is executed program control continues with next line in the program which follows entire try and catch mechanism.
Try and catch statement will form a unit. Default exception handler is provided by the run time system. It is useful for the debugging the program in which exception is handled. This will provide two benefits: first- it will fix the errors and second- it will prevent the program which is stopped running and print a stack trace whenever an error will be occurred
Basically exception handling in the Java can be defined as an object which will describe an exceptional condition which has been occurred in a piece of code. When an exception condition arises object representing that exception will be created and thrown in the method which will cause the error.
Java exceptional handling will be managed by the five keywords which includes: try, catch, throw, throws and finally.
Catch and Try will provide two benefits: first- it will fix the errors and second- it will prevent the program which is stopped running and print a stack trace whenever an error will be occurred
Basically exception handling in Java can be defined as an object which will describe an exceptional condition that has occurred in a piece of code. When an exception condition arises object representing that exception will be created and thrown in the method, which will cause the error.Java exceptional handling will be managed by the five keywords which include: try, catch, throw, throws, and finally.
Using try and catch:
Let us see an example for this which processes arithmetic exception generated by division by zero
Class Exc2
{
Public static void main(string args[])
{
Int d,a;
Try {
d = 0;
A = 42 / d;
System.out.println(“this will not be printed”);
} catch (arithmeticexceptoin e) {
System.out.println(“division by zero”);
}
System.out.println(“after catch statement”);
}
}
Output :
Division by zero
After catch statement
Once the exception is been thrown, the program will transfers the control out of the try block into the catch block. Catch is also not called so the execution never returns to the try block from catch thus this line will not be printed. Once the catch statement is executed program control continues with next line in the program which follows entire try and catch mechanism.
Try and catch statement will form a unit. The scope of the catch clause will be restricted to those statements which is specified by the preceding try statement. Catch statement will not catch an exception thrown by another try statement.