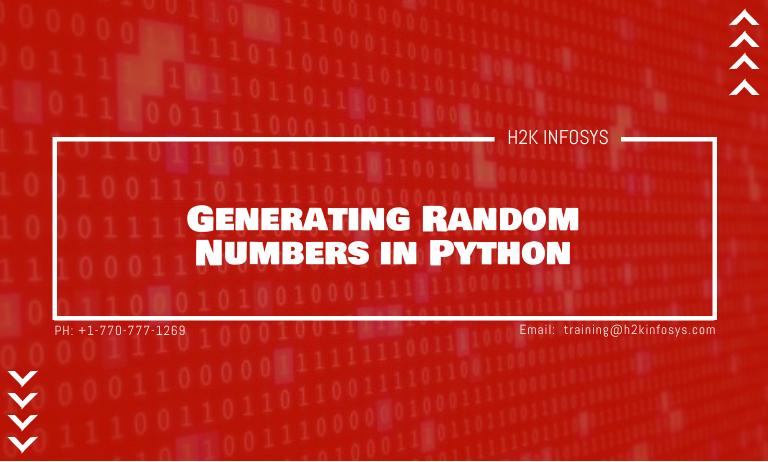
Random numbers generation has a wide range of applications in programming including cryptography, gambling, statistical sampling, etc. In Numpy, there are a plethora of methods used for generating random numbers according to your preference. In this tutorial, we will discuss how to generate random numbers in Numpy. Specifically, here is what you will learn in this tutorial:
Let’s jump right into it.
- Pseudorandom Number Generators Explained.
- Creating a Random Number in NumPy while Defining the Seed
- Creating a Randomly Generated Number that follows the Gaussian Distribution.
- Generating an Array of Random Integers
Pseudorandom Number Generators Explained: What exactly a seed is.
You may be wondering how numbers can be randomly generated. Well, they are not entirely randomly generated as a truly random sequence would involve getting the randomness from some physical entity such as a Geiger counter. In machine learning, nothing physical is used. We rather use a concept called pseudorandomness. In a pseudorandom process, the numbers are generated according to some function that is close to random. They are rather deterministic.
The sequence is generated by some functions that shuffle data based on an initialized coefficient. Calling the function returns a deterministic value (although can be seen as random) based on the initialized coefficient. In programming, the initialized coefficient is called the seed. Using the number returned as the new seed, the pseudorandom number generator is called again. This returns another deterministic value. The process continues on and on until the range of numbers is reached. There are wrapper functions that also allow the pseudo-random number generator to return integers, floating-point numbers, or numbers according to a predefined distribution.
In cases where a seed is not initialized, the pseudorandom number generator makes use of the current system time in milliseconds or seconds as the seed. Note that the seed does not really matter. Any seed used returns a random number. However, using the same seed returns the same sequence of numbers.
Defining the seed is particularly useful when you may need to run a block of code later in the future and still want to get the same output. It is also used in a classroom problem to ensure everybody arrives at a homogenous result.
Creating a Random Number in NumPy while Defining the Seed
To generate a random in Numpy, the random() function is called. The random function receives the shape of the array to be created as an argument. When no argument is passed, the function returns a single random number.
On the other hand, if you wish to initialize the seed, the seed() function is called and takes an integer as the argument. In the example below, a random number was created after defining a seed as 3.
from numpy import random #define a seed random.seed(3) #generate a random number print(random.random())
Output:
0.5507979025745755
Now, let’s create a 4 by 3 array of randomly generated numbers.
from numpy import random #define a seed random.seed(3) #generate an array random numbers print(random.random((4, 3)))
Output:
[[0.5507979 0.70814782 0.29090474]
[0.51082761 0.89294695 0.89629309]
[0.12558531 0.20724288 0.0514672 ]
[0.44080984 0.02987621 0.45683322]]
Creating a Randomly Generated Number that follows the Gaussian Distribution.
A Gaussian distribution is simply a bell-curve distribution. In other words, numbers close to the center of the distribution are more frequent than numbers in the left and right extremes. Such distributions are also called normal distributions. Generating Random Numbers that follow the normal distribution, the normal() function is called. The function takes 3 parameters: the mean, standard deviation, and the shape of the array.
Let’s create a 3 by 3 array with a mean of 2 and a standard deviation of 0.4.
from numpy import random #define a seed random.seed(3) #generate an array of random number print(random.normal(2, 0.4, (3, 3)))
Output:
[[2.71545139 2.17460394 2.03859899]
[1.25460292 1.88904472 1.85809641]
[1.96690341 1.74919973 1.98247273]]
Notice that most of the numbers are close to 2. This is because 2 was mean and a small standard deviation was selected (0.4).
Generating an Array of Random Integers
The random numbers generated up to this point were floats. If you wish to create random integers, you can use the randint() function for this purpose. The randint() function takes three arguments: the lowest number to be generated, the highest number, and the shape of the array.
If we wish to create a 4 by 3 array of random numbers between 1 and 10, the code below accomplishes that.
from numpy import random #define a seed random.seed(3) #generate an array random numbers print(random.randint(1, 10, (4, 3)))
Output:
[[9 4 9]
[9 1 6]
[4 6 8]
[7 1 5]]
Final Note
In this tutorial, you learned how to generate random numbers in Numpy. We discussed that a seed is the initialized coefficient for the pseudorandom number generator to create a random number. In your code, the seed may or may not be defined. Notice that in this example, the seed was set to 3. This means that if you run the same codes here on your PC with seed 3, the same random numbers will be created. If not, the time system of your machine in seconds is used, hence the generated number will be different every time you run the code.
If you have any questions, feel free to leave them in the comment section and I’d do my best to answer them.