Python IF, ELSE, ELIF, Nested IF & Switch Case Statement
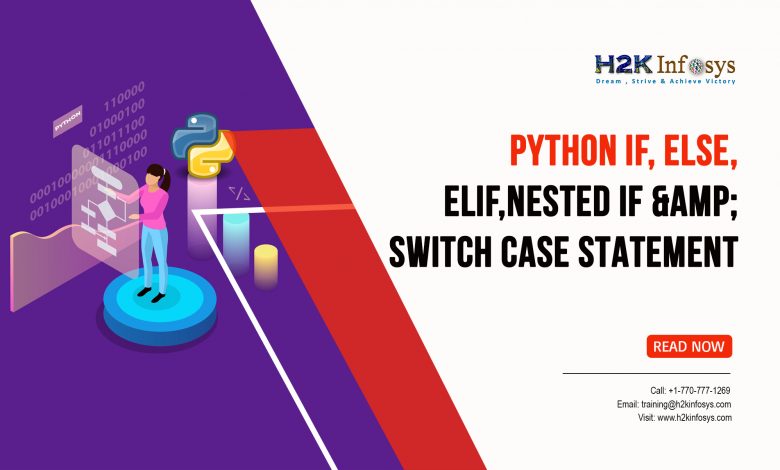
Today you will learn the concepts that are used almost in every code. Let’s just think you have been instructed to build a program for fire alarm. The fire alarm will go on when the temperature of the room goes above 50 degrees otherwise it will stay off.
IF statement
So the statement goes like this.
If the temperature is greater than 50 turn on the alarm else keep it off.
IF structure in Python
First comes if keyword then the condition. After the condition comes colon.
Temperature = 60 if temperature > 50: print("Alarm On")
Let’s code this in Python and see the output.
Else statement
Else statement always comes after IF statement. In the above example if the temperature is greater then 50 alarm will go on otherwise what will happen comes in the else part. Let’s see the code for else.
temperature = 30 if temperature > 50: print("Alarm On") else: print("Alarm OFF")
In the above example, the interpreter first checks the “if condition“
- if it is true then it will execute the 3rd line and will not go into else statement
- If the condition of “if” is false then it will go for else.
ELIF statement
Now if you are instructed to send a message to fire brigade if the temperature is greater than 40 and sound the alarm if the temperature goes above 50 then how you will write this problem?
We have 3 conditions
- Check if the temperature is greater than 40 degrees then inform the fire brigade
- Check if the temperate is greater than 50 degrees then sound the alarm
- Else keep the alarm off.
Let’s code these condition in code.
temperature = 45 if temperature > 40: print("Call fire brigade") elif temperature > 50: print("Alarm On") else: print("Alarm OFF")
In the above code, the interpreter first checked
- If the temperature is above 40 degrees then call
- Else if check the temperature if it is above 50 degrees then turn on the Alarm
- Else keep the alarm off
As the temperature was 45 degrees so the program only prints “Call fire brigade”
Nested IF statement
If you are instructed to write a program to turn on the alarm at 50 degrees and then turn on the sprinkles if the alarm is turned on then you need to use nested if statements.
Now first we have to check if the temperature is greater than 50 and then check if the alarm is turned on. Let’s see this code.
temperature = 60 alarm = "off" if temperature > 50: print("Alarm on") alarm = "on" if alarm == "on": print("turn on sprinkles")
Switch Case statement
Old languages like c++ have switch statements but Python did not include the switch statement as everything that a switch statement is used to do can be done using if, else, elif statements.
But there is a way to implement switch cases in Python using dictionaries. Let’s have a look at code.
def select(option): items = { 0: “call fire brigade”, 1: “sound the alarm”, 2: “turn on sprinkles” } return items.get(option, “keep the alarm off”) print(select(1)) |
As we passed the “1” as an option the function will return the statement which is the value of the “1”
If we pass “2” it will return “turn on sprinkles”