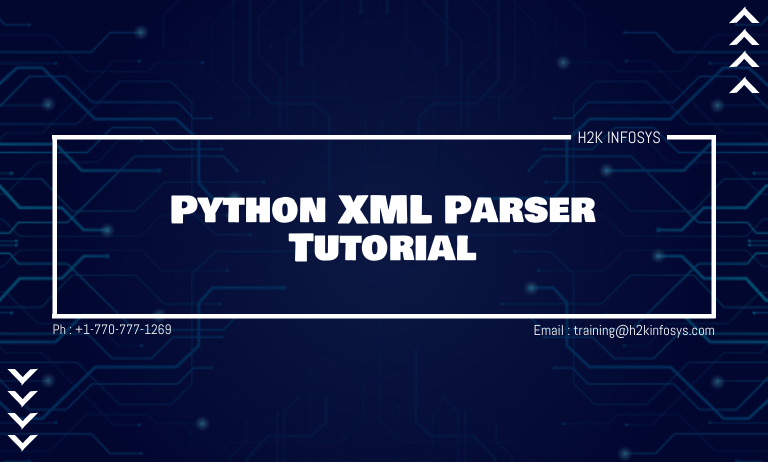
Extensible Markup language (XML) was developed in 1998 to make it both human and machine-readable, designed to store any amount of data, and is widely used for sharing information across the internet. In this, Python XML Parser Tutorial you will learn to parse and modify an XML document
In XML there are no built-in tags like HTML has. We declare tags as we want.
Python is a general-purpose programming language; it allows us to parse and modify an XML document. There are two main libraries in Python that deals with XML.
- Minidom
- ElementTree
Let’s start with Minidom
Let’s first create a simple XML file that we are going to use in this tutorial. Create a file with the name notes.xml as shown below.
<note> <to>Tove</to> <from>Jani</from> <heading>Reminder</heading> <body>Don't forget me this weekend!</body> </note>
How to Parse XML using minidom
Now let’s parse the notes.xml file and print the node name that is ‘document’ and first child tag name that is ‘note’ as we created in the XML file.
import xml.dom.minidom notes = xml.dom.minidom.parse('notes.xml') print(notes.nodeName) print(notes.firstChild.tagName)
Now let’s print out the data in the first Child.
import xml.dom.minidom notes = xml.dom.minidom.parse('notes.xml') note = notes.getElementsByTagName("to") for i in note: print(i.firstChild.data)
The following will be the output.
How to Parse XML using ElementTree
ElementTree is easy as compared to Minidom. Let’s take a look at how to parse XML file using ElementTree.
We just need the following code to print all the data in our notes.xml file.
The following will be the output.
Let’s write data to XML file using ElementTree
import xml.etree.ElementTree as ET # create the file structure data = ET.Element('notes') items = ET.SubElement(data, 'Persons') item1 = ET.SubElement(items, 'person1') item2 = ET.SubElement(items, 'person2') item1.set('name','salman') item2.set('name','Ahmed') item1.text = 'Hello Salman' item2.text = 'Hello Ahmed' # create a new XML file with the results mydata = ET.tostring(data) myfile = open("notes1.xml", "wb") myfile.write(mydata)
The following will be the output.
The following will be the output of XML file.