JAVA Tutorials
SERIALIZABLE INTERFACE
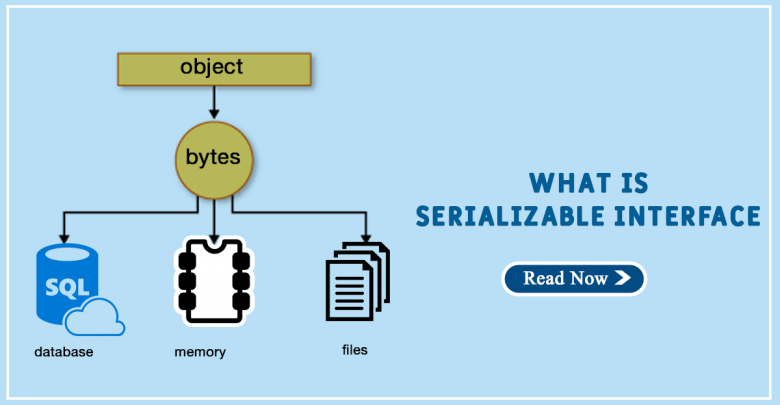
Introduction to Serialization:
- It is a way of converting the state of an object into a byte stream.
- The reverse process of Serialization is known as deserialization, where the byte-stream is converted into an object.
- Serialization and Deserialization process is a platform-independent, and it means you can serialize an object in a platform and deserialize on a different platform.
- For the serializing, we invoke the writeObject() method ObjectOutputStream, and for the deserialization we invoke the readObject() method of ObjectInputStream class.
For serializing the object, we must implement the Serializable interface.
Advantages of Serialization
- It is basically used to move the object’s state on the network.
java.io.Serializable interface
- The Serializable is a marker interface without data members and methods.
- The Cloneable and Remote is also the marker interfaces.
- It must be implemented by the class whose object you need to remain.
Note: The String class and all the wrapper classes implement the java.io.Serializable interface by default.
Let’s see the examples given below:
PROGRAM: 1
import java.io.Serializable; public class Candidates implements Serializable { int id; String name; public Student (int id, String name) { this.id = id; this.name = name; } }
PROGRAM: 2
import java.io.*; public class SerializeTest { public static void main(String [] args) { Employee e = new Employee(); e.name = “Ram”; e.address = “Delhi”; e.number = 103; try { FileOutputStream fileOut = new FileOutputStream(“/test/employee.ser”); ObjectOutputStream out = new ObjectOutputStream(fileOut); out.writeObject(e); out.close(); fileOut.close(); System.out.printf(“Serialized data is saved in /test/employee.ser”); } catch(IOException i) { i.printStackTrace(); } } }
PROGRAM: 3
public class Candidate implements java.io.Serializable{ private int candRollNum; private int candAge; private String candName; private transient String candAddress; private transient int candHeight; public Candidate (int c_roll, int c_age, String c_ name, String c_address, int c_height) { this.candRollNum = c_roll; this.candAge = c_age; this.candName = c_name; this.candAddress = c_address; this.candHeight = c_height; } public int getCandRollNum() { return candRollNum; } public void setCandRollNum(int candRollNum) { this.candRollNum = candRollNum; } public int getCandAge() { return candAge; } public void setCandAge(int candAge) { this.candAge = candAge; } public String getCandName() { return candName; } public void setCandName(StringcandName) { this.candName = candName; } public String getCandAddress() { return candAddress; } public void setCandAddress(String candAddress) { this.candAddress = candAddress; } public int getCandHeight() { return candHeight; } public void setCandHeight(int candHeight) { this.candHeight = candHeight; } }
Serialization Using Object and Use the above class(Candidate)
This class is writing an object of Candidate class to the Candidate. ser file.
Now, we are using the FileOutputStream and ObjectOutputStream to write the object to File.
Note: For Java Serialization, the file name should have a .ser extension.
import java.io.FileOutputStream; import java.io.ObjectOutputStream; import java.io.IOException; public class SerialClass { public static void main(String args[]) { Candidate obj = new Candidate(101, 25,"Seeta", "Agra", 6); try{ FileOutputStream fos = new FileOutputStream("Candidate.ser"); ObjectOutputStream oos = new ObjectOutputStream(fos); oos.writeObject(obj); oos.close(); fos.close(); System.out.println("Serialization Successfully Done!!"); } catch(IOException ioe) { System.out.println(ioe); } } }
Output: Serialization Successfully Done!!
Facebook Comments
2 Comments