Are You Ready to Ace Your Selenium Interview?
The tech industry is booming with automation roles, and Selenium remains one of the most in-demand tools for automating web applications. Whether you’re a beginner or already have some automation experience, preparing for common Selenium interview scenarios is essential. Recruiters don’t just look for textbook knowledge they want proof of real-world problem-solving.
If you’re enrolled in a Selenium certification course, this blog will elevate your understanding by walking you through the top 5 Selenium interview scenarios you’re most likely to face. With a hands-on approach and practical code examples, this guide is perfect for learners of any online Selenium course or anyone pursuing automation certification online.
Why Interview Scenarios Matter
Real-world scenarios test not only your understanding of Selenium commands but also how you apply them under specific conditions. According to a survey by Stack Overflow, over 60% of automation testers said that scenario-based questions were the most challenging part of Selenium interviews.
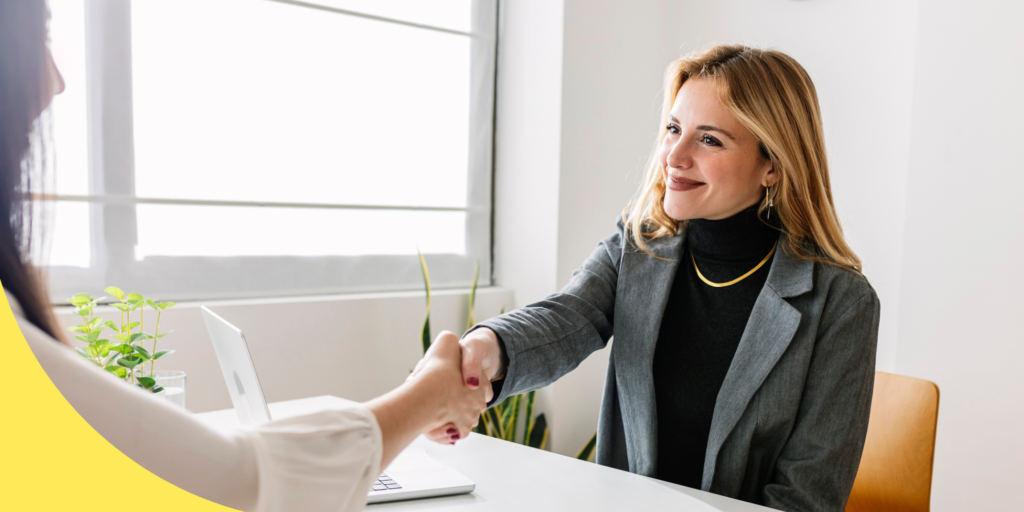
That’s why scenario prep is just as important as technical prep.
Scenario 1: Dynamic Web Elements Handling
Problem Statement:
“How would you handle elements whose properties change dynamically with every page load?”
Real-World Relevance:
Web pages often generate dynamic elements with attributes that change every time the page loads. Identifying these elements requires a deeper understanding of locator strategies.
Code Example:
java
// Using XPath with contains()
WebElement dynamicElement = driver.findElement(By.xpath("//*[contains(@id,'login-button')]"));
dynamicElement.click();
Explanation:
- Here, we use the
contains()
function in XPath to match partial attribute values. - This method is ideal for dynamic
id
,class
, orname
attributes.
Pro Tip:
Always combine dynamic locators with explicit waits to ensure the element is ready before performing actions.
java
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.xpath("//*[contains(@id,'login-button')]")));
element.click();
Skill Demonstrated:
Mastery in XPath and wait strategies topics covered in most Selenium training online platforms.
Scenario 2: Handling Multiple Windows or Tabs
Problem Statement:
“How do you handle operations in multiple browser windows or tabs?”
Real-World Relevance:
Modern web applications often trigger new windows for payments, downloads, or social logins. Managing these windows effectively is key to real-time automation.
Code Example:
java
String mainWindow = driver.getWindowHandle();
for(String windowHandle : driver.getWindowHandles()) {
if (!mainWindow.equals(windowHandle)) {
driver.switchTo().window(windowHandle);
driver.findElement(By.id("submit-button")).click();
driver.close();
}
}
driver.switchTo().window(mainWindow);
Explanation:
- Store the main window.
- Loop through all open windows and switch to the child.
- Perform required operations, then return to the main window.
Skill Demonstrated:
Control over browser contexts, an essential topic in any online Selenium course.
Scenario 3: Data-Driven Testing with Excel Integration
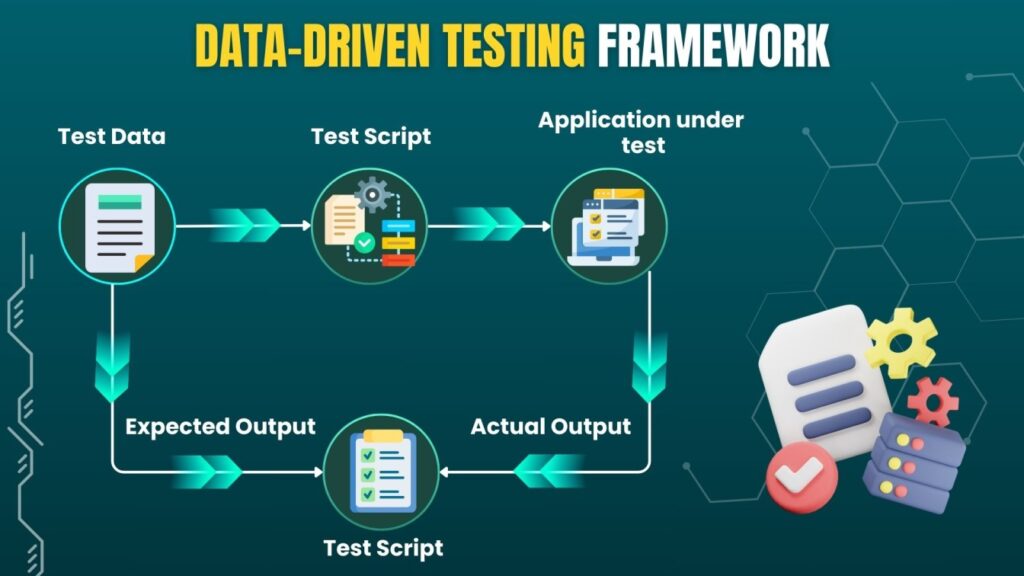
Problem Statement:
“How would you perform data-driven testing using Selenium and Excel?”
Real-World Relevance:
Testing the same functionality with multiple datasets (login credentials, form inputs) is common. This scenario often uses Apache POI for Excel integration.
Code Example:
java
FileInputStream file = new FileInputStream(new File("data.xlsx"));
XSSFWorkbook workbook = new XSSFWorkbook(file);
XSSFSheet sheet = workbook.getSheetAt(0);
for (int i = 1; i <= sheet.getLastRowNum(); i++) {
String username = sheet.getRow(i).getCell(0).getStringCellValue();
String password = sheet.getRow(i).getCell(1).getStringCellValue();
driver.findElement(By.id("user")).sendKeys(username);
driver.findElement(By.id("pass")).sendKeys(password);
driver.findElement(By.id("login")).click();
}
Explanation:
- Apache POI is used to read data from Excel files.
- Inputs are passed to the Selenium WebDriver inside a loop.
Skill Demonstrated:
Practical implementation of data-driven testing key to acing any scenario-based interview round and emphasized in automation certification online programs.
Scenario 4: Automating Alerts and Pop-Ups
Problem Statement:
“How do you handle JavaScript alerts and confirmation pop-ups?”
Real-World Relevance:
Applications often use JavaScript alerts to confirm user actions or display errors.
Code Example:
java
Alert alert = driver.switchTo().alert();
System.out.println("Alert Message: " + alert.getText());
alert.accept(); // To click OK
// alert.dismiss(); // To click Cancel
Explanation:
- Switch context to alert using
switchTo().alert()
. - Perform desired action: accept, dismiss, or read the message.
Skill Demonstrated:
Handling unexpected browser events part of advanced Selenium topics often included in a Selenium certification course.
Scenario 5: Framework Integration with TestNG and Maven
Problem Statement:
“Explain how you integrate Selenium with TestNG and Maven for automated test execution.”
Real-World Relevance:
No organization runs Selenium scripts in isolation. Integration with build tools and test frameworks is a must.
Framework Overview:
- Maven – Manages dependencies and project builds.
- TestNG – Organizes test cases, enables parallel execution, and generates reports.
Code Example: pom.xml
snippet for Maven
xml
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.1.0</version>
</dependency>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.1.0</version>
<scope>test</scope>
</dependency>
</dependencies>
TestNG Example:
java
public class LoginTest {
@Test
public void testLogin() {
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
driver.findElement(By.id("user")).sendKeys("admin");
driver.findElement(By.id("pass")).sendKeys("admin123");
driver.findElement(By.id("login")).click();
driver.quit();
}
}
Skill Demonstrated:
Understanding of CI/CD pipeline readiness and test case management typically covered in intermediate to advanced Selenium training online.
Bonus Tips for Cracking Selenium Interview Scenarios
Always Justify Your Approach
Writing code is only part of the equation in Selenium Interview Scenarios. What sets a standout candidate apart is the ability to articulate the logic behind every decision. For example, if you’re using XPath
instead of CSS Selectors
, be ready to explain that XPath
offers more flexibility for dynamic elements that change frequently. Similarly, if you choose explicit waits over implicit ones, clarify how it gives you finer control and reduces test flakiness in asynchronous environments.
This kind of explanation can often turn a good answer into a great one especially in scenario-based interviews that evaluate how well you understand Selenium’s real-world use.
Example Response in a Selenium Interview Scenario:
“I chose
WebDriverWait
withExpectedConditions.elementToBeClickable
because the page contains dynamic loading elements. This ensures my test waits until the element is ready to be interacted with, which prevents flaky failures in our automation suite.”
Key Takeaway: Employers look for engineers who not only write working code but also demonstrate critical thinking in Selenium Interview Scenarios. Always prepare to explain your logic with clarity.
Be Framework-Ready
Modern Selenium Interview Scenarios go beyond simply executing basic scripts they assess your ability to work with or build robust test automation frameworks. This is crucial because organizations expect Selenium testers to design maintainable, scalable test suites integrated into larger software development pipelines.
Here’s what often comes up in Selenium framework-related interview scenarios:
- Page Object Model (POM): This pattern separates locators and actions from test logic, making the code more reusable and maintainable.
- Behavior-Driven Development (BDD) with Cucumber: Allows collaboration among QA, developers, and product owners using simple Gherkin syntax to define behavior.
- TestNG or JUnit: These testing frameworks manage test execution flow, allow grouping, parameterization, and help generate detailed reports.
- Maven or Gradle: Manage your project’s structure and dependencies automatically for seamless integration.
- CI/CD tools (Jenkins, GitHub Actions): Execute your Selenium test suites automatically after every code commit or pull request.
Practical Tip: Be prepared to walk an interviewer through a framework you’ve worked on. Detail how you used Selenium with TestNG, how you structured your Page Objects
, handled test data with Excel or JSON, and implemented parallel testing.
Bonus Insight: Many online Selenium courses and Selenium certification courses now include framework-building as part of the curriculum. If yours doesn’t, consider upgrading to stay competitive in interviews involving Selenium Interview Scenarios.
Brush Up on Java Basics
In most Selenium Interview Scenarios, the expectation is not only to understand how Selenium works, but also to be fluent in Java programming. Since Selenium WebDriver integrates closely with Java, many scenario-based questions involve writing complete automation scripts that utilize core Java features.
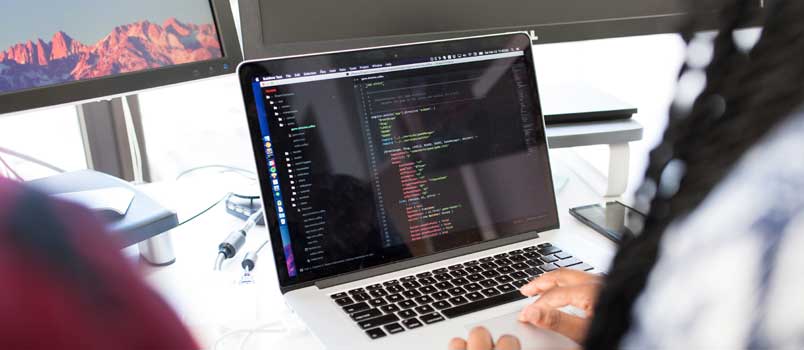
Essential Java Topics for Selenium Interview Scenarios:
- Object-Oriented Programming (OOP): Concepts like inheritance, interfaces, and abstraction are often used in framework development.
- Collections Framework: Know how to use
List
,Map
, andSet
effectively, especially for managing multiple web elements or test data. - Exception Handling: Write robust test scripts that use
try-catch-finally
blocks to handle pop-ups, timeouts, or null pointer exceptions gracefully. - File Handling: Read test data from files like Excel, CSV, or JSON to support data-driven testing.
- Loops and Conditions: Use these for iterating over test data or managing conditional UI workflows.
Sample Interview Scenario:
“Write a Selenium script to log in using multiple sets of credentials stored in an Excel file. Use Apache POI for file reading and Java collections for data handling.”
Preparation Tip: Many learners in Selenium training online programs underestimate the importance of Java skills. But in real Selenium Interview Scenarios, your Java logic can be the difference between a pass and a fail. Make sure your automation certification online includes deep dives into Java as well.
Final Thought on Bonus Tips
By mastering these bonus tips, you’re better prepared for both the technical and strategic dimensions of common Selenium Interview Scenarios. Understanding frameworks, Java logic, and the reasoning behind each automation choice ensures that you’re not just another test engineer but a standout automation expert ready for the real world.
Key Takeaways
- Scenario-based questions are crucial in Selenium interviews.
- Mastering locators, waits, alerts, data handling, and frameworks sets you apart.
- Real-world problems and frameworks matter more than just writing click-and-type scripts.
- Practice these scenarios in a local setup or as part of your online Selenium course.
Conclusion
Want to go beyond theory and practice real-world automation challenges? Join H2K Infosys’ Selenium course for hands-on projects, mock interviews, and expert-led training. Start your journey to becoming a certified automation tester today.