Understanding Python list Index with Example
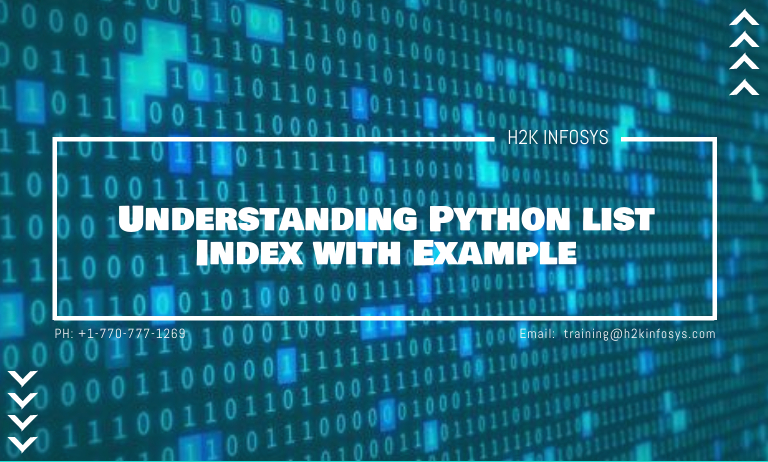
A list is perhaps the most frequently used inbuilt data structure in Python. It is a container that is used to store a collection of data. The data could be of different data types. Be it strings, integers, booleans, Nonetype, floats, etc. The data are encapsulated in square brackets ([]), and each element (or item) is separated by a comma (,).
The elements in a list are accessed through indexing. The index can be seen as a pointer to the actual data. The indexes of elements in a list are numbers from 0 to the list’s length. It is important to emphasize that the indexing begins from 0 and not one. This means that the first element has an index of 0. Following that pattern, the last element has an index of n-1 where n is the number of elements in the list.
Several functions/methods are used to carry out different operations to a list. You can add a new element to a list, remove an element from a list, sort a list, loop over a list, add a list to a list (nested lists), and many more. This tutorial will focus on one of those methods, an important one. And that’s the index() function. We will go a step further to see other methods of getting the index of a list.
By the end of this tutorial, you will learn.
- How to use the index() function
- How to use for loops to find list indexes
- How to use list comprehensions
- How to use while loops to find list indexes
- How to use NumPy to find the indexes in a list
Let’s jump right into it.
Python list index()
The index() method is used to find the index of a list. There are several other methods to return the list index, but the easiest is the index() method. It takes the element whose index you want to be returned. If that element appears more than once in the list, the index() function returns the first copy of that element’s index. Let’s see its syntax.
Syntax
list.index(element, start, end)
The start and end arguments are optional. As explained earlier, python begins indexing from 0 and according to its documentation, stops at 9223372036854775807. You can modify how you want the Python interpreter to start and stop its indexing by defining the start and end argument.
Lets see some examples.
#create a list names = ['Felix', 'Vijay', 'Tom', 'Anna', 'Ola', 'Wu'] #check the index of different element print(f"The index of Felix is: {names.index('Felix')}") print(f"The index of Vijay is: {names.index('Vijay')}") print(f"The index of Anna is: {names.index('Anna')}") print(f"The index of Wu is: {names.index('Wu')}")
Output:
The index of Felix is: 0
The index of Vijay is: 1
The index of Anna is: 3
The index of Wu is: 5
As seen the first element has an index of 0.
Passing an element that is not on the list.
If the element passed in the index() method is not on the list for some reason, the python interpreter returns a Value Error. We can try it out. Obviously, ‘Toby’ is not on the list. Let’s see what happens if we pass it as an argument.
#create a list names = ['Felix', 'Vijay', 'Tom', 'Anna', 'Ola', 'Wu'] #check the index of Toby print(f"The index of Toby is: {names.index('Toby', 0, 100)}")
Output:
Traceback (most recent call last):
File "c:/Users/DELL/Documents/VS codes files/fresh.py", line 5, in <module>
print(f"The index of Toby is: {names.index('Toby', 0, 100)}")
ValueError: 'Toby' is not in list
The Value Error was returned as expected.
Finding the index of an element using for loop
While using the index method may be the easiest way to return the index of a list, other methods are available. We can also use a for loop to traverse the elements in a list and return their index. The index is captured by calling the range() function on the len() function. Let’s take a coding example.
#create a list names = ['Felix', 'Vijay', 'Tom', 'Anna', 'Ola', 'Wu'] indexes = [] for index in range(len(names)): indexes.append(index) print(f'The list {names}') print(f'The index {indexes}')
Output:
The list ['Felix', 'Vijay', 'Tom', 'Anna', 'Ola', 'Wu']
The index [0, 1, 2, 3, 4, 5]
Finding the index of an element that appears more than once
With for loops, if an element appears more than once, you can return the index of each element. Recall that when using the index() method, it returns only the first copy of the element in the event that it appears more than once in the list. Let’s see an example.
#create a list with Felix appearing thrice names = ['Felix', 'Vijay', 'Tom','Felix', 'Felix', 'Anna', 'Ola', 'Wu'] indexes = [] for index in range(len(names)): if names[index] == 'Felix': indexes.append(index) print('Felix appears at index ', indexes)
Output:
Felix appears at index [0, 3, 4]
Finding the index of a list using List comprehension
List comprehension provides a shorter alternative to writing for loops and appending results to a list. Hence, the previous section’s example can be reproduced using for loops. See the code below.
#create a list with Felix appearing thrice names = ['Felix', 'Vijay', 'Tom','Felix', 'Felix', 'Anna', 'Ola', 'Wu'] #create a list comprehension for to return index for Felix result = [index for index in range(len(names)) if names[index] == 'Felix'] print('Felix appears at index ', result)
Output:
Felix appears at index [0, 3, 4]
Using While Loops to return Indexes of elements appearing more than once.
While loops can as well be used to return the index of an element that appears more than once. They are used along with the index() method to achieve this. While loops are generally longer than for loops. See the coding example below.
#create a list with Felix appearing thrice names = ['Felix', 'Vijay', 'Tom','Felix', 'Felix', 'Anna', 'Ola', 'Wu'] result = [] loop_index = -1 #create an infinite loop while True: try: #append the index of Felix loop_index = names.index('Felix', loop_index + 1) result.append(loop_index) #break the loop when the index() method returns a value error #it means the list has been traversed completely except ValueError: break print('Felix appears at index ', result)
Output:
Felix appears at index [0, 3, 4]
Using the Enumerate Function to get the index of a list.
The enumerate function is another method of returning the index of elements in a list. The enumerate function, when called, returns a list of tuples that contains the index and element of the list.
For example, if a list contains say [‘Felix’, ‘Vijay’, ‘Tom’,’Felix’, ‘Felix’, ‘Anna’, ‘Ola’, ‘Wu’] calling he enumerate function returns [(0, ‘Felix’), (1, ‘Vijay’), (2, ‘Tom’), (3, ‘Felix’), (4, ‘Felix’), (5, ‘Anna’), (6, ‘Ola’), (7, ‘Wu’)]
Now let us see how to use the enumerate function to return the index of a specific element in a list.
#create a list with Felix appearing thrice names = ['Felix', 'Vijay', 'Tom','Felix', 'Felix', 'Anna', 'Ola', 'Wu'] #create a list comprehension for to return index for Felix result = [index for index, _ in enumerate(names) if names[index] == 'Felix'] print('Felix appears at index ', result)
Output:
Felix appears at index [0, 3, 4]
The underscore (_) was used because the element itself was not going to be used anywhere in the code.
Using NumPy to get the index of an element in a list
Numpy is a popular Python library used for numerical computation. You can also use the NumPy library to find the index of an element in a library. First off, you have to make sure you have NumPy running on your machine. To do so, type
pip install numpy
On your command prompt and wait for it to download all its requirements. Once it’s done, you can try to import it to check if it was installed correctly. To import numpy, just type
import numpy as np
If the above code runs without any errors, it means that it has run successfully. To use lists in NumPy, you need to convert the list into a NumPy array. After using the where() method to return the index of the element where a condition is satisfied. In our case, the condition would be where the element in the list is Felix. See the coding example below.
#import the necessary library import numpy as np #create a list with Felix appearing thrice names = ['Felix', 'Vijay', 'Tom','Felix', 'Felix', 'Anna', 'Ola', 'Wu'] #convert names into a numpy array names = np.array(names) result = np.where(names == 'Felix') print('Felix appears at index ', result)
Output:
Felix appears at index (array([0, 3, 4], dtype=int32),)
In summary, you have seen the different methods to access the elements in a list. Here are some vital takeaway
- The index() method returns the index of the first element that matches the argument passes. It is the quickest and easiest method.
- In cases where the argument passed is not an element in the list, the python interpreter throws a ValueError
- You can use for and while loops to find the index of elements in a list.
- You can use list comprehensions to make for loops shorter
- You can use the enumerate function
- You can use the numpy.where() function to return the index of the list’s element.
If you’ve got any questions, please leave them in the comment section and I’d do my best to answer them.
One Comment