What is Spring AOP?
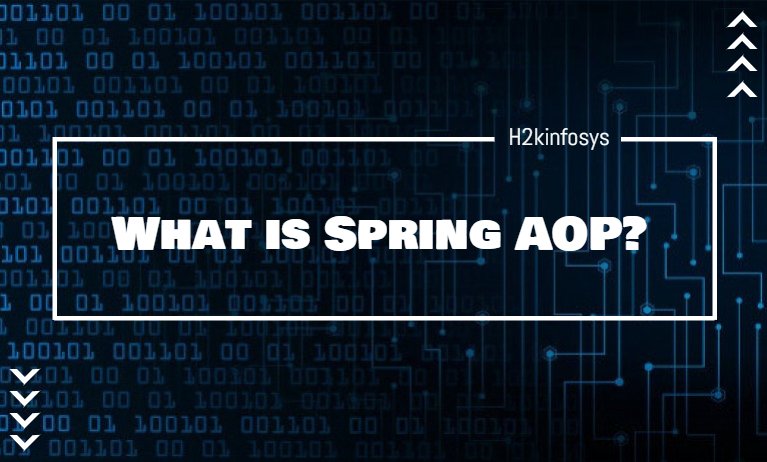
AOP (Aspect Oriented Programming) is a programming methodology that provides modularity. It breaks down the program logic into distinct parts known as concerns.
In OOPs, the key unit of modularity is class, while in AOP, the key unit is the aspect.
A cross-cutting concern is defined as a concern that affects the complete application and is centralized in one location in the code. Examples of aspects are caching, logging, security, authentication, etc.
AOP is used to provide declarative enterprise services, and also it allows users to implement custom aspects.
Why AOP?
It provides a way to add the additional concern before, after or around the logic dynamically.
Suppose in a class there are 10 methods available
class A{ public void m1(){...} public void m2(){...} public void m3(){...} public void m4(){...} public void m5(){...} public void n1(){...} public void n2(){...} public void p1(){...} public void p2(){...} public void p3(){...} }
Here five methods start from m, two methods start from n, and three methods start from p.
You have to maintain a log and want to send a notification after calling methods that start from m.
The first method is to write the code in all the five methods. Let us say in future client does not want to send notification then you need to change all the methods leading to the maintenance issue.
Another method is to define additional concern for maintaining a log and sending notifications that will be entered in the XML file. So in the future client wants to remove the notification functionality you need to change only in the XML file. Hence maintenance becomes easy with the help of AOP.
The terminology used in AOP:
- Join Point: Any point in the program like method execution, exception handling, etc., where you can plug-in the AOP aspect is a join point.
- Advice: An action taken at any join point by an aspect is called Advice. The different types of Advice are:
- Before Advice: executes before a join point.
- After Returning Advice: executes after a join point finishes normally.
- After Throwing Advice: executes if the method throws an exception.
- After (finally) Advice: executes after a join point.
- Around Advice: it executes before and after the join point.
- Pointcut: It is an expression language to match join points.
- Introduction: It allows you to add new methods, attributes, interfaces to the existing classes.
- Target Object: It is the object that is advised by one or more aspects. Spring AOP is implemented using run time proxies. Hence also called a proxied object.
- Aspect: Aspect is a class that contains advice, joinpoints, etc.
- Interceptor: An Aspect, which contains only one piece of Advice, is known as an interceptor.
- AOP Proxy: This is used to implement aspect contracts. It uses JDK Dynamic Proxy.
- Weaving: The process of linking aspect with other application types to create an advised object and is done at run time, compile-time, and load time.
AOP Implementations:
AOP can be implemented by:
- AspectJ: This is an extension of Java Programming creates at the PARC research center. It uses the same syntax as you do in Java and includes IDE integrations. It has its own weaver and compiler.
- Spring AOP: This uses XML based configurations as well as annotations which uses libraries of AspectJ for parsing and matching purpose.
- JBoss AOP: This is an open-source Java Application developed by JBoss.
Example of Spring AOP:
Step 1: Import Spring AOP Dependencies say pom.xml
<dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>5.2.7.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context-support</artifactId> <version>5.2.7.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aop</artifactId> <version>5.2.7.RELEASE</version> </dependency> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjrt</artifactId> <version>1.9.5</version> </dependency> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjweaver</artifactId> <version>1.9.5</version> </dependency>
Step 2: Enable AOP Configurations in AopConfig.java
@Configuration @EnableAspectJAutoProxy public class AopConfig { }
Step 3: Write Aspect and pointcut expressions in EmployeeCRUDAspect.java
@Aspect public class EmployeeCRUDAspect { @Before("execution(* EmployeeManager.getEmployeeById(..))") //point-cut expression public void logBeforeV1(JoinPoint joinPoint) { System.out.println("EmployeeCRUDAspect.logBeforeV1() : " + joinPoint.getSignature().getName()); } }
Step 4: Write methods to execute advices in EmployeeManager.java
@Component public class EmployeeManager { public EmployeeDTO getEmployeeById(Integer employeeId) { System.out.println("Method getEmployeeById() called"); return new EmployeeDTO(); } }
Step 5: Run the application using TestAOP.java
public class TestAOP { @SuppressWarnings("resource") public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext ("com/demo/aop/applicationContext.xml"); EmployeeManager manager = context.getBean(EmployeeManager.class); manager.getEmployeeById(1); } }
OUTPUT:
EmployeeCRUDAspect.logBeforeV1() : getEmployeeById Method getEmployeeById() called
One Comment