Handling Exceptions in JSP
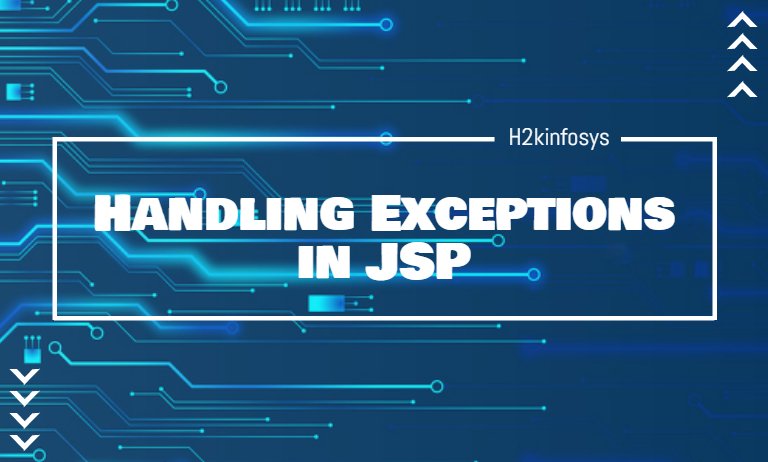
In the JSP, there are two technics for handling exceptions. Firstly, we can use the exception implicit object and other, try-catch block inside a scriptlet
During application execution, an unwanted and unexpected event can occur. It can be because of coding mistakes made by the programmer, problems with handling a wrong input, and other unforeseeable things.
When an issue occurs, Java normally generates an error message and can even stop the execution of the whole application. In this situation we use the technical term: Java throws an exception (throws an error).
Let’s understand the difference between an Error and an Exception.
An Error indicates a serious problem occurred during the execution of an application that a reasonable application should not try to catch. If error it can be a virtual machine error, an assertion error, etc.
An Exception indicates conditions where some issues occurred, but that a reasonable application might try to catch.
An application has to handle exceptions and to give meaningful messages to the user, so that the user would be able to understand the issue and take some appropriate actions. For example, an exception occurred because of the wrong data entered by the user. He has to get a message that data are wrong and it will help him to fix the not a pleasant situation.
The most known exceptions: IOException, JAXBException, SQLException, TimeoutException, DataFormatException, XMLParseException, etc.
The Java language has checked and unchecked exceptions.
The checked exceptions are the exceptions that are checked at the time of compilation. If some code within a method throws a checked exception, then the method has to handle it by declaring the exception in the method signature using throws keyword, using a try-catch block.
For example, we are reading some text file and want to display it on the screen. The checked exception can be thrown in the three places. If the file doesn’t exist we can get FileNotFoundException. The during reading the file we can get IOException. Also, the method that closes the file input stream can throw IOException. In all these three cases we need to add a try-catch block, in case an exception occurs, or propagate an exception further by the use of throws operator in the method signature. Without code that is handling a checked exception, we will get a compilation error.
RuntimeException is the superclass of exceptions that can appear during any normal running of operations on the Java Virtual Machine. All subclasses of RuntimeException are unchecked exceptions. This type of exception does not need to be handled in a method or constructor even if they can be thrown during the execution of the method or constructor.
The most known runtime exceptions: ArithmeticException, ClassCastException, FileSystemNotFoundException, IllegalArgumentException, IllegalMonitorStateException, IndexOutOfBoundsException, NoSuchElementException, NullPointerException, WrongMethodTypeException. In the case of the unchecked exceptions, the program would not give a compilation error if you don’t have a code for handling it. Most of the times these exceptions are related to the bad data provided by a user. The programmer can decide to handle the conditions that can cause an exception or not handle it. One of the examples of RuntimeException can be some arithmetic operation and accidentally you got division by zero, or you are working on arrays and your iterator is bigger than the number of elements in the matrix. The most popular is probably the situation when your object is not created(it is null), and you are calling a method on it.
Errors are ignored in code because the developer in most cases can do nothing to fix an error. For example, a stack overflow causes an error. They are ignored during compilation time. As an example, we can take another error OutOfMemoryError. This error is not easy to handle, it requires a long work and deep analysis of the situation.
You can create your own checked exceptions. For this, your exception class needs to extend the Exception class or unchecked exception by extending RuntimeException class.
Handling Exceptions in the JSP pages
In the JSP, there are two technics to handle exceptions. Firstly, we can use the exception implicit object. Another way of exception handling is to use a try-catch block inside a scriptlet. Let’s look at the examples of these two methods.
Exception handling using exception implicit object
There is a possibility in JSP to specify the exception handling page with the use of the Page directive and its errorPage attribute. In the case of any exception, in the main JSP page, the control will be switched to the page mentioned in the errorPage attribute of the Page directive. We will create the errorpage.jsp page as an exception handler page.
We will use the exception implicit object. And for this, the handler page should have isErrorPage flag set to true. In another way, we would not be able to use the exception implicit object. Because of this reason we have set the isErrorPage as true for errorpage.jsp.
Let’s intentionally create situation when the NullPointerException is thrown. Here is the code of our page test.jsp with exception:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1" errorPage="errorpage.jsp" %> <!DOCTYPE html> <html> <head> <title>Example of JSP exception handling</title> </head> <body> <% //Declared and initialized object with null Integer integer = null; //It should throw NullPointer Exception integer.intValue(); %> </body> </html>
Here is the errorpage.jsp:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1" isErrorPage="true" %> <!DOCTYPE html> <html> <head> <title>Page to display the Exception Message</title> </head> <body> <h2>Exception!</h2> <i>An exception has occurred in the test.jsp Page. Please fix it. Below is more information:</i> <b><%= exception %></b> </body> </html>
The demonstration of work:
Let’s create another example where we will give the possibility for the client to enter data. Here is test.jsp code where user can enter data:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1" %> <!DOCTYPE html> <html> <head> <title>Division</title> </head> <body> <form action="result.jsp"> <h2>Enter two numbers for Division</h2> First Input Number:<input type="text" name="first_number" /> Second Input Number:<input type="text" name="second_number" /> <input type="submit" value="Get Results"/> </form> </body> </html>
After hitting the button “Get Results” the client will be redirecting to result.jsp page:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1" isErrorPage="true" %> <!DOCTYPE html> <html> <head> <title>Page to display the Exception Message</title> </head> <body> <%@ page errorPage="exception.jsp" %> <% String num1=request.getParameter("first_number"); String num2=request.getParameter("second_number"); int v1= Integer.parseInt(num1); int v2= Integer.parseInt(num2); int res= v1/v2; out.print("Output is: "+ res); %> </body> </html>
When issue occured the client was redirected to error page exception.jsp:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <!DOCTYPE html> <html> <head> <meta charset="ISO-8859-1"> <title>Exception occured</title> </head> <body> <%@ page isErrorPage="true" %> Application got this Exception: <%= exception %> Please enter another data to get a correct result. </body> </html>
Exception handling using try-catch block within scriptlets
Let’s try to handle an exception inside scriptlet. We are going to use an Exception object.
All exceptions are subclasses of Throwable class and have a lot of useful methods:
Throwable getCause() – method returns the reason that caused this throwable or null for a nonexistent or unknown reason.
String getLocalizedMessage() – method creates a description of this throwable that is localized.
String getMessage() – method returns the detailed message about current throwable.
StackTraceElement[] getStackTrace() – method provides access programmaticly to the information that we can print with method printStackTrace().
void printStackTrace() – method prints current information about throwable and its backtrace to the standard error stream.
void printStackTrace(PrintStream s) – method dedicated to print current throwable and its backtrace to the print stream specified in parameters.
void printStackTrace(PrintWriter s) – method prints this throwable and its backtrace to the print writer specified in parameters.
String toString() – method returns a short description of this throwable.
Let’s try to handle an exception inside scriptlet with a try-catch block.
For this example, we will get a situation where a division by zero occurred. It is not mandatory to handle such kind of exception, because it is RuntimeException, but we will do this.
Here is our JSP page:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1" %> <!DOCTYPE html> <html> <head> <title>Exception handling using try-catch blocks</title> </head> <body> <% try{ //Declared and initialized two integers int number1 = 122; int number2 = 0; //It will throw Arithmetic Exception int div = number1/number2; out.println("The result of the division is "+ div); } catch (Exception exp){ out.println("An exception has occurred: " + exp); } %> </body> </html>
The output will be:
Let’s try another example, but with giving possibility to enter the numbers by user.
The example will be related to array of integers.
Here is test.jsp:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1" %> <!DOCTYPE html> <html> <head> <title>Division</title> </head> <body> <form action="result.jsp"> <h2>The array is: 1, 2, 3, 4, 5, 6 </h2> Number of the Element :<input type="text" name="index" /> <input type="submit" value="Get Element"/> </form> </body> </html>
This page looks like this:
Here is the page with result result.jsp:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1" %> <!DOCTYPE html> <html> <head> <title>Page to display the Exception Message</title> </head> <body> <% try{ //We have defined an array with 6 elements int arr[]={1,2,3,4,5,6}; // here we are getting element by index that client sent int index = Integer.parseInt(request.getParameter("index")); int num = arr[index]; out.println("You element has value "+num); } catch (Exception exp){ out.println("Try to enter another data. Exception occured: <br/>" + exp); } %> </body> </html>
If the client enters intex 10 in the previous page we will get: