Introduction to Exception Handling
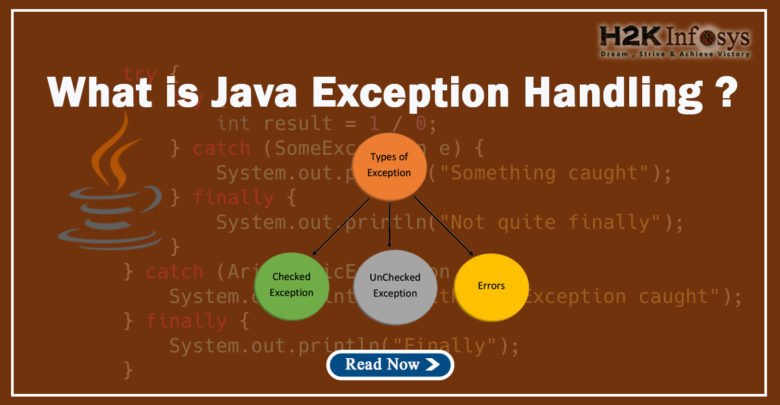
An exception is an error that disrupts the normal flow of execution of the code.
Mainly there are two types of errors:
- Compile-time errors
- Runtime errors
Compile-time errors are further classified into two types:
- Syntax Errors
- Semantic Errors
Example of Syntax Errors:
Suppose you have declared a variable name as in a; instead of declaring as int a; then, the compiler will throw a syntax error.
Let us take another example, suppose you have declared a variable int a; and after some lines, you again declare a variable as int a;. All these errors will be thrown when you compile the code.
Example of Runtime Errors:
A Runtime error is called an Exception error. It is an event that interrupts the normal flow of program execution.
Some examples of exceptions are the arithmetic exception, Nullpointer exception, Divide by zero exception, etc.
The need for Handling Exception?
Suppose you have created a program to access the server, and things worked fine while developing the code.
But during the actual production run, the server is down, and when your program tried to access it, it raised an exception.
The process to Handle Exception:
For handling exceptions, you need a Robust Programming, which takes care of such exceptional situations, and such code is called Exception Handler.
In this example, a proper exception handling would be that when the server is down, it should connect to the backup server.
To implement this, enter your code using traditional if and else to connect to the server.
Hence you will check if the server is down. If yes, then write the code to connect to the backup server.
Such implementation of code using “if” and “else” loop is not effective when there are multiple java exceptions in the code.
class connectServer{
if(Server is Up){
// code for connecting to server
}
else{
// code for connecting to BACKUP server
}
}
Try Catch Block
Java has its own inbuilt exceptional handling where the normal code goes into a TRY block and the exception handling code goes into the CATCH block.
class connectServer
{
try
{
// normal code
}
catch
{
// Exception Handling Code
}
}
In our example, TRY block contains the code to connect to the server and CATCH block contains the code to connect to the backup server.
If the server is up, the code in the CATCH block gets ignored, and if the server is down, an exception occurs, and the code in the catch block will be executed.
In this way, an exception is handled in Java.
Java Exception class Hierarchy:
After the execution of one catch statement, the others are ignored, and execution again starts continuing after the try/catch block. The exception hierarchy is followed by the nested catch blocks.
All the exception classes in Java extend the class ‘Throwable.’ Throwable consists of two subclasses, Error and Exception.
- The Error class contains those exceptions that are not expected to occur under normal circumstances. E.g., example Memory error, Hardware error, JVM error, etc.
- The Exception class contains those exceptions that can be handled by our program, and our program can recover from this exception with the help of try and catch block.
- A Runtime exception is a subclass of the exception class and, it contains those exceptions that occur at the run time and which are not being tracked at the compile time. E.g., divide by zero exception, or null pointer exception, etc.
- During input and output operations IO exception is generated.
During multiple threading, Interrupted exceptions are generated.
Java Finally Block:
The final block gets executed either an exception is raised in the try block or not and is optional to use with a try block.
try
{
//code
}
catch (ExceptionType exceptionName)
{
//code
}
Finally
{
//code
}
If an exception arises in the try block, then finally block gets executed after the execution of the catch block.
4 Comments