Expression Language
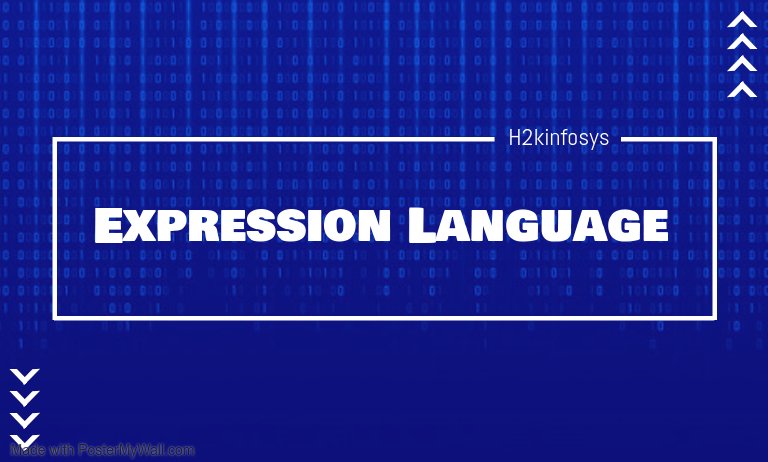
Expression Language is used to access the data. IT helps easily access the application data stored objects like Java Beans, request, session, and application, etc. The JSP EL allows software developers to access objects from code using a simple syntax.
Expression Language was introduced in JSP version 2.0. Before this feature developers used only Scriptlets, Expressions. Also, thy used the custom tag to include the server information in the JSP page.
- EL helps to integrate the server-side(controller) with the presentation(view) layer.
- EL expressions are not limited to the JSTL action attributes. It can be used in any action attribute that accepts a runtime expression.
- You can use EL expressions in the static text and can directly use in the template text outside of actions
- EL expressions are usually between the delimiters ${ and }
The Syntax of Expression Language
By default, in JSP pages the scripting elements are enabled, but EL statements and expressions are disabled. If you want to enable the EL expressions you need to use the page directive, mentioned below:
<%@ page isELIgnored="false"%>
The EL Syntax looks like this:
${expression}
In JSP, everything included in the braces is evaluated at runtime and the results are sent to the output stream. EL expressions can be mixed with a static text and combined with other expressions. Sometimes you can build really complicated expressions.
To understand expression in a JSP better, let’s look at the example below. We will use to add two numbers:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1" %> <!DOCTYPE html> <html> <head> <title>EL example</title> </head> <body> 1 + 2 = ${1+2} </body> </html>
The output will be:
1 + 2 = 3
We can use Implicit Objects in Expression Language. Developers can use them to get parameter values and attributes from different scopes. It is important to mention that EL Implicit Objects are different from JSP Implicit Objects.
The pageScope implicit object has type Map and maps attribute names with the values from the page scope.
The requestScope has also type Map. It maps attribute names with the value in the request scope.
The sessionScope has type Map and maps names of attributes with values from the session scope.
Implicit object applicationScope is a Map, contains attribute names and values from the application scope.
The param Implicit object has type Map and contains the request parameters with names and values with one-to-one relationships.
The Map paramValues is an implicit object that maps the request parameter to an array of values.
The header implicit object Map contains the request header name with its single value. The headerValues implicit object Map contains the request header name with an array of values.
The cookie Map contains the cookie name and the cookie value.
Implicit object initParam is a Map that contains the initialization parameters.
The pageContext simplicity object provides access to many objects, such as to requests, sessions, etc.
From JSP 2.1 there are two expression language resolvers for expressions handling that reference ImplicitObjectELResolver and ScopedAttributeELResolver objects.
ImplicitObjectResolver evaluate variables that match one of the implicit objects. After finding an implicit object, the MapELResolver instance resolves the attributes for it.
For example:
${sessionScope.profile}
ScopedAttributeELResolver will resolve sessionScope, MapELResolver will resolve profile attribute.
Expression Language has many reserved words: lt, le, get, get, eq, ne, true, false, and, or, not, instanceof, div, mod, empty, null. These words cannot be used then as an identifier for your variables. Some of these words from the list are not used in the language for now but they may be used there in the future.
Operators of JSP EL
The operators of the JSP expression language used in rvalue expressions :
Arithmetic operators: +, – (binary), *, / and div, % and mod, – (unary)
Logical operators: and, &&, or, ||, not, !
Relational operators: ==, eq, !=, ne, <, lt, >, gt, <=, ge, >=, le.
The empty operator is a prefix operation that is used to determine whether a value is null or empty.
Conditional operation: Looks like this: A ? B : C. Evaluates B or C, depending on the result of the evaluation of A.
The priority of operators from highest to lowest, from left to right is as follows:
- []
- () (used to change the precedence of operators)
- (unary) not ! empty
- * / div % mod
- + – (binary)
- < > <= >= lt gt le ge
- == != eq ne
- && and
- || or
- ? :
Let’s look at some EL expressions and the results:
${999.0 == 999} - true ${(6*6) ne 36} - false ${'a' < 'z'} - true ${1 div 4} - 0.25 ${10 mod 4} - 2 ${pageContext.request.contextPath} - gets context path ${header["host"]} - returns value of a header host ${users[userName]} - returns the value of the entry named userName in the users map.
Functions
The JSP expression language gives a possibility to define a function that you can invoke in an expression. The mechanism to define functions is the same as for custom tags.
Functions refer to static methods and return value. They are identified statically at translation time.
Function parameters and invocations are specified as part of an EL expression.
Functions can be used in a static text and tag attribute values. If you want to use a function in a JSP, you need to add the taglib directive to import the tag library containing the function. You also need to preface the function invocation with the prefix from the directive.
Let’s define a function. For this, we need to develop it as a public static method in a public class.
Here is an example:
package test; public class Utils { ... public static boolean compare( String l1, String l2 ) { return l1.equals(l2); } } Then you have to add mapping for the function name as used in the EL expression to the defining class and function signature in a TLD file. Here is utils.tld file for our example: <function> <name>compare</name> <function-class>test.Utils</function-class> <function-signature>boolean compare( java.lang.String, java.lang.String )</function-signature> </function> For example, let's imports the some Util library and invokes the function compare in an expression: <%@ taglib prefix="u" uri="/utils"%> ... <c:when test="${u:compare(string1, string2)}" >
Here, the expression referencing the function is using immediate evaluation syntax.
Example:
Let’s create an example and demonstrate how expression language works. We will have servlet that will forward some data to our jsp. Also, we need one Java class that will play role of a structure:
import java.io.Serializable; public class Person implements Serializable{ private String name; private int age; public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } } Here is our servlet: package servlets; import java.io.IOException; import javax.servlet.RequestDispatcher; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.http.HttpSession; public class MyServlet extends HttpServlet { private static final long serialVersionUID = 1L; protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Setting Person Object as an attribute In The Request Scope Person personBob = new Person(); personBob.setName("Bob"); request.setAttribute("personBob", personBob); // Setting Person Object as an attribute In The Request Person personMary = new Person(); personMary.setName("Mary"); request.setAttribute("personMary", personMary); // Setting Person Object as an attribute In The Request Person personJerry = new Person(); personJerry.setName("Jerry"); personJerry.setAge(20); HttpSession session = request.getSession(); session.setAttribute("personJerry", personJerry); // Setting Sample Attributes In The Application Scope getServletContext().setAttribute("User.Cookie", "This_Is_Cookie"); RequestDispatcher dispatcherObj = getServletContext().getRequestDispatcher("/myjsp"); dispatcherObj.forward(request, response); } }
Also, we plan to set some values(context parameter) in web.xml:
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" version="2.5"> <display-name>ELExample</display-name> <context-param> <param-name>number</param-name> <param-value>100</param-value> </context-param> <servlet> <servlet-name>MyServlet</servlet-name> <servlet-class>servlets.MyServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>MyServlet</servlet-name> <url-pattern>/myservlet</url-pattern> </servlet-mapping> </web-app>
Here is our test.jsp page:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <%@ page isELIgnored="false"%> <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=US-ASCII"> <title>JSP Expression Language Example</title> </head> <body> <% pageContext.setAttribute("testAttribute", "testAttribute"); java.util.ArrayList<String> listAttributes = new java.util.ArrayList<String>(); listAttributes.add("attr1"); listAttributes.add("attr2"); pageContext.setAttribute("listAttributes", listAttributes); %> <div> Person name (Request Scope) = ${requestScope.personBob.name} <br/><br/> Person name (Without Scope) = ${personMary.name} <br/><br/> Person name and age(Session Scope) = ${sessionScope.personJerry.name} ${sessionScope.personJerry.age} <br/><br/> Page Context Attribute = ${testAttribute} <br/><br/> Page Context Attribute List = ${listAttributes[0]}; ${listAttributes[1]}; <br/><br/> Application Scope Example = ${applicationScope["User.Cookie"]} <br/><br/> Header Information = ${header["Accept-Encoding"]} <br/><br/> HTTP Method Is = ${pageContext.request.method} <br/><br/> Context Parameter Example= initParam.number <br/><br/> Arithmetic Operator EL Example = ${initParam.number + 200} <br/><br/> Relational Operator EL Example = ${initParam.number < 200} <br/><br/> </div> </body> </html>
As you can see, we showed how to work with expression language Implicit Objects and attributes from different scopes and with different types (int, String, Map). We got a piece of header information and init parameter from descriptor file web.xml. Also, we tried an arithmetic operator and relational operator of JSP expression language.
Our servlet MyServlet will create several objects of class Person, set some attributes to different scopes, and will forward the request to test.jsp.
Now, if we run our application and navigate to URL “http://localhost:8080/myservlet” we will see the following page:
It is worth to mention that in JSP expression language doesn’t throw an exception in the case of NULL. If an attribute is not found or some expression return NULL, the JSP continues to work and to show results. For arithmetic operations, EL treats NULL as 0. For logical operations, EL treats NULL as false. For String that is NULL, we get an empty String.