Importing Modules in Python with Examples
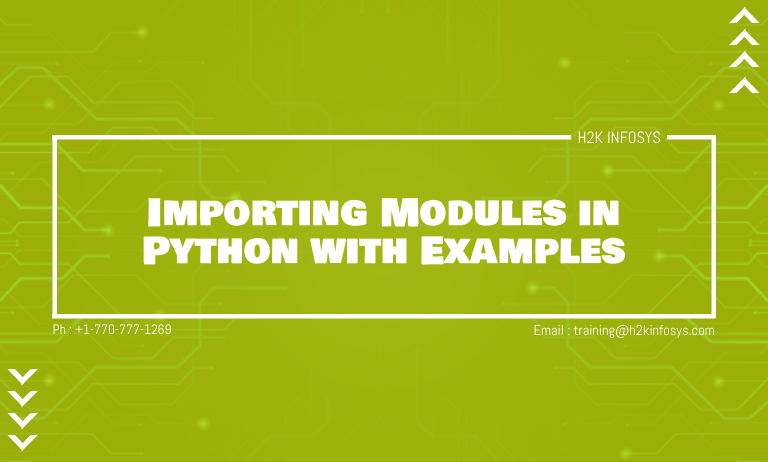
A module is simply a block of code saved as a python file recognized with the .py extension. The codes, in the python file, which could be in functions, classes, or variables can be reused in a different python file. When you want to reuse the code in a python file in another code, you need to import the file as a module. The filename generally becomes the name of the module.
For example, if you want to reuse the codes in a python file saved as app.py, you will have to use the import statement. Thereafter, you can call functions or instantiate objects from the class. In numerous tutorials, you have used the import statement to import external modules such as numpy, json, matplotlib, math, and so on. In this tutorial, you will learn how to create modules and reuse them in another code using the import statement.
In specific terms, you will learn the following by the end of this tutorial
- What are modules in Python?
- How to create a module in python
- Import a Class in Python
- Importing a Module as a Class
- Checking the Attributes from an Imported Module
- Packages in Python
- Using Alias during Import
Let’s get the ball rolling.
What are modules in Python?
As explained in the introduction, modules are simply python files. The functionalities of a python file can be used in another python file by importing the module using the import keyword. This technique holds for other languages such as Javascript, Java, C, Ruby, etc.
How to Create a Module in Python
Step 1
Create a folder to store your codes.
Step 2
Create a python file in this folder. Make sure the file is saved as a .py file. You can use any IDLE for this. Let’s call the file check.py
Step 3
Write some code in the new file
def print_msg(name): print('Hello', name, ', welcome to H2k Infosys!')
Step 4
Create another python file in the folder. Import the base module and call the print_mg() function from the base module.
import base base.print_msg('Ann')
Output:
Hello Ann , welcome to H2k Infosys!
As seen, we were able to use the print_msg function in the base module in the new code by importing it.
Import a Class in Python
Going beyond creating functions, we can also create classes and import classes. Let’s create a class that takes the entities of a student. The student class will take vital classes like name, email, and age.
#create a class that stores student information class Student: def __init__(self, f_name, l_name, age): '''This constructor takes the basic information of the student''' self.f_name = f_name self.l_name = l_name self.email = self.f_name.lower() + self.l_name.lower() + '@gmail.com' self.age = age def display_info(self): '''This function displays the student info''' print(f"This student’s full name is {self.f_name} {self.l_name}.") print(f"Their email address is {self.email}.") print(f"They are {self.age} years old.") def study(self): print(f"{self.f_name} is studying.") def rest(self): print(f"{self.f_name} is resting.")
If you are not sure what a constructor is or how to create a class in Python, please refer to our article on Object-Oriented Programming in python.
Once this class has been created, we can instantiate an object in the class and call different functions in the class. Let’s see some examples.
Let’s create an instance of the class and call it student1.
#create an instance of the class student1 = Student('David', 'Rahul', 23)
We can call all the functions in the class created on this object.
This student’s full name is David Rahul.
Their email address is [email protected].
They are 23 years old.
And it prints the function. Let’s create other functions in the class. Say a function that would print the student is studying and another function that will print that the student is resting.
#create a class that stores student information class Student: def __init__(self, f_name, l_name, age): '''This constructor takes the basic information of the student''' self.f_name = f_name self.l_name = l_name self.email = self.f_name.lower() + self.l_name.lower() + '@gmail.com' self.age = age def display_info(self): '''This function displays the student info''' print(f"This student full name is {self.f_name} {self.l_name}.") print(f"Their email address is {self.email}.") print(f"They are {self.age} years old.") def study(self): print(f"{self.f_name} is studying.") def rest(self): print(f"{self.f_name} is resting.") #create an instance of the class student1 = Student('David', 'Rahul', 23) student1.display_info() print() student1.study() student1.rest()
Output:
This student full name is David Rahul.
Their email address is [email protected].
They are 23 years old.
David is studying.
David is resting.
Importing a Module as a Class
Now let’s say you have another python file that you wish to use the function in the Student class previously created. You do not need to type the entire code again. You only need to import the class as a module. Since we are importing a class from the module, you can either write:
from base import Student or import base.Student
Let’s stick with the first method Next, we can access the functions in the class. Remember first, you must instantiate the class as an object. Afterward, we can run the code.
from base import Student #create an instance of the class student2 = Student('Vic', 'Trent', 27) student2.display_info() print() student2.study() student2.rest()
Output:
This student full name is David Rahul.
Their email address is [email protected].
They are 23 years old.
David is studying.
David is resting.
This student full name is Vic Trent.
Their email address is [email protected].
They are 27 years old.
Vic is studying.
Vic is resting.
If you look at the output, the functions on the first instance ‘student1’ were printed. There are two ways to go around this. You could either delete the functions from the base module before importing or add a condition that if __name == ‘__main__, before calling the functions.
That condition ensures that the functions are only called when the module is run directly and not imported to another model. Let’s add the if __name == ‘__main__ condition to our base model.
#create a class that stores student information class Student: def __init__(self, f_name, l_name, age): '''This constructor takes the basic information of the student''' self.f_name = f_name self.l_name = l_name self.email = self.f_name.lower() + self.l_name.lower() + '@gmail.com' self.age = age def display_info(self): '''This function displays the student info''' print(f"This student full name is {self.f_name} {self.l_name}.") print(f"Their email address is {self.email}.") print(f"They are {self.age} years old.") def study(self): print(f"{self.f_name} is studying.") def rest(self): print(f"{self.f_name} is resting.") if __name__ == '__main__': #create an instance of the class student1 = Student('David', 'Rahul', 23) student1.display_info() print() student1.study() student1.rest()
Now, let’s import the base module in another python script again.
from base import Student #create an instance of the class student2 = Student('Vic', 'Trent', 27) student2.display_info() print() student2.study() student2.rest()
Output:
This student full name is Vic Trent.
Their email address is [email protected].
They are 27 years old.
Vic is studying.
Vic is resting.
Checking the Attributes from an Imported Module
We can also check the attributes of the constructor when you import a class. The coding example below prints the name, email, and age of the instantiated object.
from base import Student #create an instance of the class student2 = Student('Vic', 'Trent', 27) print(student2.f_name) print(student2.l_name) print(student2.email) print(student2.age)
Output:
Vic
Trent
[email protected]
27
Packages in Python
A package in Python is a file directory that has more than one .py file and a special .py file called __init__.py. In the real sense, the __init__.py file is what informs python to treat the files in the directory as a package. In the directory below, even as the folder contains more than one .py file, the folder is not seen as a package
To make this a package, we need to add an __init__.py file. Head over to your VS code and create an empty Python file named __init__.py
Let’s add some functions to the check2.py file
def say_hi(): print('Hi world') def say_hello(): print('Hello world')
Now, we can set to use the files in the folder. Let’s say we wish to import the check to file and access the functions, we can write the following code.
#import the modules from the package from pycodes import check2 #call the functions in module check2.say_hi() check2.say_hello()
Output:
Hi world
Hello world
Using Alias during Import
It is good practice to shorten the python names during import. This way, your code looks cleaner. The shorter name used is called an alias. Conventionally, the pandas module is imported with an alias called pd, seaborn is imported with an alias called sns, and so on.
Let’s say we wish to use the alias ‘chk’ for the check2 module, we use the as keyword.
We can reproduce the last code using the alias, chk.
#import the modules from the package with an alias from pycodes import check2 as chk #call the functions in module chk.say_hi() chk.say_hello()
Output:
Hi world
Hello world
As seen, the code produces the same result.
In summary,
In this tutorial, you have learned what a module is and how to import modules in Python using the import statement. We discussed how to create classes, functions and how to use these functions with Object-Oriented Programming (OOP). This functionality is not only applicable to Python but other languages such as Javascript, Java, etc. Worthy of note is also the use of if __name__ == ‘__main__’ condition. This condition checks whether the function is running from the python file itself or it’s imported.
You also discovered how to create packages in python by creating the __init__.py file in the package directory. Going forward, you learned about using aliases in importing modules in Python. If you’ve got any questions, feel free to leave them in the comment section and I’d do my best to answer them.