In this article, you will study how to select values from dropdown in Selenium WebDriver. Before moving ahead with this tutorial, first let us understand some of the basics of handling drop-downs in Selenium WebDriver.
Select in Selenium WebDriver
In Selenium WebDriver the ‘Select’ class is used for selecting and deselecting option from a dropdown. The select type object can be initialized as parameter by passing the webElement dropdown to its constructor.
WebElement DropDown = driver.findElement(By.id(“testDropdown”));
Select dropdown = new Select(DropDown);
Selecting an option from drop-down menu
Below are the three ways to select an option from the drop-down menu.
- selectByValue
- selectByIndex
- selectByVisibleText
Method Name: selectByValue:
Purpose: This option is used to select a value matching with the given argument by the user.
Syntax: select.selectByValue(Value);
dropdown.selectByValue(“Testing”)
Method Name: selectByIndex:
Purpose: This option is used to select an option based on its index given by the user. There is an attribute called “values” which will have the values index.
Syntax: select.selectByIndex(Index);
dropdown.selectByIndex(4);
Method Name: selectByVisibleText:
Purpose: This option is used to select all the options based on the display text matching the given argument. It will not look for any value or index, it will try to match with the VisibleText.
Syntax: select.selectByVisibleText(Text);
dropdown.selectByVisibleText(“AutomationTesting”);
De-select operations
Method Name: deselectByIndex
Syntax: select.deselectByIndex(Index);
Purpose: This command deselects an option by index
Method Name: deselectByValue
Syntax: select.deselectByValue(Value);
Purpose: This command deselects an option by using “value” attribute”
Method Name: deselectByVisibleText
Syntax: select.deselectByVisibleText(Text);
Purpose: This command deselects an option through its displayed text
Method Name: deselectAll
Syntax: select.deselectAll();
Purpose: This command will deselects all the selected options which are selected previously.
Let’s create a test case in which we will automate the following scenarios to handle Drop-downs:
- Invoke a Google chrome browser.
- Open URL: https://www.facebook.com/
- Select the option “May” month from the drop-down menu.
- Close the browser.
Now, we will create a test case step by step in order to understand of how to handle drop-downs in WebDriver.
Step 1: Launch Eclipse IDE.
Step 2: Go to File > New > Click on Java Project.
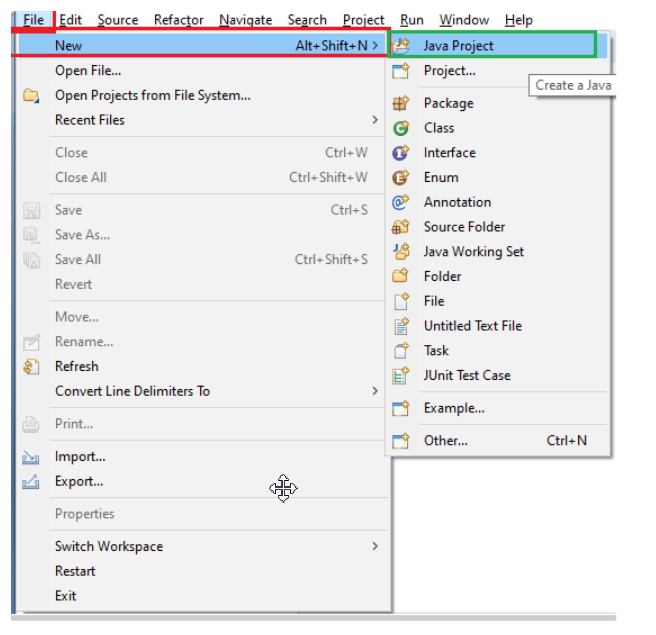
Step 3: Right click on the src folder and click on the New > class.
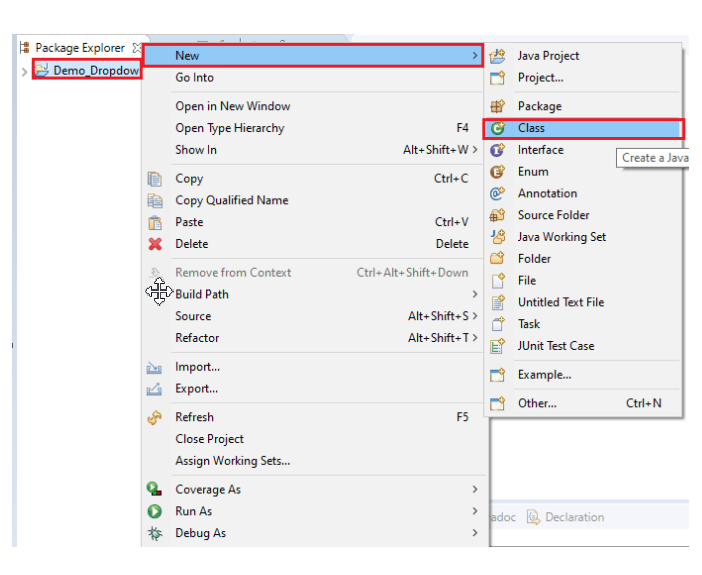
Give your Class name as “Test_Dropdown” and Select the checkbox “public static void main(String[] args) and click on “Finish” button.
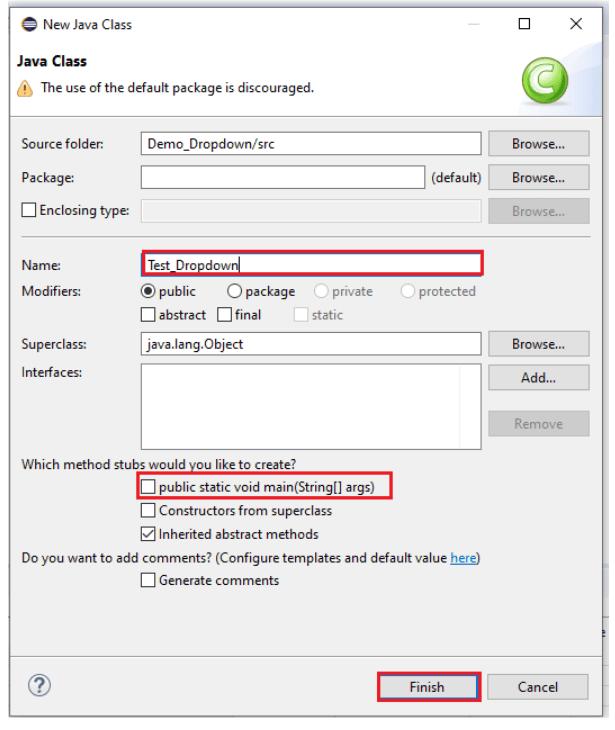
Step 4: Invoke the Google Chrome browser and set the system property to the path of your chromedriver.exe file.
Here is the sample code to set Chrome driver system property:
// System Property for Chrome Driver
System.setProperty("webdriver.chrome.driver", “ D:\\Drivers\\geckodriver.exe ");
After that, we have to initialize the Chrome driver using ChromeDriver Class. Below is the sample code to initialize Chrome driver using ChromeDriver class.
// Instantiate a ChromeDriver class.
WebDriver driver=new ChromeDriver();
We will get the below code to launch the Google Chrome browser after combining both of the above codes.
System.setProperty("webdriver.chrome.driver", “ D:\\Drivers\\geckodriver.exe ");
WebDriver driver=new ChromeDriver();
After that we need to navigate to the desired URL.
Below is the sample code to navigate to the desired URL:
// Launch Website driver.navigate().to("https://www.facebook.com/");
Here is the complete code for above scenario:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class Test_Dropdown {
public static void main(String[] args) {
// System Property for Chrome Driver
System.setProperty("webdriver.chrome.driver", “ D:\\Drivers\\geckodriver.exe ");
// Instantiate a ChromeDriver class.
WebDriver driver=new ChromeDriver();
// Launch Website
driver.navigate().to("https://www.facebook.com/");
}
}
Step 5: Now we will try to locate the drop-down menu.
Follow the below steps to locate the drop-down menu on the web page.
- Open URL: https://www.facebook.com/
- Right click on the drop-down menu on the web page and click on Inspect
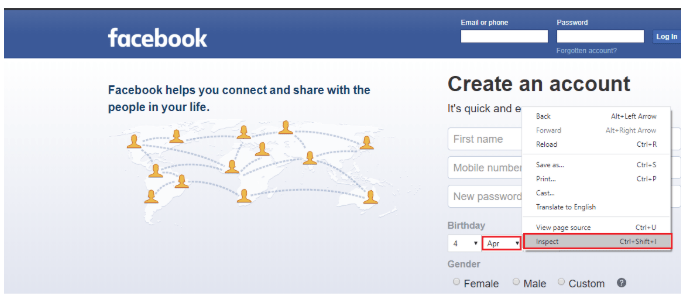
Step 6: Now we try to locate the “Month” dropdown by inspecting its HTML code. It will launch a window containing all the specific codes of the drop-down menu.
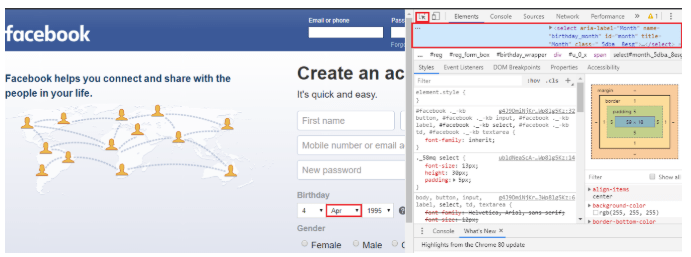
Note the id attribute of a “Month” dropdown.

Step 7: Now we need to write the code which will select the option “May” from the drop-down menu.
Below is the sample code to select the option from the drop-down menu:
//By using Select class for selecting value from dropdown
Select dropdown = new Select(driver.findElement(By.id("month")));
dropdown.selectByVisibleText("May");
Now, our final test script will look something like:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.Select;
public class Test_Dropdown {
public static void main(String[] args) {
// System Property for Chrome Driver
System.setProperty("webdriver.chrome.driver","D:\\ChromeDriver\\chromedriver.exe");
// Instantiate a ChromeDriver class.
WebDriver driver=new ChromeDriver();
// Launch Website
driver.navigate().to("https://www.facebook.com/");
//Use Select class for selecting value from dropdown menu
Select dropdown = new Select(driver.findElement(By.id("month")));
dropdown.selectByVisibleText("May");
// Close the Browser
driver.close();
}
}
Step 8: Right click on the Eclipse and select the option Run As > Java Application.
After running, the above test script will launch the Chrome browser and select the value from the dropdown.
Conclusion:
Element | Command | Description |
Dropdown Box | selectByValue()deselectByValue() | This command selects/deselects an option by using “value” attribute” |
selectByVisibleText()deselectByVisibleText() | This command selects/deselects an option through its displayed text | |
selectByIndex()deselectByIndex() | This command select/deselects an option by index | |
deselectAll() | This command will deselects all the selected options which are selected previously | |
isMultiple() | This command will returns TRUE if the drop-down element allows multiple selection at a time, otherwise it will returns FALSE |
One Response