In this article, we study Selenium WebDriver XPath and learn how to handle various XPath expressions to locate complex or dynamic elements, whose attributes change dynamically whenever the developer makes changes in the code.
In Selenium, if elements are not found with general locators like id, name, class, linkText, partialLinkText then XPath is used to find an element on the webpage.
What is XPath?
XPath is called as XML Path. It is a query language or syntax used for navigating to any element on the web page through XML documents. It is one of the important strategies to locate elements in selenium. XPath expressions is used to locate any element on a webpage using HTML DOM structure.
The basic syntax of XPath is explained with below screenshot.
Syntax for XPath
The standard syntax of an Selenium XPath is
[box type=”shadow” align=”” class=”” width=””]//tag[@atrributeName=’attributeValues’][/box]
- //: It is used to choose the current node
- tagname: It is the tagname of the particular node
- @: It is used for selection of an attribute
- Attribute: It is the attribute name of a node
- Value: It defines the attribute value
There are different locators to find the element on the web pages
Locators | Description |
Id | Finds the element by ID of the element on a webpage |
Classname | Finds the element by Classname of the element on a webpage |
Name | Finds the element by name of the element on a webpage |
LinkText | Finds the element by link text of the element on a webpage |
XPath | XPath used for finding dynamic elements on a webpage and traverse among various elements |
CSSSelector | It locates elements on a webpage which have no id, name or class |
Types of XPath
There are two types of XPath expressions
- Absolute XPath
- Relative XPath
Absolute XPath
It is the direct way to find the element on the webpage. Absolute XPath uses full path starting from root element to the desired UI element. The main disadvantage of Absolute XPath is that if developer makes any changes in the path of the element then our written XPath gets failed. The main advantage with absolute XPath is that it identifies the element very fast.
The characteristic of XPath expression is that it begins with the single forward slash(/), which means that it starts the selection from the root node.
The example of an absolute XPath of an element is shown below
[box type=”shadow” align=”center” class=”” width=””]/html/head/body/table/tbody/tr/th[/box]
If the developer adds a tag between the body and table.
[box type=”shadow” align=”center” class=”” width=””]/html/head/body/form/table/tbody/tr/th[/box]
The first path will not work as “form” tag is added between body and a table
Relative XPath
A relative XPath begin by referencing from the middle of the HTML DOM structure. It starts with the double forward-slash(//). It doesn’t need to start from the root node, which means it starts searching for the element anywhere at the webpage.
The example of an relative XPath of an element is shown below
[box type=”shadow” align=”center” class=”” width=””]//input[@id=’email’][/box]
Now, let’s consider an example
Let’s launch Google chrome and navigate to google.com
Here, by using an XPath we will try to locate the search bar. On inspecting the search bar web element you can see it having an input tag and attributes like class and id. Now, by using the tag name and attributes you can generate the XPath which will locate the search bar.
Here, press Ctrl + F and click Elements tab to open a search box in developer’s chrome tool. Based on this criteria you can write XPath to search for the web element. From the above image, you can see it has an input tag and you can start writing with //input which implies tag name. Now, we use the attribute
name and pass the name attribute ‘q’ as its value in single quotes. This shows XPath as below:
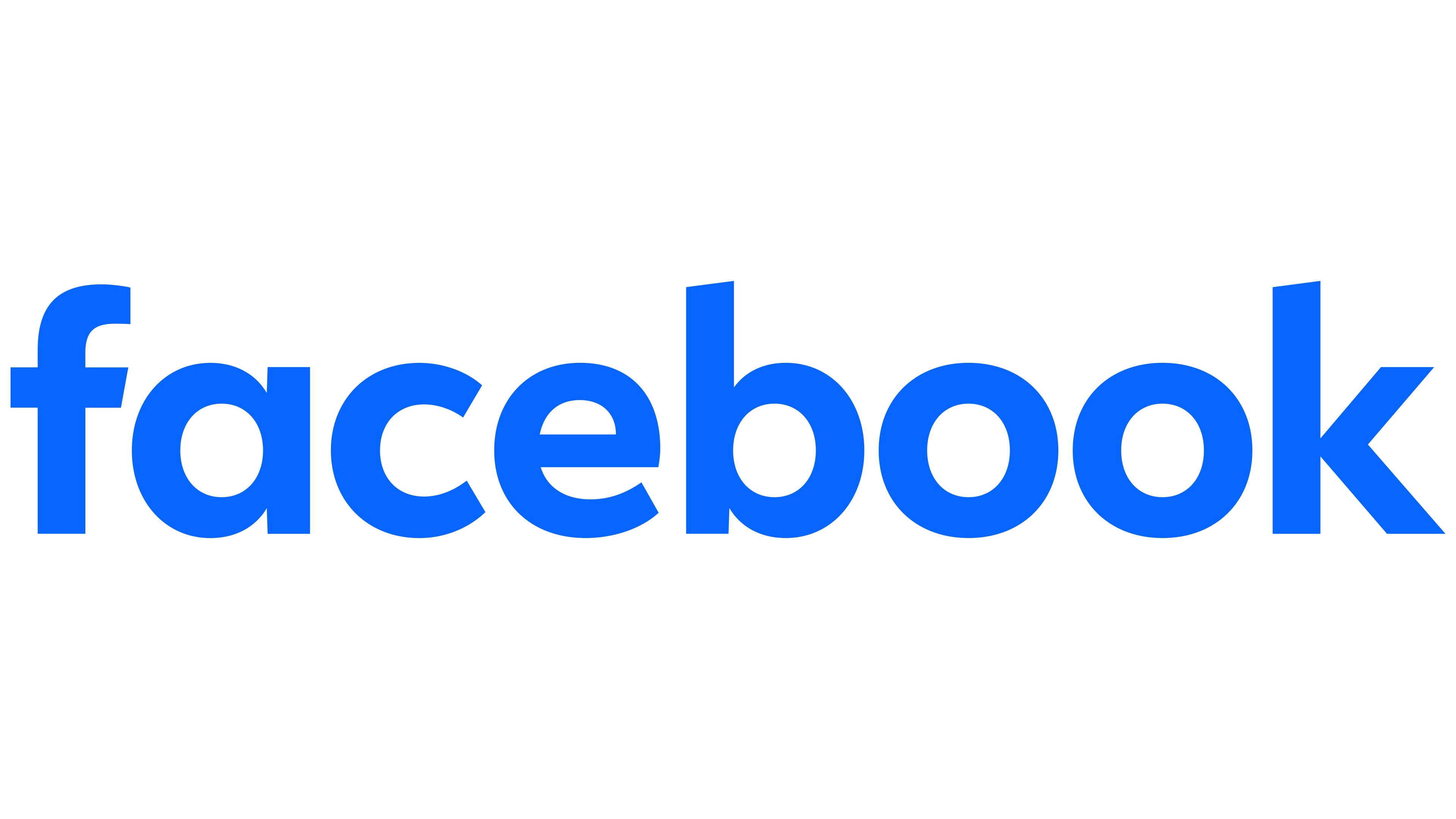
How to generate XPath
From the above image you can see that the element got highlighted on writing on XPath which shows that particular element was located using XPath.
Generally, we generate the XPath in two different ways i.e manually and by inbuilt utilities. But sometimes the HTML file is quite large or complex and writing the XPath manually each time would be a quite difficult task. In such cases, there are certain inbuilt utilities which can help us.
Chrome Browser has Inbuilt utility to inspect and generate the XPath.
Example:
In below example, we login to Facebook application by entering Email, Password and by clicking Log In button by opening Chrome browser.
Right-click on Email or Phone, and select Inspect to inspect the Email or phone web element.
It will open HTML DOM structure once inspecting the Email or Phone.
Right click on HTML DOM structure > Select Copy and Click on Copy XPath to get the XPath of Email or Phone
In the above case, the XPath is
Right-click on Password field and select Inspect to inspect the Password web element.
It will open HTML DOM structure once inspecting the Password field.
Right click on HTML DOM structure > Select Copy and Click on Copy XPath to get the XPath of Password field.
In the above case, the XPath is
Right-click on Log In button and select Inspect to inspect Log In button
It will open HTML DOM structure once inspecting the Log In button.
Right click on HTML DOM structure > Select Copy and Click on Copy XPath to get the XPath of Log In button.
In the above case, the XPath is
Now, our final test script will look something like:
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class RelativeXPathTest { public static void main(String[] args) { // TODO Auto-generated method stub System.setProperty("webdriver.chrome.driver", "D:\\Drivers\\geckodriver.exe "); WebDriver driver= new ChromeDriver(); driver.get("https://www.facebook.com/"); driver.manage().window().maximize(); //XPath for Email Field driver.findElement(By.xpath("//*[@id='email']")).sendKeys("[email protected]"); //XPath for Password Field driver.findElement(By.xpath("//*[@id='pass']")).sendKeys("xxxxxxx"); //XPath for Log In button driver.findElement(By.xpath("//*[@id=\"u_0_a\"]")).click(); } }
XPath Functions
Automation using Selenium has many ways to identify an element on a web page. Sometimes elements on a web page have same attributes at that time we face problem in identifying the element. Some cases can be elements having the same names and attributes or more than one button with same ids and name. In such cases, it’s challenging to identify a particular object and that is where XPath comes into the picture.
Different Types of XPath Functions
Below are the three XPath functions which are used mostly
- contains()
- text()
- starts-with()
- And/OR
contains()
Contains() function is widely used in XPath. This method is used when the value of any attribute changes dynamically. With available partial text we can locate a web element on the web page by using this method.
Examples of contains() method.
- Xpath=.//* [contains (@id, ‘button’)]
- Xpath=//*[contains(@name, ‘login’)]
- Xpath=//*[contains(text (),’automationtesting’)]
- Xpath=//*[contains (@href,’https://www.google.com’)]
- Xpath=//*[contains (@type, ‘sub-type’)]
- //span[contains(text(),'( Username : Admin | Password : qwerty )’)]
- //a[contains(text(),’message?’)]
text()
This function is used to locate an element on a web page with an exact text.
Examples of text() method.
- //span[text()='( Username : Admin | Password : admin123 )’]
Starts-with()
This function can be used when starting partial attribute value associated with the web element.
- //div[starts-with(@id,message)]
And/OR
expression is used when want to join two conditions together, and based on whether they are true or false.
- //input[@name=’txtpassword’ or @id=’txtpassword’]
5 Responses
Hey
Just finished reading your Selenium WebDriver XPath tutorial. It’s awesome! Clear explanations, helpful examples, and great tips. I just like to add more information for Xpath by sharing the blog
Thanks for sharing your expertise