Understanding Python Lists
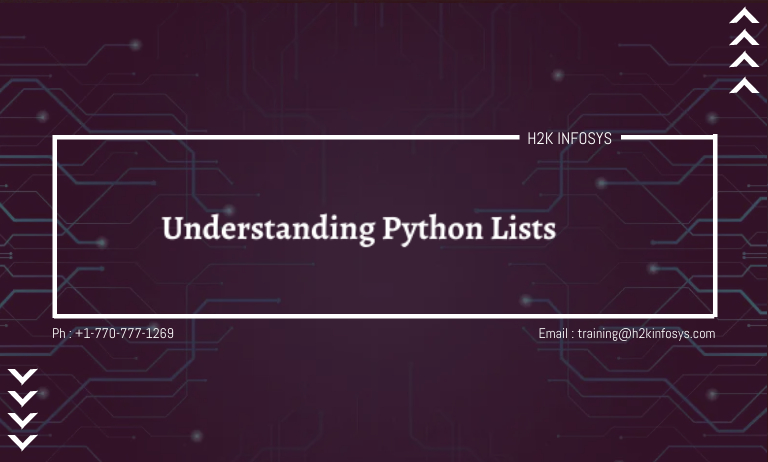
Lists are considered as dynamically sized arrays that will be used which are utilized in another language. Lists don’t need to be homogeneous always, which makes it the most powerful tool in python. A single list consists of data types like integers, strings, and objects. Lists are mutable and can be altered even after their creation.
Lists in python will be ordered and have a definite count. The elements in a list are indexed as per definite sequence and indexing of a list is done with 0 being the first index. Each element in the list has its definite place in the list where it is allowed to duplicate elements in a list with each element which is having its own distinct place and also credibility.
Creating a list
The lists in python can be built by just placing sequencing the square brackets[]. Lists have mutable elements. Consider the example
Python program to demonstrate # Creating a ListList = []print(“Blank List: “) print(List) # Creating a List of numbers List = [10, 20, 14]print(“\nList of numbers: “) print(List) # Creating a List of strings and accessing # using index List = [“Hello”, “For”, “people”]print(“\nList Items: “) print(List[0])print(List[2]) # Creating a Multi-Dimensional List # (By Nesting a list inside a List) List = [[‘Hello’, ‘For’], [‘people’]]print(“\nMulti-Dimensional List: “) print(List) |
Output:
Blank List:
[]List of numbers:
[10, 20, 14]List Items
Hello
people
Multi-Dimensional List:
[[‘Hello’, ‘For’], [‘people’]]Adding the elements to the list
By using the append() method
The elements that may be added to the list using the built-in append() function. Only one element that time added to the list by using the append method for any addition of multiple elements with append() method, loops will be used, Tuples that can also be included to the list with the use of the append method because the tuples are immutable. Lists that can be added to the existing list with the use of the append() method. For example
Python program to demonstrate # Addition of elements in a List # Creating a List List = []print(“Initial blank List: “) print(List) # Addition of Elements in the List List.append(1) List.append(2) List.append(4) print(“\nList whereafter Addition of Three elements: “) print(List) # Adding elements to the List # using Iterator for i in range(1, 4): List.append(i) print(“\nList whereafter Addition of elements from 1-3: “) print(List) # Adding Tuples to the List List.append((6,5)) print(“\nList whereafter Addition of a Tuple: “) print(List) # Addition of a ListL ist2 = [‘For’, ‘people’]List.append(List2) print(“\nList whereafter Addition of a List: “) print(List) |
Output:
Initial blank List:
[]List whereafter Addition of Three elements:
[1, 2, 4]List whereafter Addition of elements from 1-3:
[1, 2, 4, 1, 2, 3]List whereafter Addition of a Tuple:
[1, 2, 4, 1, 2, 3, (6,5)]List whereafter Addition to List:
[1, 2, 4, 1, 2, 3, (6, 5), [‘For’, ‘people’]]By using the insert() method
The append () method does the addition of elements at the last end of the list, for the addition of elements at the required position, the insert() method is used. Append() which takes only one argument, the insert() method requires two arguments(position,value). For example
Python program to demonstrate # Addition of elements in a List # Creating a List List = [1,2,3,4]print(“Initial List: “) print(List) # Addition of Element at specific Position # (By using Insert Method) List.insert(3, 12) List.insert(0, ‘people’) print(“\nList whereafter performing Insert Operation: “) print(List) |
Output:
Initial List:
[1, 2, 3, 4]List whereafter performing Insert Operation:
[‘people’, 1, 2, 3, 12, 4]Using the extend () method
Apart from append() and insert() method there’s one more method we have for the addition of elements, extend(), this method will be used to add the multiple elements at the end.
For example Python program to demonstrate # Addition of elements in a List # Creating a List List = [1, 2, 3, 4]print(“Initial List: “) print(List) # Addition of multiple elements # to the List at the end # (using Extend Method) List.extend([8, ‘people’, ‘Always’]) print(“\nList whereafter performing Extend Operation: “) print(List) |
Output:
Initial List:
[1, 2, 3, 4]List whereafter performing Extend Operation:
[1, 2, 3, 4, 8, ‘people’, ‘Always’]Questions
- What are Lists in Python how it is used?
2. Explain the insert method with an example?
1) What are Lists in Python how it is used?
A list is a collection of similar or different types of data.
For example,
Suppose we need to record the age of 5 students. Instead of creating 5 separate variables, we can simply create a list:
17,18,15,19,14
List of Age
Elements of a list
A list is created in Python by placing items inside [], separated by commas . For example,
# A list with 3 integers
numbers = [1, 2, 5]
print(numbers)
# Output: [1, 2, 5]
2) Explain the insert method with an example?
Python insert() method inserts the element at the specified index in the list.
Example:
cities = [‘Mumbai’, ‘London’, ‘Paris’, ‘New York’]
cities.insert(0, ‘New Delhi’) # inserts at 0 index (first element)
print(cities)
cities.insert(2, ‘Chicago’) # inserts at 2nd index
print(cities)
Output
[‘New Delhi’, ‘Mumbai’, ‘London’, ‘Paris’, ‘New York’]
[‘New Delhi’, ‘Mumbai’, ‘Chicago’, ‘London’, ‘Paris’, ‘New York’]
1.What are Lists in Python how it is used?
-The list is an inbuilt data structure in Python used to store and use data items collectively and efficiently. Formally, a list can be defined as a group of data items referenced under a common name. Lists have the extra benefit of being dynamic in size, which means that, you don’t have to specify the size of lists beforehand, size is determined by the elements created in the list. Lists show benefits like, it can hold data items with different data types
example: # Creating a List of numbers
List = [10, 20, 14]print(“\nList of numbers: “)
print(List)
output : List of numbers:
[10, 20, 14]
2.Explain the insert method with an example?
-For the addition of elements at the required position, the insert() method is used.The insert() method requires two arguments(position,value).
For example: Python program to demonstrate
# Addition of elements in a List
# Creating a List
List = [1,2,3,4]print(“Initial List: “)
print(List)
# Addition of Element at specific Position
# (By using Insert Method)
List.insert(3, 12)
List.insert(0, ‘people’)
print(“\nList whereafter performing Insert Operation: “)
print(List)
Output:
Initial List:
[1, 2, 3, 4]
List whereafter performing Insert Operation:
[‘people’, 1, 2, 3, 12, 4]
The lists in python can be built by just placing sequencing the square brackets[]. Lists have mutable elements. Consider the example
Python program to demonstrate
# Creating a ListList = []print(“Blank List: “)
print(List)
# Creating a List of numbers
List = [10, 20, 14]print(“\nList of numbers: “)
print(List)
# Creating a List of strings and accessing
# using index
List = [“Hello”, “For”, “people”]print(“\nList Items: “)
print(List[0])print(List[2])
# Creating a Multi-Dimensional List
# (By Nesting a list inside a List)
List = [[‘Hello’, ‘For’], [‘people’]]print(“\nMulti-Dimensional List: “)
print(List)
Output:
All IT Courses 50% Off
Blank List:
[]
List of numbers:
[10, 20, 14]
List Items
Hello
people
Multi-Dimensional List:
[[‘Hello’, ‘For’], [‘people’]]
Adding the elements to the list
By using the append() method
The elements that may be added to the list using the built-in append() function. Only one element that time added to the list by using the append method for any addition of multiple elements with append() method, loops will be used, Tuples that can also be included to the list with the use of the append method because the tuples are immutable. Lists that can be added to the existing list with the use of the append() method.
By using the insert() method
The append () method does the addition of elements at the last end of the list, for the addition of elements at the required position, the insert() method is used. Append() which takes only one argument, the insert() method requires two arguments(position,value). For example
Python program to demonstrate
# Addition of elements in a List
# Creating a List
List = [1,2,3,4]print(“Initial List: “)
print(List)
# Addition of Element at specific Position
# (By using Insert Method)
List.insert(3, 12)
List.insert(0, ‘people’)
print(“\nList whereafter performing Insert Operation: “)
print(List)
Output:
Initial List:
[1, 2, 3, 4]
List whereafter performing Insert Operation:
[‘people’, 1, 2, 3, 12, 4]
Using the extend () method
Apart from append() and insert() method there’s one more method we have for the addition of elements, extend(), this method will be used to add the multiple elements at the end.
For example
Python program to demonstrate
# Addition of elements in a List
# Creating a List
List = [1, 2, 3, 4]print(“Initial List: “)
print(List)
# Addition of multiple elements
# to the List at the end
# (using Extend Method)
List.extend([8, ‘people’, ‘Always’])
print(“\nList whereafter performing Extend Operation: “)
print(List)
Understanding Python lists:
What are Lists in Python and how is it used?
One of the most powerful tools in Python is Lists. A single list may consist of data types like integers, strings, and objects. Lists are mutable and can be altered even after their creation.
Creating a list:
The lists in python can be built by just placing the sequence in the square brackets[].
For our understanding: consider list as follows
List = [1, 2, 3, 4, 5]
List[0] = 1
list[1] = 2
list[2] = 3
list[3] = 4
list[4] = 5
List[0:] = 1, 2, 3, 4, 5
List[:] = 1, 2, 3, 4, 5
List[1:4] = 2, 5
List[0:3] = 1, 4
# Creating a List of numbers
List = [23,45,78]print(“\nList of numbers: “)
print(List)
Result = 23, 45, 78
Explain the insert method with an example?
To add the addition of elements at the required position, we use the ‘insert()’ method which has two arguments namely position and value whereas the append () method does adding the elements only at the last end of the list.
# Addition of elements in a List:
# Creating a List
List = [A,B,D,E]print(“Initial List: “)
print(List)
# Addition of Element at specific Position (By using Insert Method)
List.insert(3, C)
List.insert(0, ‘Alphabet=’)
print(“\nList whereafter performing Insert Operation: “)
print(List)
# Output:
Initial list : [A,B,D,E]
List after insert :[‘Alphabet=’, A,B,C,D]
A.Lists are considered as dynamically sized arrays that will be used which are utilized in another language. Lists don’t need to be homogeneous always, which makes it the most powerful tool in python. A single list consists of data types like integers, strings, and objects. Lists are mutable and can be altered even after their creation.
Lists in python will be ordered and have a definite count. The elements in a list are indexed as per definite sequence and indexing of a list is done with 0 being the first index. Each element in the list has its definite place in the list where it is allowed to duplicate elements in a list with each element which is having its own distinct place and also credibility.
B.By using the insert() method
The append () method does the addition of elements at the last end of the list, for the addition of elements at the required position, the insert() method is used. Append() which takes only one argument, the insert() method requires two arguments(position,value). For example
Python program to demonstrate
# Addition of elements in a List
# Creating a List
List = [1,2,3,4]print(“Initial List: “)
print(List)
# Addition of Element at specific Position
# (By using Insert Method)
List.insert(3, 12)
List.insert(0, ‘people’)
print(“\nList whereafter performing Insert Operation: “)
print(List)
Output:
Initial List:
[1, 2, 3, 4]
List whereafter performing Insert Operation:
[‘people’, 1, 2, 3, 12, 4]
Using the extend () method
Lists in Python how it is used ?
Lists are considered as dynamically sized arrays that will be used which are utilized in another language. Lists don’t need to be homogeneous always, which makes it the most powerful tool in python. A single list consists of data types like integers, strings, and objects. Lists are mutable and can be altered even after their creation.
Lists in python will be ordered and have a definite count. The elements in a list are indexed as per definite sequence and indexing of a list is done with 0 being the first index. Each element in the list has its definite place in the list where it is allowed to duplicate elements in a list with each element which is having its own distinct place and also credibility.
1. What are Lists in Python how it is used?
Lists in python will be ordered and have a definite count. The elements in a list are indexed as per definite sequence and indexing of a list is done with 0 being the first index. Each element in the list has its definite place in the list where it is allowed to duplicate elements in a list with each element which is having its own distinct place and also credibility. The lists in python can be built by just placing sequencing the square brackets[]. Lists have mutable elements.
2. Explain the insert method with an example?
For the addition of elements at the required position, the insert() method is used. Append() which takes only one argument, the insert() method requires two arguments(position,value). For example
Python program to demonstrate
# Addition of elements in a List
# Creating a List
List = [1,2,3,4]print(“Initial List: “)
print(List)
# Addition of Element at specific Position
# (By using Insert Method)
List.insert(3, 12)
List.insert(0, ‘people’)
print(“\nList whereafter performing Insert Operation: “)
print(List)
Output:
Initial List:
[1, 2, 3, 4]
List whereafter performing Insert Operation:
[‘people’, 1, 2, 3, 12, 4]
1.Lists are dynamically sized arrays that will be used and utilized in another language. A list can have data types like strings, integers, and objects. They are mutable and can be altered. They have an order and definite count. Each element in the list has its definite place in the list where you can duplicate elements and each element has its own distinct place and credibility. Example
Python program to demonstrate
# Creating a ListList = []print(“Blank List: “)
print(List)
# Creating a List of numbers
List = [10, 20, 14]print(“\nList of numbers: “)
print(List)
# Creating a List of strings and accessing
# using index
List = [“Hello”, “For”, “people”]print(“\nList Items: “)
print(List[0])print(List[2])
# Creating a Multi-Dimensional List
# (By Nesting a list inside a List)
List = [[‘Hello’, ‘For’], [‘people’]]print(“\nMulti-Dimensional List: “)
print(List)
2. Append() method will add elements at the end of the list and insert() method will add elements at the required position. Append will take 1 argument and insert will take 2 arguments (position, value). Example
Python program to demonstrate
# Addition of elements in a List
# Creating a List
List = [1,2,3,4]print(“Initial List: “)
print(List)
# Addition of Element at specific Position
# (By using Insert Method)
List.insert(3, 12)
List.insert(0, ‘people’)
print(“\nList whereafter performing Insert Operation: “)
print(List)
1. What are Lists in Python how it is used?
Lists are used to store multiple items in a single variable. Lists are one of 4 built-in data types in Python used to store collections of data, the other 3 are Tuple, Set, and Dictionary, all with different qualities and usage. The list is a most versatile datatype available in Python which can be written as a list of comma-separated values (items) between square brackets. Important thing about a list is that items in a list need not be of the same type.
How it is used: Creating a list is as simple as putting different comma-separated values between square brackets.
For Example:
# empty list with square brackets
empty_list = []
# empty list with square brackets
empty_list = list()
# list of integers
integer_list = [1, 2, 3]
# list with mixed data types
mixed_list = [8, “Hi”, 3.3]
# list from an iterable object
iterable_list = list(“data-science”)
# a nested list
nested list = [“cat”, [3, 2], [‘d’]]
2. Explain the insert method with an example?
Inserts a new item before a specified item in a combo box or selection list. The new item can consist of a label, a list of labels, or a label-value pair. insert() Function is a Python library function that is used to insert the given element at a particular index in a list.
Example:
Insert the value “orange” as the second element of the fruit list:
fruits = [‘apple’, ‘banana’, ‘cherry’]
fruits.insert(1, “orange”)
1) What are Lists in Python how it is used?
A) In Python, a list is a collection of values that are ordered and changeable. It allows you to store multiple items of different data types in a single variable. Lists are one of the most commonly used data structures in Python and are very versatile.
Python program to demonstrate
# Creating a ListList = []print(“Blank List: “)
print(List)
# Creating a List of numbers
List = [10, 20, 14]print(“\nList of numbers: “)
print(List)
# Creating a List of strings and accessing
# using index
List = [“Hello”, “For”, “people”]print(“\nList Items: “)
print(List[0])print(List[2])
# Creating a Multi-Dimensional List
# (By Nesting a list inside a List)
List = [[‘Hello’, ‘For’], [‘people’]]print(“\nMulti-Dimensional List: “)
print(List)
2) Explain the insert method with an example?
A) The insert() method is a built-in method in Python that allows you to add an element to a specific index position in a list. Here’s how the method works:
my_list = [1, 2, 3, 4, 5]
# Inserting a new element at index 2
my_list.insert(2, “new”)
print(my_list)
# Output: [1, 2, “new”, 3, 4, 5]
In this example, we create a list called my_list that contains five integers. We then use the insert() method to add a new element (“new”) at index position 2 in the list. The first argument to the insert() method specifies the index position where the new element should be added, and the second argument specifies the value of the new element.
After calling insert(), the new element is added to the list at the specified index position, and all the existing elements are shifted to the right. In this case, the “new” element is added at index position 2, and the existing elements at positions 2-4 are shifted to the right to make room for it.