Cross Browser Testing using Selenium WebDriver
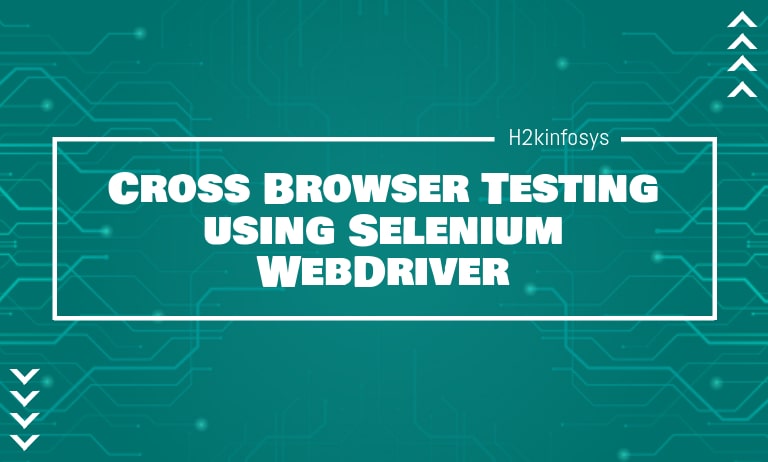
What is Cross Browser Testing?
Cross Browser Testing in Selenium is a type of functional testing to verify that your web application works across different browsers as expected. It is the process to verify that your application’s compatibility with different browsers.
Introduction
Sometimes we observed that on some browsers some websites are not properly displayed and we think that the website is broken. But, as soon as you open the same website on a different browser, the website opens properly. Thus this explains the compatibility of a website with different browsers. Each browser interprets the information on the website differently. Thus, some browsers may lack the features of the website and make your website look broken on that browser.
With the advanced technology, there are several options available for browsers, and it’s not just enough that our website should work on one of the browsers. To access your application users should not be restricted to use any specific browser. Thus, it is necessary to test the compatibility of your website with different browsers. Some of the most commonly used browsers are Chrome, Firefox, Safari, Opera, Internet Explorer, etc.
Why do we need Cross Browser Testing?
Web-based applications are different from Windows-based applications. An end-user can open a web application in any browser. For example, some people prefer to open https://www.facebook.com in the Chrome browser, while others can be using the Firefox browser or IE.
So we make sure that the web application will work as expected in all popular browsers so that many people can access it and use it. This can be fulfilled with Cross Browser Testing of the product.
Reason for Cross-Browser Issues
- Different JavaScript implementation
- Mismatch of font size in different browsers
- No support of HTML5 in some browsers
- Differences in CSS, and HTML validation
- Page alignment and div size
- Incompatibility with Operating System.
How to perform Cross Browser Testing
We can automate test cases using Firefox, Internet Explorer, Chrome, and Safari browsers in selenium web driver.
We integrate TestNG framework with Selenium WebDriver to execute test cases with different browsers in the same machine at the same time.
Your testing.xml will look like
This test.xml will map with the Test Case which will look like
Here the test.xml has two Test tags (‘FirefoxTest’, ‘ChromeTest’), this test case will execute two times for 2 different browsers.
First Test ‘FirefoxTest’ will pass the value of parameter ‘browser’ as ‘Firefox’ so FirefoxDriver will be executed. On the Firefox browser, this test case will run.
Second Test ‘ChromeTest’ will pass the value of parameter ‘browser’ as ‘Chrome’ so ChromeDriver will be executed. On the Chrome browser, this test case will run.
Complete Code:
CrossBrowserExample.java
package parallelTesting; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.ie.InternetExplorerDriver; import org.testng.annotations.BeforeTest; import org.testng.annotations.Parameters; import org.testng.annotations.Test; public class CrossBrowserExample { WebDriver driver; @BeforeTest @Parameters("browser") public void setup(String browser) throws Exception{ //Check if parameter passed from TestNG is 'firefox' if(browser.equalsIgnoreCase("firefox")){ //create firefox instance System.setProperty("webdriver.gecko.driver","F:\\drivers\\geckodriver.exe"); driver = new FirefoxDriver(); } //Check if parameter passed as 'chrome' else if(browser.equalsIgnoreCase("chrome")){ //set path to chromedriver.exe System.setProperty("webdriver.chrome.driver","F:\\drivers\\chromedriver.exe"); //create chrome instance driver = new ChromeDriver(); } //Check if parameter passed as 'Edge' else if(browser.equalsIgnoreCase("IE")){ //set path to Edge.exe System.setProperty("webdriver.ie.driver","F:\\drivers\\IEDriverServer.exe"); //create Edge instance driver = new InternetExplorerDriver(); } else{ //If no browser passed throw exception throw new Exception("Browser is not correct"); } driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); } @Test public void testParameterWithXML() throws InterruptedException{ driver.get("https://www.facebook.com/"); //Find user name WebElement Email = driver.findElement(By.name("email")); //Fill user name Email.sendKeys("[email protected]"); //Find password WebElement password = driver.findElement(By.name("pass")); //Fill password password.sendKeys("xxxxxx"); } }
test.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd"> <suite name="TestSuite" thread-count="2" parallel="tests" > <test name="ChromeTest"> <parameter name="browser" value="chrome" /> <classes> <class name="parallelTesting.CrossBrowserExample"> </class> </classes> </test> <test name="FirefoxTest"> <parameter name="browser" value="firefox" /> <classes> <class name="parallelTesting.CrossBrowserExample"> </class> </classes> </test> <test name="IETest"> <parameter name="browser" value="IE" /> <classes> <class name="parallelTesting.CrossBrowserExample"> </class> </classes> </test> </suite>
Run the test by right-clicking on the test.xml > select Run As, and Click TestNG Suite.
Conclusion:
- Cross Browser Testing in selenium is a type of functional testing to verify that your web application works across different browsers as expected.
- For automation selenium support different type of browsers.
- To perform Multi-Browser Testing selenium can be integrated with TestNG.
One Comment