Python Numpy Array Tutorial: A Beginners Guide
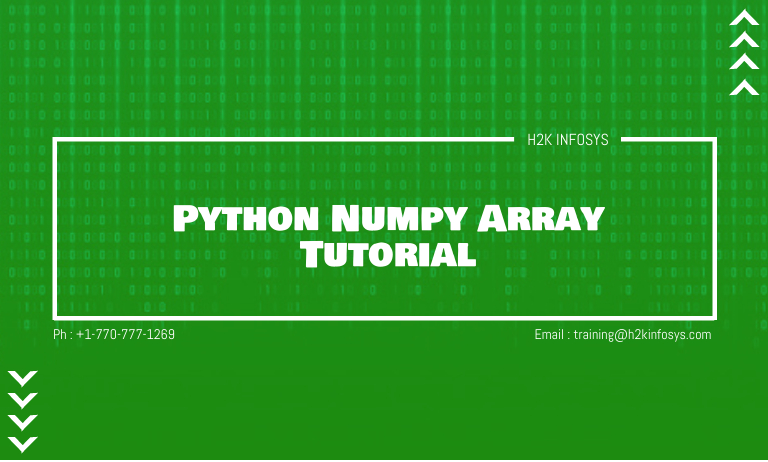
In this tutorial, we will discuss Python Numpy Arrays. While datasets come in different formats such as texts, images, audios, etc, they can all be seen as arrays of numbers. For example, a document can be seen as an array of vectors based on the frequency of the words or similarity of one to another. Images can be seen as a 2-dimensional array of the intensity of the pixels. Audios can be seen as a 1-dimensional array of the sound pitch as time progresses. Generally speaking, all forms of data can be converted into Numpy array, and thus it is vital to understand how to work with them.
The NumPy library is a robust library that allows for data manipulation in arrays. Arrays in NumPy are called ndarrays. If you are wondering why arrays and not list? Well, Python Numpy arrays are way faster than lists in mathematical computations and they are also easier to manage. This tutorial will focus on how to create NumPy arrays and check for some of their key properties.
How to create a NumPy array.
A NumPy array can be created by calling the array() method. The argument received is typically a list. When the array() is called, the list passed is converted to a Numpy array, whose data type is called ndarray. See the example below.
import numpy as np numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9] print(type(numbers)) numbers = np.array(numbers) print(type(numbers))
Output:
<class 'list'>
<class 'numpy.ndarray'>
As seen, the numbers were first of type list but calling the array() method on them converts them to a NumPy arr
Performing Basic Mathematical Operations on a NumPy Array
NumPy arrays are capable of performing basic mathematical operations such as addition, subtraction, multiplication, and division. Given that the arrays are of the same length, the operations will be performed based on the elements of the arrays. For instance, the computation of [1, 2] + [2, 1] returns [3, 3]. See the coding example below for the four basic operations.
import numpy as np number1 = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9]) number2 = np.array([2, 4, 5, 2, 6, 2, 5, 2, 8]) print(number1 + number2) print() print(number1 - number2) print() print(number1 * number2) print() print(number1 / number2)
Output:
[ 3 6 8 6 11 8 12 10 17]
[-1 -2 -2 2 -1 4 2 6 1]
[ 2 8 15 8 30 12 35 16 72]
[0.5 0.5 0.6 2. 0.83333333 3.
1.4 4. 1.125 ]
Finding the shape of an array
One important property of an array is its shape. The shape of an array is simply the number of elements horizontally by the number of elements vertically. To check the shape of an array, the shape attribute is used.
import numpy as np number = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9]) print(number.shape)
Output:
(9,)
The result shows that the array is a 1-dimensional array with 9 elements on the x-axis and 1 element on the y axis. Going forward in this series, we will discuss how to create a 2-dimensional array and check its shape.
Finding the DataTypes of the Elements of an Array
Another important property of an array is the datatype of the array. You can find the datatype which the elements of the array are of, using the dtype attribute. An array that contains only integers has a dtype, int. Meanwhile, you can specify the dtype of an array using the dtype parameter when the array was created.
import numpy as np number1 = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9]) number2 = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9], dtype='float64') #check the datatype of the arrays print(number 1.dtype) print(number2.dtype)
Output:
int32
float64
As seen, while the dtype of the first array is an int, the dtype of the second is a float. Printing the array to the console makes it clearer.
print(number2)
Output:
[1. 2. 3. 4. 5. 6. 7. 8. 9.]
From the result, observe that the integers were converted to floating-point numbers. That’s it for this tutorial. If you have any questions, feel free to leave them in the comment section and I’d do my best to answer them.
2 Comments