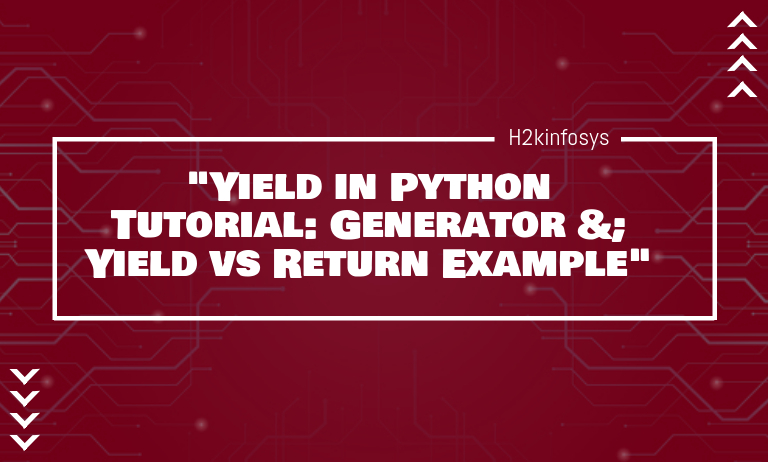
While working with a large amount of data the programmer sometimes needs to control the function’s start and stop state. The Yield gives the programmer the power to execute the program the way he/she wants rather than computing them at once. Let’s take a look at an example. Suppose you have a function that prints “Hello World” 5 times and functions that Yields “Hello World” five times. Let’s take a look at the difference between Yield and simple return for Yield in Python.
def normal(): |
Take a look at the above output. The normal function gives us back 5 print statements but the yielding function gives a generator.
Now we need to loop over the generator to get the output.
def yielding(): |
How to read the values from the generator?
Let’s take a look at different ways to read values from generators.
Using : list()
We can use the list function to generate all the output and store it in a list.
def even_num(x): |
Using : for-loop
In Yield in Python, We used for loop to iterate over the generator in the first example. Let’s take another example.
def even_num(x): |
Using next()
There is a built-in function next which is used to generate the next number from generator. After generating the last item it will give an error.
def even_num(x): |