ASP.NET Validators
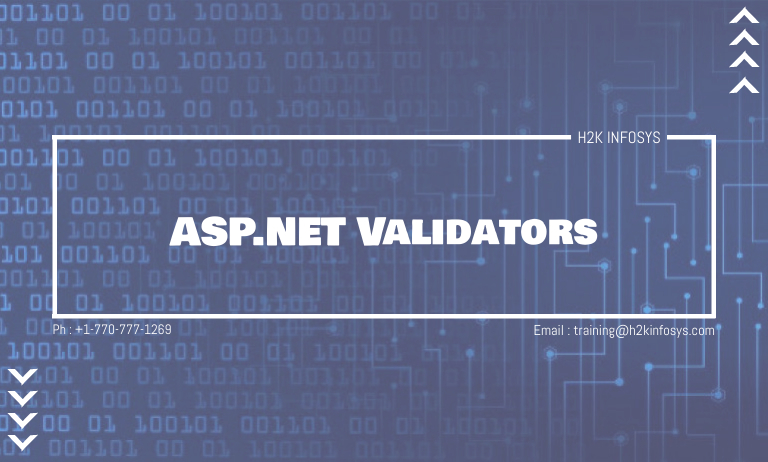
ASP.NET provides controls for performing validation that automatically checks user input and requires no code. You can also create custom validation for your application.
Following are the validation controls:
- CompareValidator
- RangeValidator
- RegularExpressionValidator
- RequiredFieldValidator
- ValidationSummary
ASP.NET CompareValidator Control:
It is used for comparing the value of an input control against the value of another input control.
// comparevalidator_demo.aspx <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="comparevalidator_demo.aspx.cs" Inherits="asp.netexample.comparevalidator_demo" %> <!DOCTYPE html> <html xmlns="https://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> <style type="text/css"> .auto-style1 { width: 100%; } .auto-style2 { height: 26px; } .auto-style3 { height: 26px; width: 93px; } .auto-style4 { width: 93px; } </style> </head> <body> <form id="formValidator" runat="server"> <table class="auto-style1"> <tr> <td class="auto-style3"> Small number:</td> <td class="auto-style2"> <asp:TextBox ID="txtfirstvalue" runat="server" required="true"></asp:TextBox> </td> </tr> <tr> <td class="auto-style4"> Big number:</td> <td> <asp:TextBox ID="txtsecondvalue" runat="server"></asp:TextBox> </td> </tr> <tr> <td class="auto-style4"></td> <td> <asp:Button ID="btnSaveButton" runat="server" Text="save"/> </td> </tr> </table> < asp:CompareValidator ID="CompareValidator" runat="server" ControlToCompare="txtsecondvalue" ControlToValidate="txtfirstvalue" Display="Dynamic" ErrorMessage="The first number should be smaller" ForeColor="Red" Operator="LessThan" Type="Integer"></asp:CompareValidator> </form> </body> </html>
Output:
ASP.NET RangeValidator Control:
This ASP.NET validators will evaluate the value of an input control for checking that the value lies between specified ranges.
It also allows us to check whether the user input is between a specified upper boundary and lower boundary. This range can be either numbers, alphabetic characters or dates.
The ControlToValidateproperty is used for specifying the control to validate. The properties MinimumValue and MaximumValue are used to set minimum and maximum boundaries for the control.
// RangeValidator_demo.aspx <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="RangeValidator_demo.aspx.cs" Inherits="asp.netexample.RangeValidator_demo" %> <!DOCTYPE html> <html xmlns="https://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> <style type="text/css"> .auto-style1 { height: 82px; } .auto-style2 { width: 100%; } .auto-style3 { width: 89px; } .auto-style4 { margin-left: 80px; } </style> </head> <body> <form id="formRangeValidator" runat="server"> <div class="auto-style1"> <p class="auto-style4"> Enter value between 100 and 200<br/> </p> <table class="auto-style2"> <tr> <td class="auto-style3"> <asp:Label ID="lblEnterValue" runat="server" Text="Enter a value"></asp:Label> </td> <td> <asp:TextBox ID="txtuserInput" runat="server"></asp:TextBox> <asp:RangeValidator ID="RangeValidator" runat="server" ControlToValidate="txtuserInput" ErrorMessage="Enter value in specified range" ForeColor="Red" MaximumValue="199" MinimumValue="101" SetFocusOnError="True"Type=" Integer"></asp:RangeValidator> </td> </tr> <tr> <td class="auto-style3"> </td> <td> <br/> <asp:Button ID="btnSaveButton" runat="server" Text="Save"/> </td> </tr> </table> <br/> <br/> </div> </form> </body> </html>
Output:
It will throw an error message when the input is not in range.
ASP.NET RegularExpressionValidator Control:
This ASP.NET validators is used to validate an input control’s value against the pattern defined by a regular expression.
It also allows us to check and validate predictable sequences of characters like e-mail addresses, telephone numbers, etc.
The property ValidationExpression is used to specify the regular expression; this expression is used to validate input control.
// RegularExpression_Demo.aspx <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="RegularExpression_Demo.aspx.cs" Inherits="asp.netexample.RegularExpression_Demo" %> <!DOCTYPE html> <html xmlns="https://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="formRegExValidator" runat="server"> <div> <table class="auto-style1"> <tr> <td class="auto-style2">Email ID</td> <td> <asp:TextBox ID="txtemail" runat="server"></asp:TextBox> <asp:RegularExpressionValidator ID="RegularExpressionValidator" runat="server"ControlToValidate="txtemail" ErrorMessage="Please enter valid email" ForeColor="Red" ValidationExpression="\w+([-+.'_]\w+)*@\w+([-._]\w+)*\.\w+([-._]\w+)*"> </asp:RegularExpressionValidator> </td> </tr> <tr> <td class="auto-style2"></td> <td> <br/> <asp:Button ID="btnSaveButton" runat="server" Text="Save"/> </td> </tr> </table> </div> </form> </body> </html>
Output:
It produces the following output when viewing in the browser.
It will validate the e-mail format as we specified in the regular expression. If validation fails, it will throw an error message.
ASP.NET RequiredFieldValidator Control:
RequiredFieldValidator is used to make an input control required which will throw an error if the user leaves input control empty.
It is also used to mandate form control required and restrict the user from providing data.
It can remove extra spaces from the beginning and end of the input value before validation is performed.
The ControlToValidateproperty must be set with the ID of the control to validate.
// RequiredFieldValidator_demo.aspx <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="RequiredFieldValidator_demo.aspx.cs" Inherits="asp.netexample.RequiredFieldValidator_demo" %> <!DOCTYPE html> <html xmlns="https://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> <style type="text/css"> .auto-style1 { width: 100%; } .auto-style2 { width: 165px; } </style> </head> <body> <form id="formFieldValidator" runat="server"> <div> </div> <table class="auto-style1"> <tr> <td class="auto-style2">User Name</td> <td> <asp:TextBox ID="txtname" runat="server"></asp:TextBox> <asp:RequiredFieldValidatorIDasp:RequiredFieldValidatorID="user" runat="server" ControlToValidate="txtname" ErrorMessage="Please enter a user name." ForeColor="Red"></asp:RequiredFieldValidator> </td> </tr> <tr> <td class="auto-style2">Password</td> <td> <asp:TextBox ID="txtpwd" runat="server"></asp:TextBox> <asp:RequiredFieldValidator ID="pass" runat="server" ControlToValidate="txtpwd" ErrorMessage="Please enter a password" ForeColor="Red"></asp:RequiredFieldValidator> </td> </tr> <tr> <td class="auto-style2"> </td> <td> <br/> <asp:Button ID="btnLoginButton" runat="server" Text="login"/> </td> </tr> </table> </form> </body> </html>
Output:
It will produce the following output when view in the browser.
It will throw an error message when the user login with empty controls.
ASP.NET ValidationSummary Control:
This validator is used to display a list of all validation errors in the web form.
It also allows us to summarize the error messages at a single location.
We can set the DisplayMode property to display error messages as a list, bullet list, or single paragraph.
// ValidationSummery_Demo.aspx <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="ValidationSummery_Demo.aspx.cs" Inherits="asp.netexample.ValidationSummery_Demo" %> <!DOCTYPE html> <html xmlns="https://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="formSummaryValidation" runat="server"> <div> </div> <table class="auto-style1"> <tr> <td class="auto-style2">User Name</td> <td> <asp:TextBox ID="txtname" runat="server"></asp:TextBox> <asp:RequiredFieldValidator ID="user" runat="server" ControlToValidate="txtname" ErrorMessage="Please enter a user name." ForeColor="Red">*</asp:RequiredFieldValidator> </td> </tr> <tr> <td class="auto-style2">Password</td> <td> <asp:TextBox ID="txtpwd" runat="server"></asp:TextBox> <asp:RequiredFieldValidator ID="pass" runat="server" ControlToValidate="txtpwd" ErrorMessage="Please enter a password" ForeColor="Red">*</asp:RequiredFieldValidator> </td> </tr> <tr> <td class="auto-style2"> <br/> <asp:Button ID="btnLoginButton" runat="server"Text="login"/> </td> <td> <asp:ValidationSummary ID="ValidationSummary" runat="server" ForeColor="Red"/> <br/> </td> </tr> </table> </form> </body> </html>
Output:
It produces the following output when viewing in the browser.
It throws an error summary when the user login without credentials.