JAVA Tutorials
Reflection Framework in Java
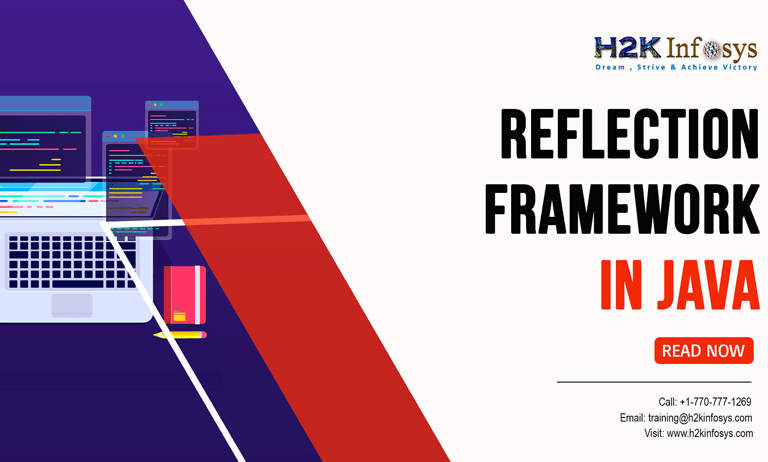
Reflection concept in Java is used to inspect and change the behavior of classes, methods, interfaces, and constructors during compile time.
- All the classes required for Reflection are available under package java.lang.reflect
- This API is mainly used in IDE like Eclipse, Netbeans, etc., debugging tools like JUnit, etc.
- It gives the information about the class to which the object belongs and also the methods that are accessible by the object.
Advantages:
- Reflection is used in Debugging and Testing tools to determine the private members of a class.
- External and user-defined classes can also be used with the help of instance creations using their full names.
Drawbacks:
- Reflective code changes the behavior with the updation in a platform and thereby breaking the abstraction.
- It slows the performance and hence should be avoided when there is recursion in the code.
- It requires runtime permissions, which may not be available if the system is working under a security manager, which may also cause the failure of the system.
- With the help of Reflection, we can access the private members of the class, which can cause security issues.
- Reflection code is less flexible and hard to maintain.
Reflection is used in
- Class: getClass() method is used to retrieve the name of the class to which the object belongs.
- Method: getMethod() returns all the public methods of the class to which the object belongs.
- Constructor: getConstructor() returns all the public constructors of the class to which the object belongs.
How to create the object of a class
There are three methods to create the objects of a class:
1. Using forName() : It takes string as an argument.
Eg. Class C = Class.forName(“Cat”);
2. Using getClass() : It uses the object of a class to create object of its own class.
Eg. Animal A = new Animal();
Class C = A.getClass();
3. Using .class: Objects can also be instantiated using .class extension.
Eg. Class C = Animal.class;
GetInterfaces() : It is used to collect the information about the constructors of a class. Return type is array.
interface Animal { public void display(); } interface Mammal { public void makeSound(); } class Dog implements Animal, Mammal { public void display() { System.out.println("I am a dog."); } public void makeSound() { System.out.println("Bark bark"); } } class ReflectionDemo { public static void main(String[] args) { try { // create an object of Dog class Dog d1 = new Dog(); Class obj = d1.getClass(); Class[] objInterface = obj.getInterfaces(); for(Class c : objInterface) { // print the name of interfaces System.out.println("Interface Name: " + c.getName()); } } catch(Exception e) { e.printStackTrace(); } } }
GetSuperclass(): This method gives the information about all the constructors of a class.
interface Animal { public void display(); } public class Dog implements Animal { public void display() { System.out.println("I am a dog."); } } class ReflectionDemo { public static void main(String[] args) { try { Dog d1 = new Dog(); Class obj = d1.getClass(); int modifier = obj.getModifiers(); System.out.println("Modifier: " + Modifier.toString(modifier)); Class superClass = obj.getSuperclass(); System.out.println("Superclass: " + superClass.getName()); } catch(Exception e) { e.printStackTrace(); } } }
Methods used in accessing fields:
- getFields(): It returns all the public fields of the class along with its super class.
- getModifiers():It returns the modifier of the class in the form of integer.
- Get(ClassObject): It returns the value of the field.
- setAccessible(Boolean): It makes the private fields of a class accessible.
class Dog { public String type; } class ReflectionDemo { public static void main(String[] args) { try{ Dog d1 = new Dog(); Class obj = d1.getClass(); Field field1 = obj.getField("type"); field1.set(d1, "labrador"); String typeValue = (String)field1.get(d1); System.out.println("type: " + typeValue int mod1 = field1.getModifiers(); String modifier1 = Modifier.toString(mod1); System.out.println("Modifier: " + modifier1); System.out.println(" "); } catch(Exception e) { e.printStackTrace(); } } }
Methods used in accessing methods of a class:
- getMethods():It returns all the public methods of the class along with its super class.
- getModifiers():It returns the modifier of the method of a class in the form of integer.
- getName():It will return the name of the method.
- getReturnType(): It returns the type of a method.
class Dog { public void display() { System.out.println("I am a dog."); } protected void eat() { System.out.println("I eat dog food."); } private void makeSound() { System.out.println("Bark Bark"); } } class ReflectionDemo { public static void main(String[] args) { try { Dog d1 = new Dog(); Class obj = d1.getClass(); Method[] methods = obj.getDeclaredMethods(); for(Method m : methods) { System.out.println("Method Name: " + m.getName()); int modifier = m.getModifiers(); System.out.println("Modifier: " + Modifier.toString(modifier)); System.out.println("Return Types: " + m.getReturnType()); System.out.println(" "); } } catch(Exception e) { e.printStackTrace(); } } }
Methods used in accessing constructors of a class:
- getConstructors():It returns all the public constructors of the class along with its super class.
- getModifiers():It returns the modifier of the constructor of a class in the form of integer.
- getName():It returns the name of the constructor.
- getParameterCount(): It returns the number of parameters used in a constructor.
class Dog { public Dog() { } public Dog(int age) { } private Dog(String sound, String type) { } } class ReflectionDemo { public static void main(String[] args) { try { Dog d1 = new Dog(); Class obj = d1.getClass(); Constructor[] constructors = obj.getDeclaredConstructors(); for(Constructor c : constructors) { System.out.println("Constructor Name: " + c.getName()); int modifier = c.getModifiers(); System.out.println("Modifier: " + Modifier.toString(modifier)); System.out.println("Parameters: " + c.getParameterCount()); } } catch(Exception e) { e.printStackTrace(); } }
Facebook Comments