MVC Project
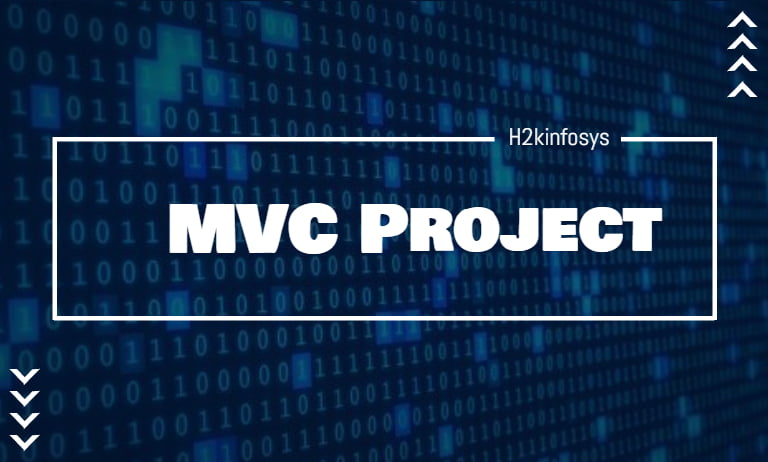
MVC is a software architectural pattern that is used for developing web applications. It categorizes a given application into the three interconnected parts.
a) Model -It means the data. The model did not depend on the Controller or the View. We can say, it is a virtual representation of data used to perform any operation in the code.
b) View – View is responsible for displaying the UI to the user.
c) Controller – It mainly handles the incoming request coming from the browser and process these requests to implement the View.
The Model View Controller(MVC) design pattern decouples these major components allowing us for code reusability and parallel development. In this pattern, the Controller receives all incoming requests from the browser and then works with the model to identify the data used to create a view. The View then uses the data and generates a final presentable UI.
The MVC Architectural framework is generally a term for a software architecture for implementing the user interfaces. You can separate the software’s source code into three different layers:
- Model
- View
- Controller
Let us have an example to create Login Form using JSP + Servlet + JDBC + MySQL Example
Now we will create an Employee Login Form, and we will validate employee username and password with the database.
1. Create table in MySQL
CREATE TABLE `login` ( `user_name` varchar(40) NOT NULL, `pass_word` varchar(40) DEFAULT NULL, PRIMARY KEY (`user_name`) )
2. Now insert SQL statement:
INSERT INTO `mysql_database`.`login` (`user_name`, `pass_word`) VALUES ("Ram", "Ramesh");
3. LoginBean.java
import java.io.Serializable; public class LoginBean implements Serializable { private static final long serialVersionUID = 1 L; private String user_name; private String pass_word; public String getUsername() { return user_name; } public void setUsername(String username) { this.username = user_name; } public String getPassword() { return pass_word; } public void setPassword(String password) { this.password = pass_word; } }
4. LoginDao.java
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import test.login.bean.LoginBean; public class LoginDao { public booleanvalidate(LoginBeanloginBean) throws ClassNotFoundException { boolean status = false; Class.forName("com.mysql.jdbc.Driver"); try (Connection connection = DriverManager .getConnection("jdbc:mysql://localhost:3306/mysql_database?useSSL=false", "root", "root"); // Step 2:Create a statement using the connection object PreparedStatementpreparedStatement = connection .prepareStatement("select * from login where user_name = ? and pass_word = ? ")) { preparedStatement.setString(1, loginBean.getUsername()); preparedStatement.setString(2, loginBean.getPassword()); System.out.println(preparedStatement); ResultSetrs = preparedStatement.executeQuery(); status = rs.next(); } catch (SQLException e) { // process sql exception printSQLException(e); } return status; } private void printSQLException(SQLException ex) { for (Throwable e: ex) { if (e instanceofSQLException) { e.printStackTrace(System.err); System.err.println("SQLState: " + ((SQLException) e).getSQLState()); System.err.println("Error Code: " + ((SQLException) e).getErrorCode()); System.err.println("Message: " + e.getMessage()); Throwable t = ex.getCause(); while (t != null) { System.out.println("Cause: " + t); t = t.getCause(); } } } } }
5. Create a LoginServlet.java
Let us create LoginServlet to process HTTP request parameters and redirect to the appropriate JSP page based on the employee login status. If login successfully validated with the database, then redirect to loginsuccess.jsp page otherwise redirect to login.jsp page.
import java.io.IOException; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.http.HttpSession; import test.login.bean.LoginBean; import test.login.database.LoginDao; @WebServlet("/login") public class LoginServlet extends HttpServlet { private static final long serialVersionUID = 1 L; private LoginDaologinDao; public void init() { loginDao = new LoginDao(); } protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String username = request.getParameter("user_name"); String password = request.getParameter("pass_word"); LoginBeanloginBean = new LoginBean(); loginBean.setUsername(user_name); loginBean.setPassword(pass_word); try { if (loginDao.validate(loginBean)) { //HttpSession session = request.getSession(); // session.setAttribute("username",user_name); response.sendRedirect("loginsuccess.jsp"); } else { HttpSession session = request.getSession(); //session.setAttribute("user", user_name); //response.sendRedirect("login.jsp"); } } catch (ClassNotFoundException e) { e.printStackTrace(); } } }
8. Create a login.jsp
Let us design login HTML form with following fields:
- username
- password
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <!DOCTYPE html> <html> <head> <meta charset="ISO-8859-1"> <title>Insert title here</title> </head> <body> <div align="center"> <h1>Employee Login Form</h1> <form action="<%=request.getContextPath()%>/login" method="post"> <table style="with: 100%"> <tr> <td>UserName</td> <td><input type="text" name="username" /></td> </tr> <tr> <td>Password</td> <td><input type="password" name="password" /></td> </tr> </table> <input type="submit" value="Submit" /> </form> </div> </body> </html>
9. Create a loginsuccess.jsp
After an employee successfully login then this page shows a successful message on screen:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <%@page import="test.login.database.*"%> <!DOCTYPE html> <html> <head> <meta charset="ISO-8859-1"> <title>Insert title here</title> </head> <body> <div align="center"> <h1>You have logined successfully</h1> </div> </body> </html>
First OUTPUT, after entering the details:
EMPLOYEE LOGIN FORM
User Name: Ram
Password: ****
Submit
Second OUTPUT:
You Have Logged in Successfully.
One Comment