How to Print Objects in Python using the Print Function
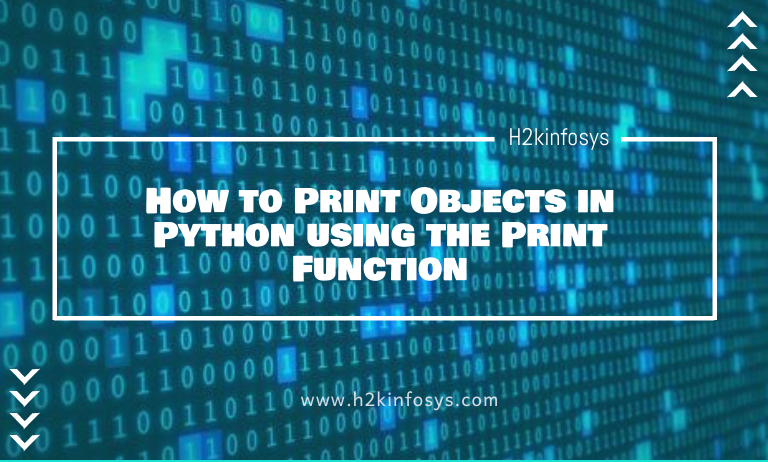
In python, you will always need to display output on your console to check the flow of your code. You can do this using the print objects in python 3.
NB: In python 2, ‘print’ was not a function but a statement. This is one of the major changes from Python 2 to Python 3.
That said, let’s see how to work with the print function in Python 3.
Print Function Syntax
Docstring: print(value, ..., sep=' ', end='\n', file=sys.stdout, flush=False) Prints the values to a stream, or to sys.stdout by default. Optional keyword arguments: file: a file-like object (stream); defaults to the current sys.stdout. sep: string inserted between values, default a space. end: string appended after the last value, default a newline. flush: whether to forcibly flush the stream.
As earlier discussed, the print function is used to display object values on your console. From the docstring, you’d find that you can print more than one object at a time. Each object is separated by a comma. Let’s see an example
#print all 4 objects at once print('Hello', 1, True, 23.4)
Output:
Hello 1 True 23.4
You’d realize that each object was separated by whitespace. You can change this using the sep argument.
Using the sep argument in the Print Function Python
With the sep argument, you can change how each object is separated. If we want each object to be separated by a forward slash, we set sep=’/ ’. Let’s see a coding example.
#print all 4 objects separated with / print('Hello', 1, True, 23.4, sep='/ ')
Output:
Hello/ 1/ True/ 23.4
As seen the objects are now separated by a backward slash /. Note that you can change the argument to whatever character you wish. However, the argument has to be a string.
Using the end argument in the Print Function Python
When you call the print function twice or more consecutively, the python interpreter displays the values of the print function on a new line. See the example below.
#print each object individually print('Hello') print(1) print(True) print(23.4)
Output:
Hello
1
True
23.4
Notice that each object was printed on a new line. You can change this using the end argument. If we set end=’, ‘, it means a comma will be added after the values of the print function are printed.
print each object individually
print('Hello', end=', ')
print(1, end=', ')
print(True, end=', ')
print(23.4)
Output:
Hello
1
True
23.4
Note that you can change the end argument for every print function called.
‘\n’ means new line
‘\t’ means tab i.e 8 whitespaces
‘…’ means ellipses and so on.
Let’s see an example.
print each object individually
print('Hello', end='\n')
print(1, end='\t')
print(True, end=', ')
print(23.4, end='…')
Output:
Hello
1 True, 23.4…
As seen in the result, after ‘Hello’, the next object was printed on a new line. After 1, the next object was printed with 8 whitespaces. After True, a comma was printed. Finally, after 23.4, an ellipse was printed.
These are the two major arguments you should understand when printing objects onto your terminal, the end and sep operator. Now, you know how to use the print function in Python. If you have any questions, feel free to drop them in the comment section and I’d do my best to answer them.
2 Comments