Servlet Configuration and Context
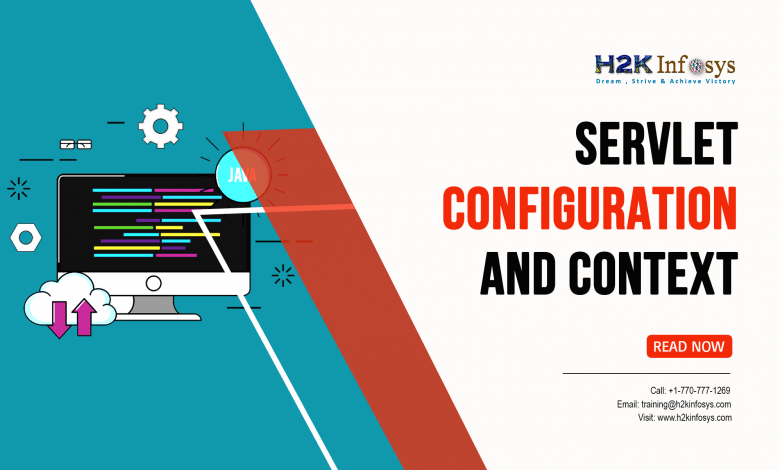
Servlet Configuration
Each Servlet keeps its configuration in a ServletConfig object. This object is created by the web container for each servlet. A ServletConfig object can be used to get configuration information from the web.xml file. It is easier to manage the web application if the servlet configuration information is in one web.xml file. The best advantage of ServletConfig is that you don’t need to edit the servlet file if the information is in this XML file. The ServletConfig is feeling with information by Web container during initialization of servlets.
The ServletConfig is an interface. It has several methods.
Enumeration getInitParameterNames().
They have an Enumeration type. We can get the name of the servlet:
String getServletName().
There is a method that returns the context of the Servlet:
ServletContext getServletContext().
This means that Java servlet configuration contains servlet context.
Let’s look closer at web.xml file. This file is located in the folder WEB-INF.
Under the servlet element, you can define a name for the servlet, a compiled class, or JSP that executes the servlet, definitions for initialization attributes, and the servlet security roles.
Let’s assume that we have our class MyTestServlet that extends HTTPServlet class in the package edu.test.myservlets. In the configuration file web.xml we will declare it like this:
<servlet> <servlet-name>mytestservlet</servlet-name> <servlet-class>edu.test.myservlets.MyTestServlet</servlet-class> </servlet> In the servlet element, we can also initialize parameters. This particular element looks like following: <init-param> <param-name>parametername</param-name> <param-value>parametervalue</param-value> </init-param> The init parameters can only be accessed by that servlet that contains it. Let's insert this element into our servlet element: <servlet> <servlet-name>mytestservlet</servlet-name> <servlet-class>edu.test.myservlets.MyTestServlet</servlet-class> <init-param> <param-name>test</param-name> <param-value>test</param-value> </init-param> </servlet>
Let’s look how you can read the init parameters from your servlet. It happens in the servlets init() method:
public void init(ServletConfig servletConfig) throws ServletException{ this.testParam = servletConfig.getInitParameter("test"); }
Eventually, the servlet element has also a subelement called load-on-startup. This element you should use to control when the servlet container needs to load the servlet. Without specifying a load-on-startup element, the servlet container will load your servlet when the first request arrives for it.
You can tell to load the servlet as soon as the servlet container starts.
The number inside the load-on-startup element tells in what sequence the servlets should be loaded. The lower numbers are loaded first. In case your value is negative, or unspecified, the servlet container will load the servlet at any time.
The second valuable XML element in the web.xml for our Servlet is its mapping. Servlet mapping describes the way you can access a servlet. It shows the URL used to invoke the servlet. In the following example, you can find how to use servlet-mapping in your application.
<servlet-mapping> <servlet-name>mytestservlet</servlet-name> <url-pattern>/test/myservlet</url-pattern> </servlet-mapping>
We can use the wildcard for a URL pattern. In this case, our servlet will be invoked for more requests. Let’s look at some cases:
<servlet-mapping> <servlet-name>fruitsservlet</servlet-name> <url-pattern>/fruits/*</url-pattern> </servlet-mapping>
The FruitsServlet will be called in case you use URLs:
http://host:port/mywebapp/fruits/summer.html
http://host:port/mywebapp/fruits/document.pdf
We can start our URL from wildcard. For example:
<servlet-mapping> <servlet-name>documentservlet</servlet-name> <url-pattern>*.doc</url-pattern> </servlet-mapping>
In this case, our ServletDocument will handle all requests that came from URLs like:
http://host:port/mywebapp/document.doc
http://host:port/mywebapp/important/document.doc
But, if we have a servlet mapped for URL mywebapp/important/*, then that servlet will be called.
In web.xml you can also set context parameters. Those values can be read from all servlets of your application. Here is an example of configuration a context parameter:
<servlet-mapping> <servlet-name>documentservlet</servlet-name> <url-pattern>*.doc</url-pattern> </servlet-mapping>
In this case, our ServletDocument will handle all requests that came from URLs like:
http://host:port/mywebapp/document.doc
http://host:port/mywebapp/important/document.doc
But, if we have a servlet mapped for URL mywebapp/important/*, then that servlet will be called.
In web.xml you can also set context parameters. Those values can be read from all servlets of your application. Here is an example of configuration a context parameter:
<context-param> <param-name>test</param-name> <param-value>Test context parameter</param-value> </context-param>
To get this value from your servlet code you need to write:
String myContextParam=request.getSession().getServletContext().getInitParameter(“test”);
Let’s write a servlet that will return servlet name and all its init parameters. Here is web.xml:
<web-app> <servlet> <servlet-name>myservlet</servlet-name> <servlet-class>edu.servlets.examples.ServletExample</servlet-class> <init-param> <param-name>myparameter</param-name> <param-value>Here is our init parameter</param-value> </init-param> </servlet> <servlet-mapping> <servlet-name>myservlet</servlet-name> <url-pattern>/testinitparams/myservlet</url-pattern> </servlet-mapping> </web-app>
Here is our servlet:
package edu.servlets.examples; import java.io.*; import java.util.Enumeration; import javax.servlet.*; import javax.servlet.http.*; // we are extending HttpServlet class public class ServletExample extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Set response content type response.setContentType("text/html"); PrintWriter out = response.getWriter(); ServletConfig configuration = getServletConfig(); // we are building a response Enumeration<String> paramNames = configuration.getInitParameterNames(); out.println("The servlet name is "+ configuration.getServletName()); out.println("Here are init parameters:"); String param = ""; while (paramNames.hasMoreElements()) { param = paramNames.nextElement(); out.print("<br>Name: " + param); out.println(" value: " + configuration.getInitParameter(param)); } out.close(); } }
If we navigate to the URL http://<host>:<port>/<mywebapp>/testinitparams/myservlet, we will get
The servlet name is myservlet
Here are init parameters:
Name: myparameter value: Here is our init parameter
ServletContext
The interface ServletContext is in the package javax.servlet.
It defines a set of methods used by a servlet to communicate with its servlet container, such as to get the MIME type of a file, to write into a log file, to dispatch requests.
Usually, there is one context is per Web application inside one Java Virtual Machine. You can call is “distributed” in its deployment descriptor. In this case, will be one context instance for each virtual machine. But, the context cannot be used as a location to share global information.
As we mentioned before, the ServletContext object is contained inside the ServletConfig object. The servlet container provides it to a servlet when the servlet is initialized.
Let’s create an example to show how name-value pairs for ServletContext work. Also we will add attribute, get that attribute and remove it.
Here is our web.xml where you can see context:
<web-app> <servlet> <servlet-name>myservlet2</servlet-name> <servlet-class>edu.servlets.examples.ServletExample2</servlet-class> </servlet> <servlet-mapping> <servlet-name>myservlet2</servlet-name> <url-pattern>/testcontextparams/myservlet</url-pattern> </servlet-mapping> <context-param> <param-name>param1</param-name> <param-value>Context parameter 1</param-value> </context-param> <context-param> <param-name>param2</param-name> <param-value>Context parameter 2</param-value> </context-param> <context-param> <param-name>param3</param-name> <param-value>Context parameter 3</param-value> </context-param> </web-app>
Here is our servlet:
package edu.servlets.examples; import java.io.*; import java.util.Enumeration; import javax.servlet.*; import javax.servlet.http.*; // we are extending HttpServlet class public class ServletExample2 extends HttpServlet { public void init() throws ServletException { } public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Set response content type response.setContentType("text/html"); PrintWriter out = response.getWriter(); ServletConfig configuration = getServletConfig(); ServletContext context = configuration.getServletContext(); // we are building a response Enumeration<String> paramNames = context.getInitParameterNames(); out.println("Here are context parameters:"); String param = ""; while (paramNames.hasMoreElements()) { param = paramNames.nextElement(); out.print("Name: " + param); out.println(" value: " + configuration.getInitParameter(param)); } //let's set and attributes context.setAttribute("attribute", "Our first attribute"); out.print("Here is our attribute: "); out.println(context.getAttribute("attribute")); //we also can remove that attribute context.removeAttribute("attribute"); out.close(); } }
If we navigate to the URL http://<host>:<port>/<mywebapp>/testcontextparams/myservlet, we will get:
Here are context parameters:
Name:param1 value: Context parameter 1
Name:param2 value: Context parameter 2
Name:param3 value: Context parameter 3
Here is our attribute: Our first attribute
The ServletContext object contains meta-information about your application. It has methods dedicated to getting initialization context parameters, setting them, also setting, getting and removing attributes. There is also a method to return the name and version of the servlet container on which the servlet is running. We can get the context path of the web application.
We can get a SessionCookieConfig object form a servlet context through which various properties of the session tracking cookies created.
There is a possibility to set the session tracking modes(COOKIE, SSL, URL) that are to become effective for this ServletContext. You can set several types of them at once.
With a ServletContext object, we can write a specified explanatory message with or without stack trace for a given Throwable exception to a servlet log file, usually an event log.
There is a possibility to manage a servlet filter with the use of ServletContext object. A filter object performs filtering tasks on the request to a resource, on the response from a resource. It can also filter both. A resource can be a servlet or static content.
Filter doFilter method performs filtering. The filter obtains its initialization parameters from the FilterConfig object. The FilterConfig object reference to the ServletContext which it can use.
We are configuring filters in the deployment descriptor of our web application.
There can be various types of filters such as Authentication Filters, Data compression Filters, Logging and Auditing Filters, Image conversion Filters, Encryption Filters, Tokenizing Filters, Filters that trigger resource access events, etc.
Summary
Let’s summarize the difference between the ServletConfig and the Servlet Context. First of all, the ServletConfig object represents a single servlet, it is like a local parameter associated with the particular servlet. On the contrary, the ServletContext is like a global parameter associated with the whole application and is shareable by all servlet object, it represents the whole application running on a particular JVM and is common for all the servlets.
If you define name-value pairs for a ServletConfig (inside the servlet section of the web.xml file) it has a servlet wide scope. The ServletContext has an application-wide scope and is defined outside of the servlet tag. All the name-value pairs defined our of the servlet element can be got by ServletContext.
We can get the ServletConfig object by the method getServletConfig(), getServletContext() method is used to get the ServletContext object.
There is only one ServletContext object available and accessible by all servlets, but each Servlet comes with a separate ServletConfig object.
4 Comments